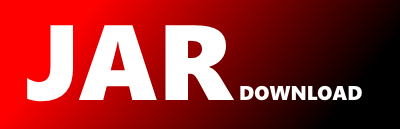
package.components.Progress.progress.scss Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of patternfly Show documentation
Show all versions of patternfly Show documentation
Assets, source, tooling, and content for PatternFly 4
The newest version!
@use '../../sass-utilities' as *;
@include pf-root($progress) {
// Component variables
--#{$progress}--GridGap: var(--pf-t--global--spacer--md);
// Element variables
--#{$progress}__bar--Height: var(--pf-t--global--spacer--md);
--#{$progress}__bar--BackgroundColor: var(--pf-t--global--color--nonstatus--gray--default); // the bar always needs white under it so that the semi-transparent color shows correctly
--#{$progress}__measure--m-static-width--MinWidth: 4.5ch; // 4.5 because the % character is wider than a 0
--#{$progress}__status--Gap: var(--pf-t--global--spacer--sm);
--#{$progress}__status-icon--Color: var(--pf-t--global--icon--color--regular);
--#{$progress}__indicator--Height: var(--#{$progress}__bar--Height);
--#{$progress}__indicator--BackgroundColor: var(--pf-t--global--color--brand--default);
--#{$progress}__helper-text--MarginBlockStart: calc(var(--pf-t--global--spacer--sm) - var(--#{$progress}--GridGap));
// Modifier variables
--#{$progress}--m-success__indicator--BackgroundColor: var(--pf-t--global--color--status--success--default);
--#{$progress}--m-warning__indicator--BackgroundColor: var(--pf-t--global--color--status--warning--default);
--#{$progress}--m-danger__indicator--BackgroundColor: var(--pf-t--global--color--status--danger--default);
--#{$progress}--m-success__status-icon--Color: var(--pf-t--global--icon--color--status--success--default);
--#{$progress}--m-warning__status-icon--Color: var(--pf-t--global--icon--color--status--warning--default);
--#{$progress}--m-danger__status-icon--Color: var(--pf-t--global--icon--color--status--danger--default);
--#{$progress}--m-inside__indicator--MinWidth: var(--pf-t--global--spacer--xl);
--#{$progress}--m-inside__measure--Color: var(--pf-t--global--text--color--on-brand--default);
--#{$progress}--m-success--m-inside__measure--Color: var(--pf-t--global--text--color--status--on-success--default);
--#{$progress}--m-warning--m-inside__measure--Color: var(--pf-t--global--text--color--status--on-warning--default);
--#{$progress}--m-danger--m-inside__measure--Color: var(--pf-t--global--text--color--status--on-danger--default);
--#{$progress}--m-inside__measure--FontSize: var(--pf-t--global--font--size--sm);
--#{$progress}--m-outside__measure--FontSize: var(--pf-t--global--font--size--sm);
--#{$progress}--m-sm__bar--Height: var(--pf-t--global--spacer--sm);
--#{$progress}--m-sm__measure--FontSize: var(--pf-t--global--font--size--body--sm);
--#{$progress}--m-lg__bar--Height: var(--pf-t--global--spacer--lg);
}
.#{$progress} {
display: grid;
grid-template-rows: 1fr auto;
grid-template-columns: auto auto;
grid-gap: var(--#{$progress}--GridGap);
align-items: end;
// Modifiers
// size modifiers
&.pf-m-sm {
--#{$progress}__bar--Height: var(--#{$progress}--m-sm__bar--Height);
.#{$progress}__measure {
font-size: var(--#{$progress}--m-sm__measure--FontSize);
}
}
&.pf-m-lg {
--#{$progress}__bar--Height: var(--#{$progress}--m-lg__bar--Height);
}
// an inside-style progress component shows the measure inside the indicator
&.pf-m-inside {
.#{$progress}__indicator {
display: flex;
align-items: center;
justify-content: center;
min-width: var(--#{$progress}--m-inside__indicator--MinWidth);
}
.#{$progress}__measure {
font-size: var(--#{$progress}--m-inside__measure--FontSize);
color: var(--#{$progress}--m-inside__measure--Color);
text-align: center;
}
}
// an outside-style progress component shows the measure and status icon to the right of the bar
&.pf-m-outside {
.#{$progress}__description {
grid-column: 1 / 3;
}
.#{$progress}__status {
grid-row: 2 / 3;
grid-column: 2 / 3;
align-self: center;
}
.#{$progress}__measure {
display: inline-block;
font-size: var(--#{$progress}--m-outside__measure--FontSize);
&.pf-m-static-width {
min-width: var(--#{$progress}__measure--m-static-width--MinWidth);
text-align: start;
}
}
.#{$progress}__bar,
.#{$progress}__indicator {
grid-column: 1 / 2;
}
}
// No Description. If a measure or status is needed, use with inside or outside measure modifier.
