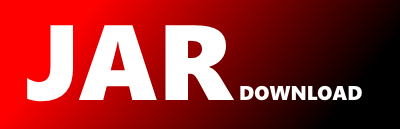
package.dist.index.d.ts Maven / Gradle / Ivy
interface CollectionSearchState {
keysSoFar: string;
timer: number;
}
interface CollectionSearchOptions {
state: CollectionSearchState;
currentValue: string | null;
timeout?: number | undefined;
}
type CollectionItem = any;
interface CollectionMethods {
/**
* The value of the item
*/
itemToValue: (item: T) => string;
/**
* The label of the item
*/
itemToString: (item: T) => string;
/**
* Whether the item is disabled
*/
isItemDisabled: (item: T) => boolean;
}
interface CollectionOptions extends Partial> {
/**
* The options of the select
*/
items: T[] | readonly T[];
}
declare class ListCollection {
private options;
/**
* The items in the collection
*/
items: T[];
constructor(options: CollectionOptions);
isEqual(other: ListCollection): boolean;
/**
* Function to update the collection items
*/
setItems(items: T[] | readonly T[]): void;
/**
* Returns all the values in the collection
*/
getValues(items?: T[]): string[];
/**
* Get the item based on its value
*/
find(value: string | null | undefined): T | null;
/**
* Get the items based on its values
*/
findMany(values: string[]): T[];
/**
* Get the item based on its index
*/
at(index: number): T | null;
private sortFn;
/**
* Sort the values based on their index
*/
sort(values: string[]): string[];
/**
* Convert an item to a value
*/
getItemValue(item: T | null | undefined): string | null;
/**
* Whether an item is disabled
*/
getItemDisabled(item: T | null): boolean;
/**
* Convert an item to a string
*/
stringifyItem(item: T | null): string | null;
/**
* Convert a value to a string
*/
stringify(value: string | null): string | null;
/**
* Convert an array of items to a string
*/
stringifyItems(items: T[], separator?: string): string;
/**
* Convert an array of items to a string
*/
stringifyMany(value: string[], separator?: string): string;
/**
* Whether the collection has a value
*/
has(value: string | null): boolean;
/**
* Whether the collection has an item
*/
hasItem(item: T | null): boolean;
/**
* Returns the number of items in the collection
*/
get size(): number;
/**
* Returns the first value in the collection
*/
get firstValue(): string | null;
/**
* Returns the last value in the collection
*/
get lastValue(): string | null;
/**
* Returns the next value in the collection
*/
getNextValue(value: string, step?: number, clamp?: boolean): string | null;
/**
* Returns the previous value in the collection
*/
getPreviousValue(value: string, step?: number, clamp?: boolean): string | null;
/**
* Get the index of an item based on its key
*/
indexOf(value: string | null): number;
private getByText;
/**
* Search for a value based on a query
*/
search(queryString: string, options: CollectionSearchOptions): string | null;
[Symbol.iterator](): Generator;
insertBefore(value: string, item: T): void;
insertAfter(value: string, item: T): void;
reorder(fromIndex: number, toIndex: number): void;
json(): {
size: number;
first: string | null;
last: string | null;
};
}
interface GridCollectionOptions extends CollectionOptions {
columnCount: number;
}
declare class GridCollection extends ListCollection {
columnCount: number;
constructor(options: GridCollectionOptions);
/**
* Returns the row data in the grid
*/
getRows(): T[][];
/**
* Returns the number of rows in the grid
*/
getRowCount(): number;
/**
* Returns the index of the specified row and column in the grid
*/
getCellIndex(row: number, column: number): number;
/**
* Returns the item at the specified row and column in the grid
*/
getCell(row: number, column: number): T | null;
/**
