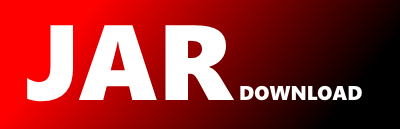
package.dist.index.mjs Maven / Gradle / Ivy
export { mergeProps } from '@zag-js/core';
import { createNormalizer } from '@zag-js/types';
import ReactExport, { Children, useRef, useSyncExternalStore, useCallback, useMemo, useEffect, useLayoutEffect } from 'react';
import { createPortal } from 'react-dom';
import { jsx, Fragment } from 'react/jsx-runtime';
import { makeGlobal, subscribe, snapshot } from '@zag-js/store';
import { isChanged, createProxy } from 'proxy-compare';
var normalizeProps = createNormalizer((v) => v);
var Portal = (props) => {
const { children, container, disabled, getRootNode } = props;
const isServer = typeof window === "undefined";
if (isServer || disabled) return /* @__PURE__ */ jsx(Fragment, { children });
const doc = getRootNode?.().ownerDocument ?? document;
const mountNode = container?.current ?? doc.body;
return /* @__PURE__ */ jsx(Fragment, { children: Children.map(children, (child) => createPortal(child, mountNode)) });
};
// ../../utilities/core/src/equal.ts
var isArrayLike = (value) => value?.constructor.name === "Array";
var isArrayEqual = (a, b) => {
if (a.length !== b.length) return false;
for (let i = 0; i < a.length; i++) {
if (!isEqual(a[i], b[i])) return false;
}
return true;
};
var isEqual = (a, b) => {
if (Object.is(a, b)) return true;
if (a == null && b != null || a != null && b == null) return false;
if (typeof a?.isEqual === "function" && typeof b?.isEqual === "function") {
return a.isEqual(b);
}
if (typeof a === "function" && typeof b === "function") {
return a.toString() === b.toString();
}
if (isArrayLike(a) && isArrayLike(b)) {
return isArrayEqual(Array.from(a), Array.from(b));
}
if (!(typeof a === "object") || !(typeof b === "object")) return false;
const keys = Object.keys(b ?? /* @__PURE__ */ Object.create(null));
const length = keys.length;
for (let i = 0; i < length; i++) {
const hasKey = Reflect.has(a, keys[i]);
if (!hasKey) return false;
}
for (let i = 0; i < length; i++) {
const key = keys[i];
if (!isEqual(a[key], b[key])) return false;
}
return true;
};
// ../../utilities/core/src/guard.ts
var isDev = () => process.env.NODE_ENV !== "production";
var fnToString = Function.prototype.toString;
fnToString.call(Object);
// ../../utilities/core/src/object.ts
function compact(obj) {
if (!isPlainObject(obj) || obj === void 0) {
return obj;
}
const keys = Reflect.ownKeys(obj).filter((key) => typeof key === "string");
const filtered = {};
for (const key of keys) {
const value = obj[key];
if (value !== void 0) {
filtered[key] = compact(value);
}
}
return filtered;
}
var isPlainObject = (value) => {
return value && typeof value === "object" && value.constructor === Object;
};
function useUpdateEffect(callback, deps) {
const render = useRef(false);
const effect = useRef(false);
useEffect(() => {
const mounted = render.current;
const run = mounted && effect.current;
if (run) {
return callback();
}
effect.current = true;
}, deps);
useEffect(() => {
render.current = true;
return () => {
render.current = false;
};
}, []);
}
// src/use-snapshot.ts
var { use } = ReactExport;
var targetCache = makeGlobal("__zag__targetCache", () => /* @__PURE__ */ new WeakMap());
function useSnapshot(service, options) {
const { actions, context, sync: notifyInSync } = options ?? {};
const lastSnapshot = useRef();
const lastAffected = useRef();
const currSnapshot = useSyncExternalStore(
useCallback((callback) => subscribe(service.state, callback, notifyInSync), [notifyInSync]),
() => {
const nextSnapshot = snapshot(service.state, use);
try {
if (lastSnapshot.current && lastAffected.current && !isChanged(lastSnapshot.current, nextSnapshot, lastAffected.current, /* @__PURE__ */ new WeakMap())) {
return lastSnapshot.current;
}
} catch {
}
return nextSnapshot;
},
() => snapshot(service.state, use)
);
service.setOptions({ actions });
const ctx = useMemo(() => compact(context ?? {}), [context]);
useUpdateEffect(() => {
const entries = Object.entries(ctx);
const previousCtx = service.contextSnapshot ?? {};
const equality = entries.map(([key, value]) => ({
key,
curr: value,
prev: previousCtx[key],
equal: isEqual(previousCtx[key], value)
}));
const allEqual = equality.every(({ equal }) => equal);
if (!allEqual) {
service.setContext(ctx);
}
}, [ctx]);
const currAffected = /* @__PURE__ */ new WeakMap();
useEffect(() => {
lastSnapshot.current = currSnapshot;
lastAffected.current = currAffected;
});
const proxyCache = useMemo(() => /* @__PURE__ */ new WeakMap(), []);
return createProxy(currSnapshot, currAffected, proxyCache, targetCache);
}
// src/use-actor.ts
function useActor(service) {
const state = useSnapshot(service);
return [state, service.send];
}
function useConstant(fn) {
const ref = useRef();
if (!ref.current) ref.current = { v: fn() };
return ref.current.v;
}
var useSafeLayoutEffect = typeof document !== "undefined" ? useLayoutEffect : useEffect;
// src/use-service.ts
function useService(machine, options) {
const { state: hydratedState, context } = options ?? {};
const service = useConstant(() => {
const instance = typeof machine === "function" ? machine() : machine;
if (context) instance.setContext(context);
instance._created();
return instance;
});
const snapshotRef = useRef();
useSafeLayoutEffect(() => {
const stateInit = hydratedState ?? snapshotRef.current;
service.start(stateInit);
return () => {
if (isDev()) {
snapshotRef.current = service.getHydrationState();
}
service.stop();
};
}, []);
return service;
}
// src/use-machine.ts
function useMachine(machine, options) {
const service = useService(machine, options);
const state = useSnapshot(service, options);
return [state, service.send, service];
}
export { Portal, normalizeProps, useActor, useMachine };
© 2015 - 2025 Weber Informatics LLC | Privacy Policy