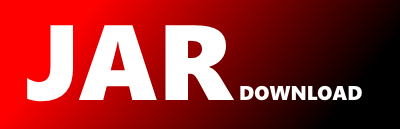
package.src.aggregationFns.ts Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of table-core Show documentation
Show all versions of table-core Show documentation
Headless UI for building powerful tables & datagrids for TS/JS.
The newest version!
import { AggregationFn } from './features/Grouping'
import { isNumberArray } from './utils'
const sum: AggregationFn = (columnId, _leafRows, childRows) => {
// It's faster to just add the aggregations together instead of
// process leaf nodes individually
return childRows.reduce((sum, next) => {
const nextValue = next.getValue(columnId)
return sum + (typeof nextValue === 'number' ? nextValue : 0)
}, 0)
}
const min: AggregationFn = (columnId, _leafRows, childRows) => {
let min: number | undefined
childRows.forEach(row => {
const value = row.getValue(columnId)
if (
value != null &&
(min! > value || (min === undefined && value >= value))
) {
min = value
}
})
return min
}
const max: AggregationFn = (columnId, _leafRows, childRows) => {
let max: number | undefined
childRows.forEach(row => {
const value = row.getValue(columnId)
if (
value != null &&
(max! < value || (max === undefined && value >= value))
) {
max = value
}
})
return max
}
const extent: AggregationFn = (columnId, _leafRows, childRows) => {
let min: number | undefined
let max: number | undefined
childRows.forEach(row => {
const value = row.getValue(columnId)
if (value != null) {
if (min === undefined) {
if (value >= value) min = max = value
} else {
if (min > value) min = value
if (max! < value) max = value
}
}
})
return [min, max]
}
const mean: AggregationFn = (columnId, leafRows) => {
let count = 0
let sum = 0
leafRows.forEach(row => {
let value = row.getValue(columnId)
if (value != null && (value = +value) >= value) {
++count, (sum += value)
}
})
if (count) return sum / count
return
}
const median: AggregationFn = (columnId, leafRows) => {
if (!leafRows.length) {
return
}
const values = leafRows.map(row => row.getValue(columnId))
if (!isNumberArray(values)) {
return
}
if (values.length === 1) {
return values[0]
}
const mid = Math.floor(values.length / 2)
const nums = values.sort((a, b) => a - b)
return values.length % 2 !== 0 ? nums[mid] : (nums[mid - 1]! + nums[mid]!) / 2
}
const unique: AggregationFn = (columnId, leafRows) => {
return Array.from(new Set(leafRows.map(d => d.getValue(columnId))).values())
}
const uniqueCount: AggregationFn = (columnId, leafRows) => {
return new Set(leafRows.map(d => d.getValue(columnId))).size
}
const count: AggregationFn = (_columnId, leafRows) => {
return leafRows.length
}
export const aggregationFns = {
sum,
min,
max,
extent,
mean,
median,
unique,
uniqueCount,
count,
}
export type BuiltInAggregationFn = keyof typeof aggregationFns
© 2015 - 2024 Weber Informatics LLC | Privacy Policy