* Returns the value of the previous row in the grid, based on the current value
*/
getPreviousRowValue(value: string, clamp?: boolean): string | null;
/**
* Returns the value of the next row in the grid, based on the current value
*/
getNextRowValue(value: string, clamp?: boolean): string | null;
}
type IndexPath = number[];
interface BaseOptions {
getChildren: (node: T, indexPath: IndexPath) => T[];
reuseIndexPath?: boolean;
}
type EnterReturnValue = void | "skip" | "stop";
type LeaveReturnValue = void | "stop";
interface VisitOptions extends BaseOptions {
onEnter?(node: T, indexPath: IndexPath): EnterReturnValue;
onLeave?(node: T, indexPath: IndexPath): LeaveReturnValue;
}
declare class TreeCollection {
private options;
rootNode: T;
constructor(options: TreeCollectionOptions);
isEqual(other: TreeCollection): boolean;
getNodeChildren: (node: T) => any[];
getNodeValue: (node: T) => string;
getNodeDisabled: (node: T) => boolean;
stringify: (value: string) => string | null;
stringifyNode: (node: T) => string;
getFirstNode: (rootNode?: T) => T | undefined;
getLastNode: (rootNode?: T, opts?: SkipProperty) => T | undefined;
at(indexPath: number[]): T;
findNode: (value: string, rootNode?: T) => T | undefined;
sort: (values: string[]) => string[];
getIndexPath: (value: string) => IndexPath | undefined;
getValuePath: (indexPath: number[] | undefined) => string[];
getDepth: (value: string) => number;
isRootNode: (node: T) => boolean;
contains: (parentIndexPath: number[], valueIndexPath: number[]) => boolean;
getNextNode: (value: string, opts?: SkipProperty) => T | undefined;
getPreviousNode: (value: string, opts?: SkipProperty) => T | undefined;
getParentNodes: (values: string) => T[];
getParentNode: (value: string) => T | undefined;
visit: (opts: Omit, "getChildren"> & SkipProperty) => void;
getSiblingNodes: (indexPath: number[]) => T[];
getValues: (rootNode?: T) => string[];
private isSameDepth;
isBranchNode: (node: T) => boolean;
getBranchValues: (rootNode?: T, opts?: SkipProperty & {
depth?: number;
}) => string[];
flatten: (rootNode?: T) => FlatTreeNode[];
private _create;
private _insert;
private _replace;
private _move;
replace: (indexPath: number[], node: T) => T;
insertBefore: (indexPath: number[], ...nodes: T[]) => T | undefined;
insertAfter: (indexPath: number[], ...nodes: T[]) => T | undefined;
reorder: (toIndexPath: number[], ...fromIndexPaths: number[][]) => T;
json(): string[];
}
declare function flattenedToTree(nodes: FlatTreeNode[]): TreeCollection<{
value: string;
}>;
interface FilePathTreeNode {
label: string;
value: string;
children?: FilePathTreeNode[];
}
declare function filePathToTree(paths: string[]): TreeCollection;
interface TreeCollectionMethods {
isNodeDisabled: (node: T) => boolean;
nodeToValue: (node: T) => string;
nodeToString: (node: T) => string;
nodeToChildren: (node: T) => any[];
}
interface TreeCollectionOptions extends Partial> {
rootNode: T;
}
type TreeNode = any;
interface FlatTreeNode {
label?: string | undefined;
value: string;
indexPath: number[];
children?: string[] | undefined;
}
type TreeSkipFn = (opts: {
value: string;
node: T;
indexPath: number[];
}) => boolean | void;
interface SkipProperty {
skip?: TreeSkipFn;
}
export { type CollectionItem, type CollectionMethods, type CollectionOptions, type CollectionSearchOptions, type CollectionSearchState, type FilePathTreeNode, type FlatTreeNode, GridCollection, type GridCollectionOptions, ListCollection, TreeCollection, type TreeCollectionMethods, type TreeCollectionOptions, type TreeNode, type TreeSkipFn, filePathToTree, flattenedToTree };
© 2015 - 2025 Weber Informatics LLC | Privacy Policy