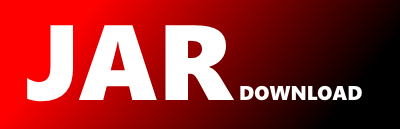
package.dist.index.d.mts Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of editable Show documentation
Show all versions of editable Show documentation
Core logic for the editable widget implemented as a state machine
The newest version!
import { InteractOutsideHandlers } from '@zag-js/interact-outside';
export { FocusOutsideEvent, InteractOutsideEvent, PointerDownOutsideEvent } from '@zag-js/interact-outside';
import * as _zag_js_anatomy from '@zag-js/anatomy';
import { RequiredBy, PropTypes, DirectionProperty, CommonProperties, NormalizeProps } from '@zag-js/types';
import * as _zag_js_core from '@zag-js/core';
import { Machine, StateMachine } from '@zag-js/core';
declare const anatomy: _zag_js_anatomy.AnatomyInstance<"input" | "area" | "label" | "root" | "preview" | "editTrigger" | "submitTrigger" | "cancelTrigger" | "control">;
interface ValueChangeDetails {
value: string;
}
interface EditChangeDetails {
edit: boolean;
}
type ActivationMode = "focus" | "dblclick" | "click";
type SubmitMode = "enter" | "blur" | "both" | "none";
type IntlTranslations = {
edit: string;
submit: string;
cancel: string;
input: string;
};
type ElementIds = Partial<{
root: string;
area: string;
label: string;
preview: string;
input: string;
control: string;
submitTrigger: string;
cancelTrigger: string;
editTrigger: string;
}>;
interface PublicContext extends DirectionProperty, CommonProperties, InteractOutsideHandlers {
/**
* The ids of the elements in the editable. Useful for composition.
*/
ids?: ElementIds | undefined;
/**
* Whether the input's value is invalid.
*/
invalid?: boolean | undefined;
/**
* The name attribute of the editable component. Used for form submission.
*/
name?: string | undefined;
/**
* The associate form of the underlying input.
*/
form?: string | undefined;
/**
* Whether the editable should auto-resize to fit the content.
*/
autoResize?: boolean | undefined;
/**
* The activation mode for the preview element.
*
* - "focus" - Enter edit mode when the preview is focused
* - "dblclick" - Enter edit mode when the preview is double-clicked
* - "click" - Enter edit mode when the preview is clicked
*
* @default "focus"
*/
activationMode: ActivationMode;
/**
* The action that triggers submit in the edit mode:
*
* - "enter" - Trigger submit when the enter key is pressed
* - "blur" - Trigger submit when the editable is blurred
* - "none" - No action will trigger submit. You need to use the submit button
* - "both" - Pressing `Enter` and blurring the input will trigger submit
*
* @default "both"
*/
submitMode: SubmitMode;
/**
* Whether the editable is in edit mode.
*/
edit?: boolean | undefined;
/**
* Whether the editable is controlled
*/
"edit.controlled"?: boolean | undefined;
/**
* Whether to select the text in the input when it is focused.
* @default true
*/
selectOnFocus?: boolean | undefined;
/**
* The value of the editable in both edit and preview mode
*/
value: string;
/**
* The maximum number of characters allowed in the editable
*/
maxLength?: number | undefined;
/**
* Whether the editable is disabled
*/
disabled?: boolean | undefined;
/**
* Whether the editable is readonly
*/
readOnly?: boolean | undefined;
/**
* Whether the editable is required
*/
required?: boolean | undefined;
/**
* The callback that is called when the editable's value is changed
*/
onValueChange?: ((details: ValueChangeDetails) => void) | undefined;
/**
* The callback that is called when the esc key is pressed or the cancel button is clicked
*/
onValueRevert?: ((details: ValueChangeDetails) => void) | undefined;
/**
* The callback that is called when the editable's value is submitted.
*/
onValueCommit?: ((details: ValueChangeDetails) => void) | undefined;
/**
* The callback that is called when the edit mode is changed
*/
onEditChange?: ((details: EditChangeDetails) => void) | undefined;
/**
* The placeholder value to show when the `value` is empty
*/
placeholder?: string | {
edit: string;
preview: string;
} | undefined;
/**
* Specifies the localized strings that identifies the accessibility elements and their states
*/
translations: IntlTranslations;
/**
* The element that should receive focus when the editable is closed.
* By default, it will focus on the trigger element.
*/
finalFocusEl?: (() => HTMLElement | null) | undefined;
}
type UserDefinedContext = RequiredBy;
type ComputedContext = Readonly<{
/**
* @computed
* Whether the editable can be interacted with
*/
isInteractive: boolean;
/**
* @computed
* Whether to submit on enter press
*/
submitOnEnter: boolean;
/**
* @computed
* Whether to submit on blur
*/
submitOnBlur: boolean;
}>;
interface PrivateContext {
}
interface MachineContext extends PublicContext, PrivateContext, ComputedContext {
}
interface MachineState {
value: "preview" | "edit";
}
type State = StateMachine.State;
type Send = StateMachine.Send;
type Service = Machine;
interface MachineApi {
/**
* Whether the editable is in edit mode
*/
editing: boolean;
/**
* Whether the editable value is empty
*/
empty: boolean;
/**
* The current value of the editable
*/
value: string;
/**
* The current value of the editable, or the placeholder if the value is empty
*/
valueText: string;
/**
* Function to set the value of the editable
*/
setValue(value: string): void;
/**
* Function to clear the value of the editable
*/
clearValue(): void;
/**
* Function to enter edit mode
*/
edit(): void;
/**
* Function to exit edit mode, and discard any changes
*/
cancel(): void;
/**
* Function to exit edit mode, and submit any changes
*/
submit(): void;
getRootProps(): T["element"];
getAreaProps(): T["element"];
getLabelProps(): T["label"];
getInputProps(): T["input"];
getPreviewProps(): T["element"];
getEditTriggerProps(): T["button"];
getControlProps(): T["element"];
getSubmitTriggerProps(): T["button"];
getCancelTriggerProps(): T["button"];
}
declare function connect(state: State, send: Send, normalize: NormalizeProps): MachineApi;
declare function machine(userContext: UserDefinedContext): _zag_js_core.Machine;
declare const props: ("invalid" | "form" | "value" | "dir" | "id" | "getRootNode" | "name" | "disabled" | "placeholder" | "maxLength" | "required" | "onFocusOutside" | "onPointerDownOutside" | "onInteractOutside" | "ids" | "autoResize" | "activationMode" | "submitMode" | "edit" | "edit.controlled" | "selectOnFocus" | "readOnly" | "onValueChange" | "onValueRevert" | "onValueCommit" | "onEditChange" | "translations" | "finalFocusEl")[];
declare const splitProps: >(props: Props) => [Partial, Omit];
export { type ActivationMode, type MachineApi as Api, type UserDefinedContext as Context, type EditChangeDetails, type ElementIds, type IntlTranslations, type Service, type SubmitMode, type ValueChangeDetails, anatomy, connect, machine, props, splitProps };
© 2015 - 2025 Weber Informatics LLC | Privacy Policy