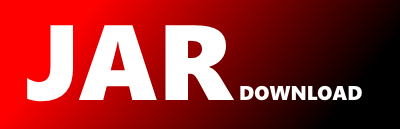
package.build.npm.cjs.utils.featureFlags.js.map Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of browser Show documentation
Show all versions of browser Show documentation
Official Sentry SDK for browsers
{"version":3,"file":"featureFlags.js","sources":["../../../../src/utils/featureFlags.ts"],"sourcesContent":["import type { Event, FeatureFlag } from '@sentry/core';\n\nimport { getCurrentScope, logger } from '@sentry/core';\nimport { DEBUG_BUILD } from '../debug-build';\n\n/**\n * Ordered LRU cache for storing feature flags in the scope context. The name\n * of each flag in the buffer is unique, and the output of getAll() is ordered\n * from oldest to newest.\n */\n\n/**\n * Max size of the LRU flag buffer stored in Sentry scope and event contexts.\n */\nexport const FLAG_BUFFER_SIZE = 100;\n\n/**\n * Copies feature flags that are in current scope context to the event context\n */\nexport function copyFlagsFromScopeToEvent(event: Event): Event {\n const scope = getCurrentScope();\n const flagContext = scope.getScopeData().contexts.flags;\n const flagBuffer = flagContext ? flagContext.values : [];\n\n if (!flagBuffer.length) {\n return event;\n }\n\n if (event.contexts === undefined) {\n event.contexts = {};\n }\n event.contexts.flags = { values: [...flagBuffer] };\n return event;\n}\n\n/**\n * Creates a feature flags values array in current context if it does not exist\n * and inserts the flag into a FeatureFlag array while maintaining ordered LRU\n * properties. Not thread-safe. After inserting:\n * - `flags` is sorted in order of recency, with the newest flag at the end.\n * - No other flags with the same name exist in `flags`.\n * - The length of `flags` does not exceed `maxSize`. The oldest flag is evicted\n * as needed.\n *\n * @param name Name of the feature flag to insert.\n * @param value Value of the feature flag.\n * @param maxSize Max number of flags the buffer should store. It's recommended\n * to keep this consistent across insertions. Default is FLAG_BUFFER_SIZE\n */\nexport function insertFlagToScope(name: string, value: unknown, maxSize: number = FLAG_BUFFER_SIZE): void {\n const scopeContexts = getCurrentScope().getScopeData().contexts;\n if (!scopeContexts.flags) {\n scopeContexts.flags = { values: [] };\n }\n const flags = scopeContexts.flags.values as FeatureFlag[];\n insertToFlagBuffer(flags, name, value, maxSize);\n}\n\n/**\n * Exported for tests. Currently only accepts boolean values (otherwise no-op).\n */\nexport function insertToFlagBuffer(flags: FeatureFlag[], name: string, value: unknown, maxSize: number): void {\n if (typeof value !== 'boolean') {\n return;\n }\n\n if (flags.length > maxSize) {\n DEBUG_BUILD && logger.error(`[Feature Flags] insertToFlagBuffer called on a buffer larger than maxSize=${maxSize}`);\n return;\n }\n\n // Check if the flag is already in the buffer - O(n)\n const index = flags.findIndex(f => f.flag === name);\n\n if (index !== -1) {\n // The flag was found, remove it from its current position - O(n)\n flags.splice(index, 1);\n }\n\n if (flags.length === maxSize) {\n // If at capacity, pop the earliest flag - O(n)\n flags.shift();\n }\n\n // Push the flag to the end - O(1)\n flags.push({\n flag: name,\n result: value,\n });\n}\n"],"names":["getCurrentScope","DEBUG_BUILD","logger"],"mappings":";;;;;AAKA;AACA;AACA;AACA;AACA;;AAEA;AACA;AACA;AACO,MAAM,gBAAiB,GAAE;;AAEhC;AACA;AACA;AACO,SAAS,yBAAyB,CAAC,KAAK,EAAgB;AAC/D,EAAE,MAAM,KAAA,GAAQA,oBAAe,EAAE;AACjC,EAAE,MAAM,WAAY,GAAE,KAAK,CAAC,YAAY,EAAE,CAAC,QAAQ,CAAC,KAAK;AACzD,EAAE,MAAM,UAAW,GAAE,WAAY,GAAE,WAAW,CAAC,MAAA,GAAS,EAAE;;AAE1D,EAAE,IAAI,CAAC,UAAU,CAAC,MAAM,EAAE;AAC1B,IAAI,OAAO,KAAK;AAChB;;AAEA,EAAE,IAAI,KAAK,CAAC,QAAS,KAAI,SAAS,EAAE;AACpC,IAAI,KAAK,CAAC,QAAS,GAAE,EAAE;AACvB;AACA,EAAE,KAAK,CAAC,QAAQ,CAAC,KAAM,GAAE,EAAE,MAAM,EAAE,CAAC,GAAG,UAAU,GAAG;AACpD,EAAE,OAAO,KAAK;AACd;;AAEA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACA;AACO,SAAS,iBAAiB,CAAC,IAAI,EAAU,KAAK,EAAW,OAAO,GAAW,gBAAgB,EAAQ;AAC1G,EAAE,MAAM,aAAc,GAAEA,oBAAe,EAAE,CAAC,YAAY,EAAE,CAAC,QAAQ;AACjE,EAAE,IAAI,CAAC,aAAa,CAAC,KAAK,EAAE;AAC5B,IAAI,aAAa,CAAC,KAAM,GAAE,EAAE,MAAM,EAAE,EAAC,EAAG;AACxC;AACA,EAAE,MAAM,KAAM,GAAE,aAAa,CAAC,KAAK,CAAC,MAAO;AAC3C,EAAE,kBAAkB,CAAC,KAAK,EAAE,IAAI,EAAE,KAAK,EAAE,OAAO,CAAC;AACjD;;AAEA;AACA;AACA;AACO,SAAS,kBAAkB,CAAC,KAAK,EAAiB,IAAI,EAAU,KAAK,EAAW,OAAO,EAAgB;AAC9G,EAAE,IAAI,OAAO,KAAM,KAAI,SAAS,EAAE;AAClC,IAAI;AACJ;;AAEA,EAAE,IAAI,KAAK,CAAC,MAAO,GAAE,OAAO,EAAE;AAC9B,IAAIC,sBAAA,IAAeC,WAAM,CAAC,KAAK,CAAC,CAAC,0EAA0E,EAAE,OAAO,CAAC,CAAA,CAAA;AACA,IAAA;AACA;;AAEA;AACA,EAAA,MAAA,KAAA,GAAA,KAAA,CAAA,SAAA,CAAA,CAAA,IAAA,CAAA,CAAA,IAAA,KAAA,IAAA,CAAA;;AAEA,EAAA,IAAA,KAAA,KAAA,CAAA,CAAA,EAAA;AACA;AACA,IAAA,KAAA,CAAA,MAAA,CAAA,KAAA,EAAA,CAAA,CAAA;AACA;;AAEA,EAAA,IAAA,KAAA,CAAA,MAAA,KAAA,OAAA,EAAA;AACA;AACA,IAAA,KAAA,CAAA,KAAA,EAAA;AACA;;AAEA;AACA,EAAA,KAAA,CAAA,IAAA,CAAA;AACA,IAAA,IAAA,EAAA,IAAA;AACA,IAAA,MAAA,EAAA,KAAA;AACA,GAAA,CAAA;AACA;;;;;;;"}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy