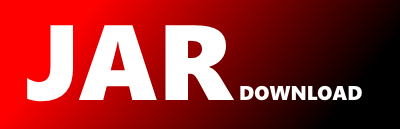
package.dist.index.d.ts Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of progress Show documentation
Show all versions of progress Show documentation
Core logic for the progress widget implemented as a state machine
The newest version!
import * as _zag_js_anatomy from '@zag-js/anatomy';
import { RequiredBy, PropTypes, DirectionProperty, CommonProperties, OrientationProperty, NormalizeProps } from '@zag-js/types';
export { Orientation } from '@zag-js/types';
import * as _zag_js_core from '@zag-js/core';
import { Machine, StateMachine } from '@zag-js/core';
declare const anatomy: _zag_js_anatomy.AnatomyInstance<"root" | "label" | "track" | "range" | "valueText" | "view" | "circle" | "circleTrack" | "circleRange">;
type ProgressState = "indeterminate" | "loading" | "complete";
interface ValueTranslationDetails {
value: number | null;
max: number;
min: number;
percent: number;
}
interface IntlTranslations {
value(details: ValueTranslationDetails): string;
}
type ElementIds = Partial<{
root: string;
track: string;
label: string;
circle: string;
}>;
interface PublicContext extends DirectionProperty, CommonProperties, OrientationProperty {
/**
* The ids of the elements in the progress bar. Useful for composition.
*/
ids?: ElementIds | undefined;
/**
* The current value of the progress bar.
* @default 50
*/
value: number | null;
/**
* The minimum allowed value of the progress bar.
* @default 0
*/
min: number;
/**
* The maximum allowed value of the progress bar.
* @default 100
*/
max: number;
/**
* The localized messages to use.
*/
translations: IntlTranslations;
}
interface PrivateContext {
}
type ComputedContext = Readonly<{
/**
* @computed
* Whether the progress bar is indeterminate.
*/
isIndeterminate: boolean;
/**
* @computed
* The percentage of the progress bar's value.
*/
percent: number;
/**
* @computed
* Whether the progress bar is at its minimum value.
*/
isAtMax: boolean;
/**
* @computed
* Whether the progress bar is horizontal.
*/
isHorizontal: boolean;
/**
* @computed
* Whether the progress bar is in RTL mode.
*/
isRtl: boolean;
}>;
type UserDefinedContext = RequiredBy;
type MachineContext = PublicContext & PrivateContext & ComputedContext;
type MachineState = {
value: "idle";
};
type State = StateMachine.State;
type Send = StateMachine.Send;
type Service = Machine;
interface ViewProps {
state: ProgressState;
}
interface MachineApi {
/**
* The current value of the progress bar.
*/
value: number | null;
/**
* The current value of the progress bar as a string.
*/
valueAsString: string;
/**
* Sets the current value of the progress bar.
*/
setValue(value: number | null): void;
/**
* Sets the current value of the progress bar to the max value.
*/
setToMax(): void;
/**
* Sets the current value of the progress bar to the min value.
*/
setToMin(): void;
/**
* The percentage of the progress bar's value.
*/
percent: number;
/**
* The percentage of the progress bar's value as a string.
*/
percentAsString: string;
/**
* The minimum allowed value of the progress bar.
*/
min: number;
/**
* The maximum allowed value of the progress bar.
*/
max: number;
/**
* Whether the progress bar is indeterminate.
*/
indeterminate: boolean;
getRootProps(): T["element"];
getLabelProps(): T["element"];
getTrackProps(): T["element"];
getValueTextProps(): T["element"];
getRangeProps(): T["element"];
getViewProps(props: ViewProps): T["element"];
getCircleProps(): T["svg"];
getCircleTrackProps(): T["circle"];
getCircleRangeProps(): T["circle"];
}
declare function connect(state: State, send: Send, normalize: NormalizeProps): MachineApi;
declare function machine(userContext: UserDefinedContext): _zag_js_core.Machine;
declare const props: ("dir" | "id" | "max" | "min" | "value" | "orientation" | "getRootNode" | "ids" | "translations")[];
declare const splitProps: >(props: Props) => [Partial, Omit];
export { type MachineApi as Api, type UserDefinedContext as Context, type ElementIds, type IntlTranslations, type ProgressState, type Service, type ValueTranslationDetails, type ViewProps, anatomy, connect, machine, props, splitProps };
© 2015 - 2025 Weber Informatics LLC | Privacy Policy