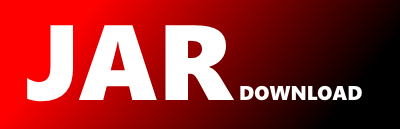
package.dist.index.d.ts Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of splitter Show documentation
Show all versions of splitter Show documentation
Core logic for the splitter widget implemented as a state machine
The newest version!
import * as _zag_js_anatomy from '@zag-js/anatomy';
import { RequiredBy, PropTypes, DirectionProperty, CommonProperties, Required, NormalizeProps } from '@zag-js/types';
import * as _zag_js_core from '@zag-js/core';
import { Machine, StateMachine } from '@zag-js/core';
declare const anatomy: _zag_js_anatomy.AnatomyInstance<"root" | "panel" | "resizeTrigger">;
type PanelId = string | number;
interface PanelSizeData {
id: PanelId;
size?: number | undefined;
minSize?: number | undefined;
maxSize?: number | undefined;
}
interface SizeChangeDetails {
size: PanelSizeData[];
activeHandleId: string | null;
}
type ElementIds = Partial<{
root: string;
resizeTrigger(id: string): string;
label(id: string): string;
panel(id: string | number): string;
}>;
interface PublicContext extends DirectionProperty, CommonProperties {
/**
* The orientation of the splitter. Can be `horizontal` or `vertical`
*/
orientation: "horizontal" | "vertical";
/**
* The size data of the panels
*/
size: PanelSizeData[];
/**
* Function called when the splitter is resized.
*/
onSizeChange?: ((details: SizeChangeDetails) => void) | undefined;
/**
* Function called when the splitter resize ends.
*/
onSizeChangeEnd?: ((details: SizeChangeDetails) => void) | undefined;
/**
* The ids of the elements in the splitter. Useful for composition.
*/
ids?: ElementIds | undefined;
}
type UserDefinedContext = RequiredBy;
type NormalizedPanelData = Array & {
remainingSize: number;
minSize: number;
maxSize: number;
start: number;
end: number;
}>;
type ComputedContext = Readonly<{
isHorizontal: boolean;
panels: NormalizedPanelData;
activeResizeBounds?: {
min: number;
max: number;
} | undefined;
activeResizePanels?: {
before: PanelSizeData;
after: PanelSizeData;
} | undefined;
}>;
interface PrivateContext {
activeResizeId: string | null;
previousPanels: NormalizedPanelData;
activeResizeState: {
isAtMin: boolean;
isAtMax: boolean;
};
initialSize: Array>>;
}
interface MachineContext extends PublicContext, ComputedContext, PrivateContext {
}
interface MachineState {
value: "idle" | "hover:temp" | "hover" | "dragging" | "focused";
tags: "focus";
}
type State = StateMachine.State;
type Send = StateMachine.Send;
type Service = Machine;
interface PanelProps {
id: PanelId;
snapSize?: number | undefined;
}
interface ResizeTriggerProps {
id: `${PanelId}:${PanelId}`;
step?: number | undefined;
disabled?: boolean | undefined;
}
interface ResizeTriggerState {
disabled: boolean;
focused: boolean;
panelIds: string[];
min: number | undefined;
max: number | undefined;
value: number;
}
interface PanelBounds {
min: number;
max: number;
}
interface MachineApi {
/**
* Whether the splitter is focused.
*/
focused: boolean;
/**
* Whether the splitter is being dragged.
*/
dragging: boolean;
/**
* The bounds of the currently dragged splitter handle.
*/
bounds: PanelBounds | undefined;
/**
* Function to set a panel to its minimum size.
*/
setToMinSize(id: PanelId): void;
/**
* Function to set a panel to its maximum size.
*/
setToMaxSize(id: PanelId): void;
/**
* Function to set the size of a panel.
*/
setSize(id: PanelId, size: number): void;
/**
* Returns the state details for a resize trigger.
*/
getResizeTriggerState(props: ResizeTriggerProps): ResizeTriggerState;
getRootProps(): T["element"];
getPanelProps(props: PanelProps): T["element"];
getResizeTriggerProps(props: ResizeTriggerProps): T["element"];
}
declare function connect(state: State, send: Send, normalize: NormalizeProps): MachineApi;
declare function machine(userContext: UserDefinedContext): _zag_js_core.Machine;
declare const props: ("dir" | "id" | "getRootNode" | "size" | "orientation" | "onSizeChange" | "onSizeChangeEnd" | "ids")[];
declare const splitProps: >(props: Props) => [Partial, Omit];
declare const panelProps: (keyof PanelProps)[];
declare const splitPanelProps: (props: Props) => [PanelProps, Omit];
declare const resizeTriggerProps: (keyof ResizeTriggerProps)[];
declare const splitResizeTriggerProps: (props: Props) => [ResizeTriggerProps, Omit];
export { type MachineApi as Api, type UserDefinedContext as Context, type MachineState, type PanelProps, type PanelSizeData, type ResizeTriggerProps, type Service, type SizeChangeDetails, anatomy, connect, machine, panelProps, props, resizeTriggerProps, splitPanelProps, splitProps, splitResizeTriggerProps };
© 2015 - 2025 Weber Informatics LLC | Privacy Policy