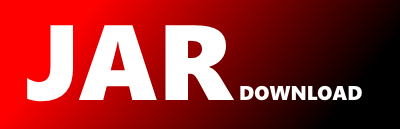
package.dist.index.d.mts Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of file-upload Show documentation
Show all versions of file-upload Show documentation
Core logic for the file-upload widget implemented as a state machine
The newest version!
import * as _zag_js_anatomy from '@zag-js/anatomy';
import { RequiredBy, PropTypes, LocaleProperties, CommonProperties, NormalizeProps } from '@zag-js/types';
import * as _zag_js_core from '@zag-js/core';
import { Machine, StateMachine } from '@zag-js/core';
import { FileError, FileMimeType } from '@zag-js/file-utils';
export { FileError, FileMimeType } from '@zag-js/file-utils';
declare const anatomy: _zag_js_anatomy.AnatomyInstance<"root" | "dropzone" | "item" | "itemDeleteTrigger" | "itemGroup" | "itemName" | "itemPreview" | "itemPreviewImage" | "itemSizeText" | "label" | "trigger" | "clearTrigger">;
interface FileRejection {
file: File;
errors: FileError[];
}
interface FileChangeDetails {
acceptedFiles: File[];
rejectedFiles: FileRejection[];
}
interface FileAcceptDetails {
files: File[];
}
interface FileRejectDetails {
files: FileRejection[];
}
type ElementIds = Partial<{
root: string;
dropzone: string;
hiddenInput: string;
trigger: string;
label: string;
item(id: string): string;
itemName(id: string): string;
itemSizeText(id: string): string;
itemPreview(id: string): string;
}>;
interface IntlTranslations {
dropzone?: string | undefined;
itemPreview?(file: File): string;
deleteFile?(file: File): string;
}
interface PublicContext extends LocaleProperties, CommonProperties {
/**
* The name of the underlying file input
*/
name?: string | undefined;
/**
* The ids of the elements. Useful for composition.
*/
ids?: ElementIds | undefined;
/**
* The localized messages to use.
*/
translations: IntlTranslations;
/**
* The accept file types
*/
accept?: Record | FileMimeType | FileMimeType[] | undefined;
/**
* Whether the file input is disabled
*/
disabled?: boolean | undefined;
/**
* Whether the file input is required
*/
required?: boolean | undefined;
/**
* Whether to allow drag and drop in the dropzone element
* @default true
*/
allowDrop?: boolean | undefined;
/**
* The maximum file size in bytes
*
* @default Infinity
*/
maxFileSize: number;
/**
* The minimum file size in bytes
*
* @default 0
*/
minFileSize: number;
/**
* The maximum number of files
* @default 1
*/
maxFiles: number;
/**
* Function to validate a file
*/
validate?: ((file: File) => FileError[] | null) | undefined;
/**
* Function called when the value changes, whether accepted or rejected
*/
onFileChange?: ((details: FileChangeDetails) => void) | undefined;
/**
* Function called when the file is accepted
*/
onFileAccept?: ((details: FileAcceptDetails) => void) | undefined;
/**
* Function called when the file is rejected
*/
onFileReject?: ((details: FileRejectDetails) => void) | undefined;
/**
* The default camera to use when capturing media
*/
capture?: "user" | "environment" | undefined;
/**
* Whether to accept directories, only works in webkit browsers
*/
directory?: boolean | undefined;
/**
* Whether the file input is invalid
*/
invalid?: boolean | undefined;
}
interface PrivateContext {
}
type ComputedContext = Readonly<{
/**
* @computed
* The accept attribute as a string
*/
acceptAttr: string | undefined;
/**
* @computed
* Whether the file can select multiple files
*/
multiple: boolean;
}>;
type UserDefinedContext = RequiredBy;
interface MachineContext extends PublicContext, PrivateContext, ComputedContext {
}
interface MachineState {
value: "idle" | "focused" | "open" | "dragging";
}
type State = StateMachine.State;
type Send = StateMachine.Send;
type Service = Machine;
interface ItemProps {
file: File;
}
interface ItemPreviewImageProps extends ItemProps {
url: string;
}
interface MachineApi {
/**
* Whether the user is dragging something over the root element
*/
dragging: boolean;
/**
* Whether the user is focused on the dropzone element
*/
focused: boolean;
/**
* Function to open the file dialog
*/
openFilePicker(): void;
/**
* Function to delete the file from the list
*/
deleteFile(file: File): void;
/**
* The accepted files that have been dropped or selected
*/
acceptedFiles: File[];
/**
* The files that have been rejected
*/
rejectedFiles: FileRejection[];
/**
* Function to set the value
*/
setFiles(files: File[]): void;
/**
* Function to clear the value
*/
clearFiles(): void;
/**
* Function to clear the rejected files
*/
clearRejectedFiles(): void;
/**
* Function to format the file size (e.g. 1.2MB)
*/
getFileSize(file: File): string;
/**
* Function to get the preview url of a file.
* It returns a function to revoke the url.
*/
createFileUrl(file: File, cb: (url: string) => void): VoidFunction;
getLabelProps(): T["label"];
getRootProps(): T["element"];
getDropzoneProps(): T["element"];
getTriggerProps(): T["button"];
getHiddenInputProps(): T["input"];
getItemGroupProps(): T["element"];
getItemProps(props: ItemProps): T["element"];
getItemNameProps(props: ItemProps): T["element"];
getItemPreviewProps(props: ItemProps): T["element"];
getItemPreviewImageProps(props: ItemPreviewImageProps): T["img"];
getItemSizeTextProps(props: ItemProps): T["element"];
getItemDeleteTriggerProps(props: ItemProps): T["button"];
getClearTriggerProps(): T["button"];
}
declare function connect(state: State, send: Send, normalize: NormalizeProps): MachineApi;
declare function machine(userContext: UserDefinedContext): _zag_js_core.Machine;
declare const props: ("dir" | "id" | "getRootNode" | "name" | "invalid" | "locale" | "disabled" | "directory" | "accept" | "capture" | "required" | "ids" | "translations" | "allowDrop" | "maxFileSize" | "minFileSize" | "maxFiles" | "validate" | "onFileChange" | "onFileAccept" | "onFileReject")[];
declare const splitProps: >(props: Props) => [Partial, Omit];
declare const itemProps: "file"[];
declare const splitItemProps: (props: Props) => [ItemProps, Omit];
export { type MachineApi as Api, type UserDefinedContext as Context, type ElementIds, type FileAcceptDetails, type FileChangeDetails, type FileRejectDetails, type FileRejection, type IntlTranslations, type ItemPreviewImageProps, type ItemProps, type Service, anatomy, connect, itemProps, machine, props, splitItemProps, splitProps };
© 2015 - 2025 Weber Informatics LLC | Privacy Policy