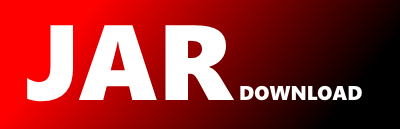
package.dist.index.d.mts Maven / Gradle / Ivy
The newest version!
declare function toArray(v: T | T[] | undefined | null): T[];
declare const fromLength: (length: number) => number[];
declare const first: (v: T[]) => T | undefined;
declare const last: (v: T[]) => T | undefined;
declare const isEmpty: (v: T[]) => boolean;
declare const has: (v: T[], t: any) => boolean;
declare const add: (v: T[], ...items: T[]) => T[];
declare const remove: (v: T[], ...items: T[]) => T[];
declare const removeAt: (v: T[], i: number) => T[];
declare const insertAt: (v: T[], i: number, ...items: T[]) => T[];
declare const uniq: (v: T[]) => T[];
declare const addOrRemove: (v: T[], item: T) => T[];
declare function clear(v: T[]): T[];
type IndexOptions = {
step?: number;
loop?: boolean;
};
declare function nextIndex(v: T[], idx: number, opts?: IndexOptions): number;
declare function next(v: T[], idx: number, opts?: IndexOptions): T | undefined;
declare function prevIndex(v: T[], idx: number, opts?: IndexOptions): number;
declare function prev(v: T[], index: number, opts?: IndexOptions): T | undefined;
declare const chunk: (v: T[], size: number) => T[][];
declare const isEqual: (a: any, b: any) => boolean;
type MaybeFunction = T | (() => T);
type Nullable = T | null | undefined;
declare const runIfFn: (v: T | undefined, ...a: T extends (...a: any[]) => void ? Parameters : never) => T extends (...a: any[]) => void ? NonNullable> : NonNullable;
declare const cast: (v: unknown) => T;
declare const noop: () => void;
declare const callAll: void>(...fns: (T | undefined)[]) => (...a: Parameters) => void;
declare const uuid: () => string;
declare function match(key: V, record: Record R)>, ...args: any[]): R;
declare const tryCatch: (fn: () => R, fallback: () => R) => R;
declare const isDev: () => boolean;
declare const isArray: (v: any) => v is any[];
declare const isBoolean: (v: any) => v is boolean;
declare const isObjectLike: (v: any) => v is Record;
declare const isObject: (v: any) => v is Record;
declare const isNumber: (v: any) => v is number;
declare const isString: (v: any) => v is string;
declare const isFunction: (v: any) => v is Function;
declare const isNull: (v: any) => v is null | undefined;
declare const hasProp: (obj: any, prop: T) => obj is Record;
declare const isPlainObject: (v: any) => boolean;
declare function compact | undefined>(obj: T): T;
declare function json(value: any): any;
declare function pick, K extends keyof T>(obj: T, keys: K[]): Pick;
declare function omit>(obj: T, keys: string[]): Omit;
type Dict = Record;
declare function splitProps(props: T, keys: (keyof T)[]): Dict[];
declare const createSplitProps: (keys: (keyof T)[]) => (props: Props) => [T, Omit];
declare function warn(m: string): void;
declare function warn(c: boolean, m: string): void;
declare function invariant(m: string): void;
declare function invariant(c: boolean, m: string): void;
export { type IndexOptions, type MaybeFunction, type Nullable, add, addOrRemove, callAll, cast, chunk, clear, compact, createSplitProps, first, fromLength, has, hasProp, insertAt, invariant, isArray, isBoolean, isDev, isEmpty, isEqual, isFunction, isNull, isNumber, isObject, isObjectLike, isPlainObject, isString, json, last, match, next, nextIndex, noop, omit, pick, prev, prevIndex, remove, removeAt, runIfFn, splitProps, toArray, tryCatch, uniq, uuid, warn };
© 2015 - 2025 Weber Informatics LLC | Privacy Policy