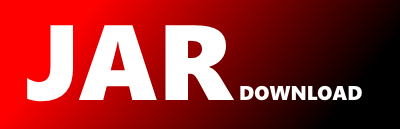
package.dist.index.d.mts Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of toast Show documentation
Show all versions of toast Show documentation
Core logic for the toast widget implemented as a state machine
The newest version!
import { CommonProperties, Direction, RequiredBy, PropTypes, DirectionProperty, NormalizeProps } from '@zag-js/types';
import * as _zag_js_core from '@zag-js/core';
import { Machine, StateMachine } from '@zag-js/core';
import * as _zag_js_anatomy from '@zag-js/anatomy';
type Type = "success" | "error" | "loading" | "info" | (string & {});
type Placement = "top-start" | "top" | "top-end" | "bottom-start" | "bottom" | "bottom-end";
type Status = "visible" | "dismissing" | "unmounted";
interface GenericOptions {
/**
* The title of the toast.
*/
title?: T | undefined;
/**
* The description of the toast.
*/
description?: T | undefined;
}
interface StatusChangeDetails {
status: Status;
}
interface ActionOptions {
/**
* The label of the action
*/
label: string;
/**
* The function to call when the action is clicked
*/
onClick: () => void;
}
interface Options extends GenericOptions {
/**
* The duration the toast will be visible
*/
duration?: number | undefined;
/**
* The duration for the toast to kept alive before it is removed.
* Useful for exit transitions.
*/
removeDelay?: number | undefined;
/**
* The placement of the toast
*/
placement?: Placement | undefined;
/**
* The unique id of the toast
*/
id?: string | undefined;
/**
* The type of the toast
*/
type?: Type | undefined;
/**
* Function called when the toast is visible
*/
onStatusChange?(details: StatusChangeDetails): void;
/**
* The action of the toast
*/
action?: ActionOptions | undefined;
/**
* The metadata of the toast
*/
meta?: Record | undefined;
}
interface MachineContext extends Omit, MachinePrivateContext, Omit, "removeDelay"> {
/**
* The duration for the toast to kept alive before it is removed.
* Useful for exit transitions.
*/
removeDelay: number;
/**
* The document's text/writing direction.
*/
dir?: Direction | undefined;
/**
* The time the toast was created
*/
createdAt: number;
/**
* The time left before the toast is removed
*/
remaining: number;
}
interface MachinePrivateContext {
}
interface MachineState {
value: "visible" | "visible:updating" | "dismissing" | "unmounted" | "visible:persist";
tags: "visible" | "paused" | "updating";
}
type State = StateMachine.State, MachineState>;
type Send = StateMachine.Send;
type Service = Machine, MachineState>;
interface GroupPublicContext extends DirectionProperty, CommonProperties {
/**
* Whether to pause toast when the user leaves the browser tab
* @default false
*/
pauseOnPageIdle: boolean;
/**
* The gap or spacing between toasts
* @default 16
*/
gap: number;
/**
* The maximum number of toasts that can be shown at once
* @default Number.MAX_SAFE_INTEGER
*/
max: number;
/**
* The offset from the safe environment edge of the viewport
* @default "1rem"
*/
offsets: string | Record<"left" | "right" | "bottom" | "top", string>;
/**
* The hotkey that will move focus to the toast group
* @default '["altKey", "KeyT"]'
*/
hotkey: string[];
/**
* Whether the toasts should overlap each other
*/
overlap?: boolean | undefined;
/**
* The placement of the toast
*/
placement: Placement;
/**
* The duration for the toast to kept alive before it is removed.
* Useful for exit transitions.
