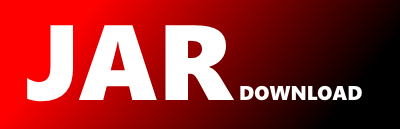
package.doc.callback.md Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nan Show documentation
Show all versions of nan Show documentation
Native Abstractions for Node.js: C++ header for Node 0.8 -> 18 compatibility
## Nan::Callback
`Nan::Callback` makes it easier to use `v8::Function` handles as callbacks. A class that wraps a `v8::Function` handle, protecting it from garbage collection and making it particularly useful for storage and use across asynchronous execution.
- Nan::Callback
### Nan::Callback
```c++
class Callback {
public:
Callback();
explicit Callback(const v8::Local &fn);
~Callback();
bool operator==(const Callback &other) const;
bool operator!=(const Callback &other) const;
v8::Local operator*() const;
MaybeLocal operator()(AsyncResource* async_resource,
v8::Local target,
int argc = 0,
v8::Local argv[] = 0) const;
MaybeLocal operator()(AsyncResource* async_resource,
int argc = 0,
v8::Local argv[] = 0) const;
void SetFunction(const v8::Local &fn);
v8::Local GetFunction() const;
bool IsEmpty() const;
void Reset(const v8::Local &fn);
void Reset();
MaybeLocal Call(v8::Local target,
int argc,
v8::Local argv[],
AsyncResource* async_resource) const;
MaybeLocal Call(int argc,
v8::Local argv[],
AsyncResource* async_resource) const;
// Deprecated versions. Use the versions that accept an async_resource instead
// as they run the callback in the correct async context as specified by the
// resource. If you want to call a synchronous JS function (i.e. on a
// non-empty JS stack), you can use Nan::Call instead.
v8::Local operator()(v8::Local target,
int argc = 0,
v8::Local argv[] = 0) const;
v8::Local operator()(int argc = 0,
v8::Local argv[] = 0) const;
v8::Local Call(v8::Local target,
int argc,
v8::Local argv[]) const;
v8::Local Call(int argc, v8::Local argv[]) const;
};
```
Example usage:
```c++
v8::Local function;
Nan::Callback callback(function);
callback.Call(0, 0);
```
© 2015 - 2024 Weber Informatics LLC | Privacy Policy