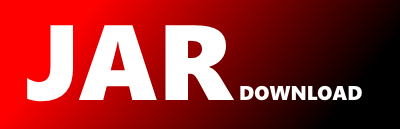
package.dist.index.d.ts Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of color-picker Show documentation
Show all versions of color-picker Show documentation
Core logic for the color-picker widget implemented as a state machine
The newest version!
import { InteractOutsideHandlers } from '@zag-js/dismissable';
export { FocusOutsideEvent, InteractOutsideEvent, PointerDownOutsideEvent } from '@zag-js/dismissable';
import * as _zag_js_anatomy from '@zag-js/anatomy';
import { RequiredBy, Orientation, PropTypes, CommonProperties, DirectionProperty, NormalizeProps } from '@zag-js/types';
import { Color, ColorFormat, ColorChannel } from '@zag-js/color-utils';
export { Color, ColorAxes, ColorChannel, ColorFormat, ColorType } from '@zag-js/color-utils';
import * as _zag_js_core from '@zag-js/core';
import { Machine, StateMachine } from '@zag-js/core';
import { PositioningOptions } from '@zag-js/popper';
export { PositioningOptions } from '@zag-js/popper';
declare const anatomy: _zag_js_anatomy.Anatomy<"area" | "label" | "root" | "content" | "control" | "trigger" | "positioner" | "areaThumb" | "valueText" | "areaBackground" | "channelSlider" | "channelSliderLabel" | "channelSliderTrack" | "channelSliderThumb" | "channelSliderValueText" | "channelInput" | "transparencyGrid" | "swatchGroup" | "swatchTrigger" | "swatchIndicator" | "swatch" | "eyeDropperTrigger" | "formatTrigger" | "formatSelect">;
type ExtendedColorChannel = ColorChannel | "hex" | "css";
interface EyeDropper {
new (): EyeDropper;
open: (options?: {
signal?: AbortSignal;
}) => Promise<{
sRGBHex: string;
}>;
[Symbol.toStringTag]: "EyeDropper";
}
declare global {
interface Window {
EyeDropper: EyeDropper;
}
}
interface ValueChangeDetails {
value: Color;
valueAsString: string;
}
interface OpenChangeDetails {
open: boolean;
}
interface FormatChangeDetails {
format: ColorFormat;
}
type ElementIds = Partial<{
root: string;
control: string;
trigger: string;
label: string;
input: string;
hiddenInput: string;
content: string;
area: string;
areaGradient: string;
positioner: string;
formatSelect: string;
areaThumb: string;
channelInput(id: string): string;
channelSliderTrack(id: ColorChannel): string;
channelSliderThumb(id: ColorChannel): string;
}>;
interface PublicContext extends CommonProperties, DirectionProperty, InteractOutsideHandlers {
/**
* The ids of the elements in the color picker. Useful for composition.
*/
ids?: ElementIds | undefined;
/**
* The current color value
* @default #000000
*/
value: Color;
/**
* Whether the color picker is disabled
*/
disabled?: boolean | undefined;
/**
* Whether the color picker is read-only
*/
readOnly?: boolean | undefined;
/**
* Whether the color picker is required
*/
required?: boolean | undefined;
/**
* Handler that is called when the value changes, as the user drags.
*/
onValueChange?: ((details: ValueChangeDetails) => void) | undefined;
/**
* Handler that is called when the user stops dragging.
*/
onValueChangeEnd?: ((details: ValueChangeDetails) => void) | undefined;
/**
* Handler that is called when the user opens or closes the color picker.
*/
onOpenChange?: ((details: OpenChangeDetails) => void) | undefined;
/**
* The name for the form input
*/
name?: string | undefined;
/**
* The positioning options for the color picker
*/
positioning: PositioningOptions;
/**
* The initial focus element when the color picker is opened.
