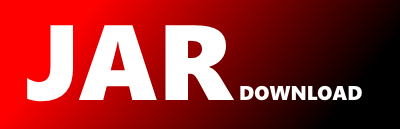
package.dist.index.d.mts Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of checkbox Show documentation
Show all versions of checkbox Show documentation
Core logic for the checkbox widget implemented as a state machine
The newest version!
import * as _zag_js_anatomy from '@zag-js/anatomy';
import { RequiredBy, PropTypes, DirectionProperty, CommonProperties, NormalizeProps } from '@zag-js/types';
import * as _zag_js_core from '@zag-js/core';
import { Machine, StateMachine } from '@zag-js/core';
declare const anatomy: _zag_js_anatomy.AnatomyInstance<"root" | "label" | "control" | "indicator">;
type CheckedState = boolean | "indeterminate";
interface CheckedChangeDetails {
checked: CheckedState;
}
type ElementIds = Partial<{
root: string;
hiddenInput: string;
control: string;
label: string;
}>;
interface PublicContext extends DirectionProperty, CommonProperties {
/**
* The ids of the elements in the checkbox. Useful for composition.
*/
ids?: ElementIds | undefined;
/**
* Whether the checkbox is disabled
*/
disabled?: boolean | undefined;
/**
* Whether the checkbox is invalid
*/
invalid?: boolean | undefined;
/**
* Whether the checkbox is required
*/
required?: boolean | undefined;
/**
* The checked state of the checkbox
*/
checked: CheckedState;
/**
* Whether the checkbox is read-only
*/
readOnly?: boolean | undefined;
/**
* The callback invoked when the checked state changes.
*/
onCheckedChange?(details: CheckedChangeDetails): void;
/**
* The name of the input field in a checkbox.
* Useful for form submission.
*/
name?: string | undefined;
/**
* The id of the form that the checkbox belongs to.
*/
form?: string | undefined;
/**
* The value of checkbox input. Useful for form submission.
* @default "on"
*/
value: string;
}
type UserDefinedContext = RequiredBy;
type ComputedContext = Readonly<{
/**
* Whether the checkbox is checked
*/
isIndeterminate: boolean;
/**
* Whether the checkbox is checked
*/
isChecked: boolean;
/**
* Whether the checkbox is disabled
*/
isDisabled: boolean;
}>;
interface PrivateContext {
}
interface MachineContext extends PublicContext, PrivateContext, ComputedContext {
}
interface MachineState {
value: "ready";
}
type State = StateMachine.State;
type Send = StateMachine.Send;
type Service = Machine;
interface MachineApi {
/**
* Whether the checkbox is checked
*/
checked: boolean;
/**
* Whether the checkbox is disabled
*/
disabled: boolean | undefined;
/**
* Whether the checkbox is indeterminate
*/
indeterminate: boolean;
/**
* Whether the checkbox is focused
*/
focused: boolean | undefined;
/**
* The checked state of the checkbox
*/
checkedState: CheckedState;
/**
* Function to set the checked state of the checkbox
*/
setChecked(checked: CheckedState): void;
/**
* Function to toggle the checked state of the checkbox
*/
toggleChecked(): void;
getRootProps(): T["label"];
getLabelProps(): T["element"];
getControlProps(): T["element"];
getHiddenInputProps(): T["input"];
getIndicatorProps(): T["element"];
}
declare function connect(state: State, send: Send, normalize: NormalizeProps): MachineApi;
declare function machine(userContext: UserDefinedContext): _zag_js_core.Machine;
declare const props: ("form" | "value" | "dir" | "id" | "getRootNode" | "name" | "invalid" | "disabled" | "checked" | "required" | "ids" | "readOnly" | "onCheckedChange")[];
declare const splitProps: >(props: Props) => [Partial, Omit];
export { type MachineApi as Api, type CheckedChangeDetails, type CheckedState, type UserDefinedContext as Context, type ElementIds, type Service, anatomy, connect, machine, props, splitProps };
© 2015 - 2025 Weber Informatics LLC | Privacy Policy