*
* @default 200
*/
removeDelay: number;
/**
* The duration the toast will be visible
*/
duration?: number | undefined;
}
interface UserDefinedGroupContext extends RequiredBy {
}
type GroupComputedContext = Readonly<{
/**
* @computed
* The total number of toasts in the group
*/
count: number;
}>;
interface GroupPrivateContext extends GenericOptions {
}
interface GroupMachineContext extends GroupPublicContext, GroupPrivateContext, GroupComputedContext {
}
interface GroupMachineState {
value: "stack" | "overlap";
}
type GroupState = StateMachine.State>;
type GroupSend = StateMachine.Send;
type GroupService = Machine, GroupMachineState>;
type MaybeFunction = Value | ((arg: Args) => Value);
interface PromiseOptions {
loading: Options;
success: MaybeFunction, V>;
error: MaybeFunction, Error>;
finally?: (() => void | Promise) | undefined;
}
interface GroupProps {
/**
* The placement of the toast region
*/
placement: Placement;
/**
* The human-readable label for the toast region
*/
label?: string | undefined;
}
interface GroupMachineApi {
/**
* The total number of toasts
*/
getCount(): number;
/**
* The placements of the active toasts
*/
getPlacements(): Placement[];
/**
* The active toasts by placement
*/
getToastsByPlacement(placement: Placement): Service[];
/**
* Returns whether the toast id is visible
*/
isVisible(id: string): boolean;
/**
* Function to create a toast.
*/
create(options: Options): string | undefined;
/**
* Function to create or update a toast.
*/
upsert(options: Options): string | undefined;
/**
* Function to update a toast's options by id.
*/
update(id: string, options: Options): void;
/**
* Function to create a success toast.
*/
success(options: Options): string | undefined;
/**
* Function to create an error toast.
*/
error(options: Options): string | undefined;
/**
* Function to create a loading toast.
*/
loading(options: Options): string | undefined;
/**
* Function to resume a toast by id.
*/
resume(id?: string | undefined): void;
/**
* Function to pause a toast by id.
*/
pause(id?: string | undefined): void;
/**
* Function to dismiss a toast by id.
* If no id is provided, all toasts will be dismissed.
*/
dismiss(id?: string | undefined): void;
/**
* Function to dismiss all toasts by placement.
*/
dismissByPlacement(placement: Placement): void;
/**
* Function to remove a toast by id.
* If no id is provided, all toasts will be removed.
*/
remove(id?: string | undefined): void;
/**
* Function to create a toast from a promise.
* - When the promise resolves, the toast will be updated with the success options.
* - When the promise rejects, the toast will be updated with the error options.
*/
promise(promise: Promise | (() => Promise), options: PromiseOptions, shared?: Partial>): string;
/**
* Function to subscribe to the toast group.
*/
subscribe(callback: (toasts: Options[]) => void): VoidFunction;
getGroupProps(options: GroupProps): T["element"];
}
interface MachineApi extends GenericOptions {
/**
* The type of the toast.
*/
type: Type;
/**
* The current placement of the toast.
*/
placement: Placement;
/**
* Whether the toast is visible.
*/
visible: boolean;
/**
* Whether the toast is paused.
*/
paused: boolean;
/**
* Function to pause the toast (keeping it visible).
*/
pause(): void;
/**
* Function to resume the toast dismissing.
*/
resume(): void;
/**
* Function to instantly dismiss the toast.
*/
dismiss(): void;
getRootProps(): T["element"];
getTitleProps(): T["element"];
getGhostBeforeProps(): T["element"];
getGhostAfterProps(): T["element"];
getDescriptionProps(): T["element"];
getCloseTriggerProps(): T["button"];
getActionTriggerProps(): T["button"];
}
declare function groupConnect(serviceOrState: GroupState | GroupService, send: GroupSend, normalize: NormalizeProps): GroupMachineApi;
declare function groupMachine(userContext: UserDefinedGroupContext): _zag_js_core.Machine, GroupMachineState, _zag_js_core.StateMachine.AnyEventObject>;
declare function createToastMachine(options: Options): _zag_js_core.Machine, MachineState, _zag_js_core.StateMachine.AnyEventObject>;
declare const anatomy: _zag_js_anatomy.AnatomyInstance<"title" | "root" | "group" | "description" | "actionTrigger" | "closeTrigger">;
declare function connect(state: State, send: Send, normalize: NormalizeProps): MachineApi;
declare const group: {
connect: typeof groupConnect;
machine: typeof groupMachine;
};
export { type ActionOptions, type MachineApi as Api, type GenericOptions, type GroupMachineApi as GroupApi, type UserDefinedGroupContext as GroupMachineContext, type GroupProps, type GroupService, type GroupState, type MachineContext, type Options, type Placement, type PromiseOptions, type Service, type Status, type StatusChangeDetails, type Type, anatomy, connect, createToastMachine as createMachine, group };
© 2015 - 2025 Weber Informatics LLC | Privacy Policy