&.pf-m-singleline {
grid-template-rows: 1fr;
.#{$progress}__description {
display: none;
}
.#{$progress}__bar {
grid-row: 1 / 2;
grid-column: 1 / 2;
}
.#{$progress}__status {
grid-row: 1 / 2;
grid-column: 2 / 3;
}
}
&.pf-m-outside,
&.pf-m-singleline {
grid-template-columns: 1fr fit-content(50%);
}
// status modifiers
&.pf-m-success {
--#{$progress}__indicator--BackgroundColor: var(--#{$progress}--m-success__indicator--BackgroundColor);
--#{$progress}__status-icon--Color: var(--#{$progress}--m-success__status-icon--Color);
--#{$progress}--m-inside__measure--Color: var(--#{$progress}--m-success--m-inside__measure--Color);
}
&.pf-m-warning {
--#{$progress}__indicator--BackgroundColor: var(--#{$progress}--m-warning__indicator--BackgroundColor);
--#{$progress}__status-icon--Color: var(--#{$progress}--m-warning__status-icon--Color);
--#{$progress}--m-inside__measure--Color: var(--#{$progress}--m-warning--m-inside__measure--Color);
}
&.pf-m-danger {
--#{$progress}__indicator--BackgroundColor: var(--#{$progress}--m-danger__indicator--BackgroundColor);
--#{$progress}__status-icon--Color: var(--#{$progress}--m-danger__status-icon--Color);
--#{$progress}--m-inside__measure--Color: var(--#{$progress}--m-danger--m-inside__measure--Color);
}
}
// Elements
// the progress__description is displayed above the progress bar on the left
.#{$progress}__description {
grid-column: 1 / 2;
word-break: break-word;
&.pf-m-truncate {
@include pf-v6-text-overflow;
}
}
// the progress__status is displayed in the upper right
.#{$progress}__status {
display: flex;
grid-row: 1 / 2;
grid-column: 2 / 3;
gap: var(--#{$progress}__status--Gap);
align-items: flex-start;
justify-content: flex-end;
text-align: end;
word-break: break-word;
}
// the progress__status-icon is an icon displayed always in the upper right
.#{$progress}__status-icon {
color: var(--#{$progress}__status-icon--Color);
}
.#{$progress}__bar::before,
.#{$progress}__indicator {
// disable linter as the indicator element should always grow ltr
// stylelint-disable liberty/use-logical-spec
inset-block-start: 0;
inset-inline-start: 0;
// stylelint-enable
}
// The progress__bar is a white underlay with a semi-transparent color on top, which matches the progress__indicator color
.#{$progress}__bar {
position: relative;
grid-row: 2 / 3;
grid-column: 1 / 3;
align-self: center;
height: var(--#{$progress}__bar--Height);
background-color: var(--#{$progress}__bar--BackgroundColor);
}
// the progress__indicator is the part that advances (widens) as progress is made
// It is assumed that the width of the progress__indicator is set inline or via Javascript
.#{$progress}__indicator {
position: absolute; // position absolute to bring above the semi-transparent bar overlay
height: var(--#{$progress}__indicator--Height);
background-color: var(--#{$progress}__indicator--BackgroundColor);
}
.#{$progress}__helper-text {
grid-row: 3 / 4;
grid-column: 1 / 3;
margin-block-start: var(--#{$progress}__helper-text--MarginBlockStart);
}
.#{$progress}__measure {
font-variant-numeric: tabular-nums;
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy