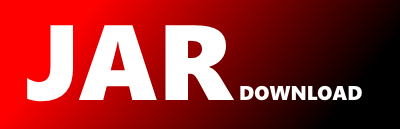
package.dist.index.d.mts Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of carousel Show documentation
Show all versions of carousel Show documentation
Core logic for the carousel widget implemented as a state machine
The newest version!
import * as _zag_js_anatomy from '@zag-js/anatomy';
import { RequiredBy, PropTypes, DirectionProperty, CommonProperties, NormalizeProps } from '@zag-js/types';
import * as _zag_js_core from '@zag-js/core';
import { Machine, StateMachine } from '@zag-js/core';
declare const anatomy: _zag_js_anatomy.AnatomyInstance<"root" | "viewport" | "itemGroup" | "item" | "nextTrigger" | "prevTrigger" | "indicatorGroup" | "indicator">;
interface SlideChangeDetails {
index: number;
}
type RectEdge = "top" | "right" | "bottom" | "left";
type ElementIds = Partial<{
root: string;
viewport: string;
item(index: number): string;
itemGroup: string;
nextTrigger: string;
prevTrigger: string;
indicatorGroup: string;
indicator(index: number): string;
}>;
interface PublicContext extends DirectionProperty, CommonProperties {
/**
* The orientation of the carousel.
* @default "horizontal"
*/
orientation: "horizontal" | "vertical";
/**
* The alignment of the slides in the carousel.
* @default "start"
*/
align: "start" | "center" | "end";
/**
* The number of slides to show at a time.
* @default 1
*/
slidesPerView: number | "auto";
/**
* Whether the carousel should loop around.
* @default false
*/
loop: boolean;
/**
* The current slide index.
*/
index: number;
/**
* The amount of space between slides.
* @default "0px"
*/
spacing: string;
/**
* Function called when the slide changes.
*/
onIndexChange?: ((details: SlideChangeDetails) => void) | undefined;
/**
* The ids of the elements in the carousel. Useful for composition.
*/
ids?: ElementIds | undefined;
}
interface PrivateContext {
slideRects: DOMRect[];
containerRect?: DOMRect | undefined;
containerSize: number;
scrollSnaps: number[];
scrollProgress: number;
}
type ComputedContext = Readonly<{
isRtl: boolean;
isHorizontal: boolean;
isVertical: boolean;
startEdge: RectEdge;
endEdge: RectEdge;
translateValue: string;
canScrollNext: boolean;
canScrollPrev: boolean;
}>;
type UserDefinedContext = RequiredBy;
interface MachineContext extends PublicContext, PrivateContext, ComputedContext {
}
interface MachineState {
value: "idle" | "dragging" | "autoplay";
}
type State = StateMachine.State;
type Send = StateMachine.Send;
type Service = Machine;
interface ItemProps {
/**
* The index of the item.
*/
index: number;
}
interface ItemState {
/**
* The text value of the item. Used for accessibility.
*/
valueText: string;
/**
* Whether the item is the current item in the carousel
*/
current: boolean;
/**
* Whether the item is the next item in the carousel
*/
next: boolean;
/**
* Whether the item is the previous item in the carousel
*/
previous: boolean;
/**
* Whether the item is in view
*/
inView: boolean;
}
interface IndicatorProps {
index: number;
readOnly?: boolean | undefined;
}
interface MachineApi {
/**
* The current index of the carousel
*/
index: number;
/**
* The current scroll progress of the carousel
*/
scrollProgress: number;
/**
* Whether the carousel is auto playing
*/
autoPlaying: boolean;
/**
* Whether the carousel is can scroll to the next slide
*/
canScrollNext: boolean;
/**
* Whether the carousel is can scroll to the previous slide
*/
canScrollPrev: boolean;
/**
* Function to scroll to a specific slide index
*/
scrollTo(index: number, jump?: boolean): void;
/**
* Function to scroll to the next slide
*/
scrollToNext(): void;
/**
* Function to scroll to the previous slide
*/
scrollToPrevious(): void;
/**
* Returns the state of a specific slide
*/
getItemState(props: ItemProps): ItemState;
/**
* Function to start/resume autoplay
*/
play(): void;
/**
* Function to pause autoplay
*/
pause(): void;
getRootProps(): T["element"];
getViewportProps(): T["element"];
getItemGroupProps(): T["element"];
getItemProps(props: ItemProps): T["element"];
getPrevTriggerProps(): T["button"];
getNextTriggerProps(): T["button"];
getIndicatorGroupProps(): T["element"];
getIndicatorProps(props: IndicatorProps): T["button"];
}
declare function connect(state: State, send: Send, normalize: NormalizeProps): MachineApi;
declare function machine(userContext: UserDefinedContext): _zag_js_core.Machine;
declare const props: ("dir" | "id" | "getRootNode" | "loop" | "align" | "orientation" | "spacing" | "slidesPerView" | "index" | "onIndexChange" | "ids")[];
declare const splitProps: >(props: Props) => [Partial, Omit];
declare const indicatorProps: (keyof IndicatorProps)[];
declare const splitIndicatorProps: (props: Props) => [IndicatorProps, Omit];
export { type MachineApi as Api, type UserDefinedContext as Context, type ElementIds, type IndicatorProps, type ItemProps, type ItemState, type Service, type SlideChangeDetails, anatomy, connect, indicatorProps, machine, props, splitIndicatorProps, splitProps };
© 2015 - 2025 Weber Informatics LLC | Privacy Policy