*/
initialFocusEl?: (() => HTMLElement | null) | undefined;
/**
* Whether the color picker is open
*/
open?: boolean | undefined;
/**
* Whether the color picker open state is controlled by the user
*/
"open.controlled"?: boolean | undefined;
/**
* The color format to use
* @default "rgba"
*/
format: ColorFormat;
/**
* Function called when the color format changes
*/
onFormatChange?: ((details: FormatChangeDetails) => void) | undefined;
/**
* Whether to close the color picker when a swatch is selected
* @default false
*/
closeOnSelect?: boolean | undefined;
}
interface PrivateContext {
}
type ComputedContext = Readonly<{
/**
* @computed
* Whether the color picker is in RTL mode
*/
isRtl: boolean;
/**
* @computed
* Whether the color picker is interactive
*/
isInteractive: boolean;
/**
* @computed
* The color value as a Color object
*/
valueAsString: string;
/**
* @computed
* Whether the color picker is disabled
*/
isDisabled: boolean;
/**
* @computed
* The area value as a Color object
*/
areaValue: Color;
}>;
type UserDefinedContext = RequiredBy;
interface MachineContext extends PublicContext, PrivateContext, ComputedContext {
}
interface MachineState {
tags: "open" | "closed" | "dragging" | "focused";
value: "idle" | "focused" | "open" | "open:dragging";
}
type State = StateMachine.State;
type Send = StateMachine.Send;
type Service = Machine;
interface ChannelProps {
channel: ColorChannel;
orientation?: Orientation | undefined;
}
interface ChannelSliderProps extends ChannelProps {
format?: ColorFormat | undefined;
}
interface ChannelInputProps {
channel: ExtendedColorChannel;
orientation?: Orientation | undefined;
}
interface AreaProps {
xChannel?: ColorChannel | undefined;
yChannel?: ColorChannel | undefined;
}
interface SwatchTriggerProps {
/**
* The color value
*/
value: string | Color;
/**
* Whether the swatch trigger is disabled
*/
disabled?: boolean | undefined;
}
interface SwatchTriggerState {
value: Color;
valueAsString: string;
checked: boolean;
disabled: boolean;
}
interface SwatchProps {
/**
* The color value
*/
value: string | Color;
/**
* Whether to include the alpha channel in the color
*/
respectAlpha?: boolean | undefined;
}
interface TransparencyGridProps {
size?: string | undefined;
}
interface MachineApi {
/**
* Whether the color picker is being dragged
*/
dragging: boolean;
/**
* Whether the color picker is open
*/
open: boolean;
/**
* The current color value (as a string)
*/
value: Color;
/**
* The current color value (as a Color object)
*/
valueAsString: string;
/**
* Function to set the color value
*/
setValue(value: string | Color): void;
/**
* Function to set the color value
*/
getChannelValue(channel: ColorChannel): string;
/**
* Function to get the formatted and localized value of a specific channel
*/
getChannelValueText(channel: ColorChannel, locale: string): string;
/**
* Function to set the color value of a specific channel
*/
setChannelValue(channel: ColorChannel, value: number): void;
/**
* The current color format
*/
format: ColorFormat;
/**
* Function to set the color format
*/
setFormat(format: ColorFormat): void;
/**
* The alpha value of the color
*/
alpha: number;
/**
* Function to set the color alpha
*/
setAlpha(value: number): void;
/**
* Function to open or close the color picker
*/
setOpen(open: boolean): void;
getRootProps(): T["element"];
getLabelProps(): T["element"];
getControlProps(): T["element"];
getTriggerProps(): T["button"];
getPositionerProps(): T["element"];
getContentProps(): T["element"];
getHiddenInputProps(): T["input"];
getValueTextProps(): T["element"];
getAreaProps(props?: AreaProps): T["element"];
getAreaBackgroundProps(props?: AreaProps): T["element"];
getAreaThumbProps(props?: AreaProps): T["element"];
getChannelInputProps(props: ChannelInputProps): T["input"];
getChannelSliderProps(props: ChannelSliderProps): T["element"];
getChannelSliderTrackProps(props: ChannelSliderProps): T["element"];
getChannelSliderThumbProps(props: ChannelSliderProps): T["element"];
getChannelSliderLabelProps(props: ChannelProps): T["element"];
getChannelSliderValueTextProps(props: ChannelProps): T["element"];
getTransparencyGridProps(props?: TransparencyGridProps): T["element"];
getEyeDropperTriggerProps(): T["button"];
getSwatchGroupProps(): T["element"];
getSwatchTriggerProps(props: SwatchTriggerProps): T["button"];
getSwatchTriggerState(props: SwatchTriggerProps): SwatchTriggerState;
getSwatchProps(props: SwatchProps): T["element"];
getSwatchIndicatorProps(props: SwatchProps): T["element"];
getFormatSelectProps(): T["select"];
getFormatTriggerProps(): T["button"];
}
declare function connect(state: State, send: Send, normalize: NormalizeProps): MachineApi;
declare function machine(userContext: UserDefinedContext): _zag_js_core.Machine;
declare const parse: (colorString: string) => Color;
export { type MachineApi as Api, type AreaProps, type ChannelInputProps, type ChannelProps, type ChannelSliderProps, type UserDefinedContext as Context, type ElementIds, type FormatChangeDetails, type OpenChangeDetails, type Service, type SwatchProps, type SwatchTriggerProps, type SwatchTriggerState, type TransparencyGridProps, type ValueChangeDetails, anatomy, connect, machine, parse };
© 2015 - 2025 Weber Informatics LLC | Privacy Policy