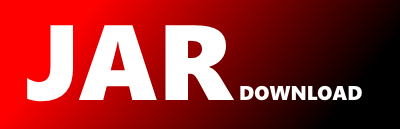
package.dist.index.d.mts Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of signature-pad Show documentation
Show all versions of signature-pad Show documentation
Core logic for the signature-pad widget implemented as a state machine
The newest version!
import * as _zag_js_anatomy from '@zag-js/anatomy';
import { RequiredBy, PropTypes, DirectionProperty, CommonProperties, NormalizeProps } from '@zag-js/types';
import * as _zag_js_core from '@zag-js/core';
import { Machine, StateMachine } from '@zag-js/core';
import { StrokeOptions } from 'perfect-freehand';
export { StrokeOptions } from 'perfect-freehand';
declare const anatomy: _zag_js_anatomy.AnatomyInstance<"root" | "control" | "segment" | "segmentPath" | "guide" | "clearTrigger" | "label">;
interface Point {
x: number;
y: number;
pressure: number;
}
interface DrawDetails {
paths: string[];
}
interface DrawingOptions extends StrokeOptions {
/**
* The color of the stroke.
* Note: Must be a valid CSS color string, not a css variable.
*/
fill?: string | undefined;
}
type DataUrlType = "image/png" | "image/jpeg" | "image/svg+xml";
interface DrawEndDetails {
paths: string[];
getDataUrl(type: DataUrlType, quality?: number): Promise;
}
type ElementIds = Partial<{
root: string;
control: string;
hiddenInput: string;
label: string;
}>;
interface IntlTranslations {
clearTrigger: string;
control: string;
}
interface PublicContext extends DirectionProperty, CommonProperties {
/**
* The ids of the signature pad elements. Useful for composition.
*/
ids?: ElementIds | undefined;
/**
* The translations of the signature pad. Useful for internationalization.
*/
translations?: IntlTranslations | undefined;
/**
* Callback when the signature pad is drawing.
*/
onDraw?(details: DrawDetails): void;
/**
* Callback when the signature pad is done drawing.
*/
onDrawEnd?(details: DrawEndDetails): void;
/**
* The drawing options.
* @default '{ size: 2, simulatePressure: true }'
*/
drawing: DrawingOptions;
/**
* Whether the signature pad is disabled.
*/
disabled?: boolean | undefined;
/**
* Whether the signature pad is required.
*/
required?: boolean | undefined;
/**
* Whether the signature pad is read-only.
*/
readOnly?: boolean | undefined;
/**
* The name of the signature pad. Useful for form submission.
*/
name?: string | undefined;
}
interface PrivateContext {
/**
* The layers of the signature pad. A layer is a snapshot of a single stroke interaction.
*/
paths: string[];
/**
* The current layer points.
*/
currentPoints: Point[];
/**
* The current stroke path
*/
currentPath: string | null;
}
type ComputedContext = Readonly<{
isInteractive: boolean;
isEmpty: boolean;
}>;
type UserDefinedContext = RequiredBy;
interface MachineContext extends PublicContext, PrivateContext, ComputedContext {
}
interface MachineState {
value: "idle" | "drawing";
}
type State = StateMachine.State;
type Send = StateMachine.Send;
type Service = Machine;
interface SegmentPathProps {
path: string;
}
interface HiddenInputProps {
value: string;
}
interface MachineApi {
/**
* Whether the signature pad is empty.
*/
empty: boolean;
/**
* Whether the user is currently drawing.
*/
drawing: boolean;
/**
* The current path being drawn.
*/
currentPath: string | null;
/**
* The paths of the signature pad.
*/
paths: string[];
/**
* Returns the data URL of the signature pad.
*/
getDataUrl(type: DataUrlType, quality?: number): Promise;
/**
* Clears the signature pad.
*/
clear(): void;
getLabelProps(): T["element"];
getRootProps(): T["element"];
getControlProps(): T["element"];
getSegmentProps(): T["svg"];
getSegmentPathProps(props: SegmentPathProps): T["path"];
getHiddenInputProps(props: HiddenInputProps): T["input"];
getGuideProps(): T["element"];
getClearTriggerProps(): T["element"];
}
declare function connect(state: State, send: Send, normalize: NormalizeProps): MachineApi;
declare function machine(userContext: UserDefinedContext): _zag_js_core.Machine;
declare const props: ("dir" | "id" | "getRootNode" | "name" | "disabled" | "required" | "ids" | "translations" | "onDraw" | "onDrawEnd" | "drawing" | "readOnly")[];
declare const splitProps: >(props: Props) => [Partial, Omit];
export { type MachineApi as Api, type UserDefinedContext as Context, type DataUrlType, type DrawDetails, type DrawEndDetails, type DrawingOptions, type ElementIds, type HiddenInputProps, type IntlTranslations, type SegmentPathProps, type Service, anatomy, connect, machine, props, splitProps };
© 2015 - 2025 Weber Informatics LLC | Privacy Policy