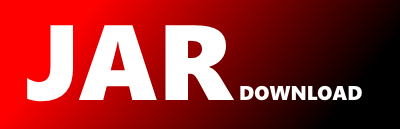
package.src.__tests__.createIcon.test.tsx Maven / Gradle / Ivy
import React from 'react';
import { render, screen } from '@testing-library/react';
import { createIcon } from '../createIcon';
const iconDef = {
name: 'IconName',
width: 10,
height: 20,
svgPath: 'svgPath'
};
const SVGIcon = createIcon(iconDef);
test('sets correct viewBox', () => {
render( );
expect(screen.getByRole('img', { hidden: true })).toHaveAttribute(
'viewBox',
`0 0 ${iconDef.width} ${iconDef.height}`
);
});
test('sets correct svgPath', () => {
render( );
expect(screen.getByRole('img', { hidden: true }).querySelector('path')).toHaveAttribute('d', iconDef.svgPath);
});
test('aria-hidden is true if no title is specified', () => {
render( );
expect(screen.getByRole('img', { hidden: true })).toHaveAttribute('aria-hidden', 'true');
});
test('title is not renderd if a title is not passed', () => {
render( );
expect(screen.queryByRole('img', { hidden: true })?.querySelector('title')).toBeNull();
});
test('aria-labelledby is null if a title is not passed', () => {
render( );
expect(screen.getByRole('img', { hidden: true })).not.toHaveAttribute('aria-labelledby');
});
test('title is rendered', () => {
const title = 'icon title';
render( );
expect(screen.getByText(title)).toBeInTheDocument();
});
test('aria-labelledby matches title id', () => {
render( );
const svg = screen.getByRole('img', { hidden: true });
const labelledby = svg.getAttribute('aria-labelledby');
const titleId = svg.querySelector('title')?.getAttribute('id');
expect(labelledby).toEqual(titleId);
});
test('additional props should be spread to the root svg element', () => {
render( );
expect(screen.getByTestId('icon')).toBeInTheDocument();
});
© 2015 - 2025 Weber Informatics LLC | Privacy Policy