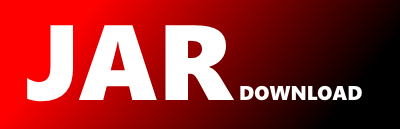
package.dist.index.d.mts Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of steps Show documentation
Show all versions of steps Show documentation
Core logic for the steps widget implemented as a state machine
The newest version!
import * as _zag_js_anatomy from '@zag-js/anatomy';
import { RequiredBy, PropTypes, DirectionProperty, CommonProperties, NormalizeProps } from '@zag-js/types';
import * as _zag_js_core from '@zag-js/core';
import { Machine, StateMachine } from '@zag-js/core';
declare const anatomy: _zag_js_anatomy.AnatomyInstance<"root" | "list" | "item" | "trigger" | "indicator" | "separator" | "content" | "nextTrigger" | "prevTrigger" | "progress">;
interface StepChangeDetails {
step: number;
}
interface ElementIds {
root?: string | undefined;
list?: string | undefined;
triggerId?(index: number): string;
contentId?(index: number): string;
}
interface PublicContext extends DirectionProperty, CommonProperties {
/**
* The custom ids for the stepper elements
*/
ids?: ElementIds | undefined;
/**
* The current value of the stepper
*/
step: number;
/**
* Callback to be called when the value changes
*/
onStepChange?(details: StepChangeDetails): void;
/**
* Callback to be called when a step is completed
*/
onStepComplete?: VoidFunction | undefined;
/**
* If `true`, the stepper requires the user to complete the steps in order
*/
linear?: boolean | undefined;
/**
* The orientation of the stepper
*/
orientation?: "horizontal" | "vertical" | undefined;
/**
* The total number of steps
*/
count: number;
}
interface PrivateContext {
}
type ComputedContext = Readonly<{
percent: number;
hasNextStep: boolean;
hasPrevStep: boolean;
}>;
type UserDefinedContext = RequiredBy;
interface MachineContext extends PublicContext, PrivateContext, ComputedContext {
}
interface MachineState {
value: "idle";
}
type State = StateMachine.State;
type Send = StateMachine.Send;
type Service = Machine;
interface ItemProps {
index: number;
}
interface ItemState {
index: number;
triggerId: string;
contentId: string;
current: boolean;
completed: boolean;
incomplete: boolean;
last: boolean;
first: boolean;
}
interface MachineApi {
/**
* The value of the stepper.
*/
value: number;
/**
* The percentage of the stepper.
*/
percent: number;
/**
* The total number of steps.
*/
count: number;
/**
* Whether the stepper has a next step.
*/
hasNextStep: boolean;
/**
* Whether the stepper has a previous step.
*/
hasPrevStep: boolean;
/**
* Function to set the value of the stepper.
*/
setStep(step: number): void;
/**
* Function to go to the next step.
*/
goToNextStep(): void;
/**
* Function to go to the previous step.
*/
goToPrevStep(): void;
/**
* Function to go to reset the stepper.
*/
resetStep(): void;
/**
* Returns the state of the item at the given index.
*/
getItemState(props: ItemProps): ItemState;
getRootProps(): T["element"];
getListProps(): T["element"];
getItemProps(props: ItemProps): T["element"];
getTriggerProps(props: ItemProps): T["element"];
getContentProps(props: ItemProps): T["element"];
getNextTriggerProps(): T["button"];
getPrevTriggerProps(): T["button"];
getProgressProps(): T["element"];
getIndicatorProps(props: ItemProps): T["element"];
getSeparatorProps(props: ItemProps): T["element"];
}
declare function connect(state: State, send: Send, normalize: NormalizeProps): MachineApi;
declare function machine(userContext: UserDefinedContext): _zag_js_core.Machine;
declare const props: ("dir" | "id" | "getRootNode" | "linear" | "step" | "orientation" | "ids" | "onStepChange" | "onStepComplete" | "count")[];
declare const splitProps: >(props: Props) => [Partial, Omit];
export { type MachineApi as Api, type UserDefinedContext as Context, type ElementIds, type ItemProps, type ItemState, type Service, type StepChangeDetails, anatomy, connect, machine, props, splitProps };
© 2015 - 2025 Weber Informatics LLC | Privacy Policy