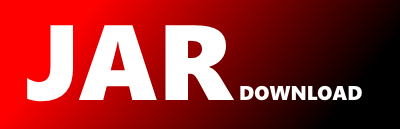
package.sass-utilities.functions.scss Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of patternfly Show documentation
Show all versions of patternfly Show documentation
Assets, source, tooling, and content for PatternFly 4
The newest version!
// PatternFly functions
// --------------------------------------------------
@use '../sass-utilities/scss-variables' as *;
@use '../sass-utilities/init' as *;
@use 'sass:math';
// Transform px to rems
@function pf-strip-unit($num) {
@if type-of($num) == 'number' and not unitless($num) {
@return math.div($num, ($num * 0 + 1));
}
@return $num;
}
@function pf-size-prem($pxval, $base: $pf-v6-global--space-size-root) {
@return math.div(pf-strip-unit($pxval), $base) * 1rem;
}
@function pf-font-prem($pxval, $base: $pf-v6-global--font-size-root) {
@return math.div(pf-strip-unit($pxval), $base) * 1rem;
}
// Return (width) breakpoint value if it exists
@function pf-breakpoint-value($breakpoint, $breakpoint-map: $pf-v6-global--breakpoint-name-map) {
$breakpoint-value: if(map-has-key($breakpoint-map, #{$breakpoint}), map-get($breakpoint-map, #{$breakpoint}), false);
@return #{$breakpoint-value};
}
// Return height breakpoint value if it exists
@function pf-height-breakpoint-value($height-breakpoint, $height-breakpoint-map: $pf-v6-global--height-breakpoint-name-map) {
$height-breakpoint-value: if(map-has-key($height-breakpoint-map, #{$height-breakpoint}), map-get($height-breakpoint-map, #{$height-breakpoint}), false);
@return #{$height-breakpoint-value};
}
// Build breakpoint map
// Based on $pf-v6-global--breakpoint-name-map
@function build-breakpoint-map($map-items...) {
$map: ();
@if length($map-items) == 0 {
$map: ("base": null);
$map: map-merge($map, $pf-v6-global--breakpoint-name-map);
} @else {
@each $breakpoint in $map-items {
@if not map-has-key($pf-v6-global--breakpoint-name-map, $breakpoint) and $breakpoint != "base" {
$map: map-merge($map, ("invalid breakpoint #{$breakpoint}": null));
} @else if $breakpoint == "base" {
$map: map-merge($map, ($breakpoint: null));
} @else {
$map: map-merge($map, ($breakpoint: map-get($pf-v6-global--breakpoint-name-map, #{$breakpoint})));
}
}
}
@return $map;
}
// Build height breakpoint map
// Based on $pf-v6-global--height-breakpoint-name-map
@function build-height-breakpoint-map($map-items...) {
$map: ();
@if length($map-items) == 0 {
$map: ("base": null);
$map: map-merge($map, $pf-v6-global--height-breakpoint-name-map);
} @else {
@each $breakpoint in $map-items {
@if not map-has-key($pf-v6-global--height-breakpoint-name-map, $breakpoint) and $breakpoint != "base" {
$map: map-merge($map, ("invalid breakpoint #{$breakpoint}": null));
} @else if $breakpoint == "base" {
$map: map-merge($map, ($breakpoint: null));
} @else {
$map: map-merge($map, ($breakpoint: map-get($pf-v6-global--height-breakpoint-name-map, #{$breakpoint})));
}
}
}
@return $map;
}
// Build spacer map
// Based on $pf-v6-global--spacer-map
@function build-spacer-map($map-items...) {
$map: ();
@if length($map-items) == 0 {
$map: ("none": 0);
$map: map-merge($map, $pf-v6-global--spacer-map);
$map: map-remove($map, "auto", "0");
}
@each $spacer in $map-items {
@if $spacer == "none" {
$map: map-merge($map, ($spacer: 0));
} @else {
$map: map-merge($map, ($spacer: map-get($pf-v6-global--spacer-map, #{$spacer})));
}
}
@return $map;
}
// Build variable map
// Based on custom map
@function build-variable-map($namespace, $map: ()) {
$new-map: ();
@each $size, $value in $map {
$new-map: map-merge($new-map, (map-get($map, $size): --#{$namespace}--#{$size}));
}
@return $new-map;
}
// Returns a calc() with the inverse of a value used for RTL. Most commonly used as the inverse/negative of a value in a transform function (eg, translate(), rotate(), scale(), etc)
// Shouldn't be used on an existing LTR style as adding a calc() in an existing style could be breaking. Should used within an RTL selector, which is basically an opt-in.
@function pf-v6-calc-inverse($val, $multiplier: var(--#{$pf-global}--inverse--multiplier)) {
@return calc(#{$val} * #{$multiplier});
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy