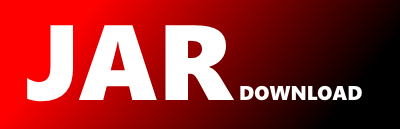
package.dist.index.d.mts Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rating-group Show documentation
Show all versions of rating-group Show documentation
Core logic for the rating-group widget implemented as a state machine
The newest version!
import * as _zag_js_anatomy from '@zag-js/anatomy';
import { RequiredBy, PropTypes, DirectionProperty, CommonProperties, NormalizeProps } from '@zag-js/types';
import * as _zag_js_core from '@zag-js/core';
import { Machine, StateMachine } from '@zag-js/core';
declare const anatomy: _zag_js_anatomy.AnatomyInstance<"root" | "label" | "item" | "control">;
interface ValueChangeDetails {
value: number;
}
interface HoverChangeDetails {
hoveredValue: number;
}
interface IntlTranslations {
ratingValueText(index: number): string;
}
type ElementIds = Partial<{
root: string;
label: string;
hiddenInput: string;
control: string;
item(id: string): string;
}>;
interface PublicContext extends DirectionProperty, CommonProperties {
/**
* The ids of the elements in the rating. Useful for composition.
*/
ids?: ElementIds | undefined;
/**
* Specifies the localized strings that identifies the accessibility elements and their states
*/
translations: IntlTranslations;
/**
* The total number of ratings.
* @default 5
*/
count: number;
/**
* The name attribute of the rating element (used in forms).
*/
name?: string | undefined;
/**
* The associate form of the underlying input element.
*/
form?: string | undefined;
/**
* The current rating value.
*/
value: number;
/**
* Whether the rating is readonly.
*/
readOnly?: boolean | undefined;
/**
* Whether the rating is disabled.
*/
disabled?: boolean | undefined;
/**
* Whether the rating is required.
*/
required?: boolean | undefined;
/**
* Whether to allow half stars.
*/
allowHalf?: boolean | undefined;
/**
* Whether to autofocus the rating.
*/
autoFocus?: boolean | undefined;
/**
* Function to be called when the rating value changes.
*/
onValueChange?: ((details: ValueChangeDetails) => void) | undefined;
/**
* Function to be called when the rating value is hovered.
*/
onHoverChange?: ((details: HoverChangeDetails) => void) | undefined;
}
type UserDefinedContext = RequiredBy;
type ComputedContext = Readonly<{
/**
* @computed
* Whether the rating is interactive
*/
isInteractive: boolean;
/**
* @computed
* Whether the pointer is hovering over the rating
*/
isHovering: boolean;
/**
* @computed
* Whether the rating is disabled
*/
isDisabled: boolean;
}>;
interface PrivateContext {
}
interface MachineContext extends PublicContext, ComputedContext, PrivateContext {
}
interface MachineState {
value: "idle" | "hover" | "focus";
}
type State = StateMachine.State;
type Send = StateMachine.Send;
type Service = Machine;
interface ItemProps {
index: number;
}
interface ItemState {
/**
* Whether the rating item is highlighted.
*/
highlighted: boolean;
/**
* Whether the rating item is partially highlighted.
*/
half: boolean;
/**
* Whether the rating item is checked.
*/
checked: boolean;
}
interface MachineApi {
/**
* Sets the value of the rating group
*/
setValue(value: number): void;
/**
* Clears the value of the rating group
*/
clearValue(): void;
/**
* Whether the rating group is being hovered
*/
hovering: boolean;
/**
* The current value of the rating group
*/
value: number;
/**
* The value of the currently hovered rating
*/
hoveredValue: number;
/**
* The total number of ratings
*/
count: number;
/**
* The array of rating values. Returns an array of numbers from 1 to the max value.
*/
items: number[];
/**
* Returns the state of a rating item
*/
getItemState(props: ItemProps): ItemState;
getRootProps(): T["element"];
getHiddenInputProps(): T["input"];
getLabelProps(): T["element"];
getControlProps(): T["element"];
getItemProps(props: ItemProps): T["element"];
}
declare function connect(state: State, send: Send, normalize: NormalizeProps): MachineApi;
declare function machine(userContext: UserDefinedContext): _zag_js_core.Machine;
declare const props: ("form" | "value" | "dir" | "id" | "getRootNode" | "name" | "disabled" | "autoFocus" | "required" | "ids" | "translations" | "count" | "readOnly" | "allowHalf" | "onValueChange" | "onHoverChange")[];
declare const splitProps: >(props: Props) => [Partial, Omit];
declare const itemProps: "index"[];
declare const splitItemProps: (props: Props) => [ItemProps, Omit];
export { type MachineApi as Api, type UserDefinedContext as Context, type HoverChangeDetails, type IntlTranslations, type ItemProps, type ItemState, type Service, type ValueChangeDetails, anatomy, connect, itemProps, machine, props, splitItemProps, splitProps };
© 2015 - 2025 Weber Informatics LLC | Privacy Policy