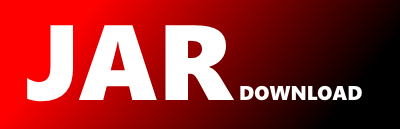
package.nan_callbacks_12_inl.h Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nan Show documentation
Show all versions of nan Show documentation
Native Abstractions for Node.js: C++ header for Node 0.8 -> 18 compatibility
/*********************************************************************
* NAN - Native Abstractions for Node.js
*
* Copyright (c) 2018 NAN contributors
*
* MIT License
********************************************************************/
#ifndef NAN_CALLBACKS_12_INL_H_
#define NAN_CALLBACKS_12_INL_H_
template
class ReturnValue {
v8::ReturnValue value_;
public:
template
explicit inline ReturnValue(const v8::ReturnValue &value) :
value_(value) {}
template
explicit inline ReturnValue(const ReturnValue& that)
: value_(that.value_) {
TYPE_CHECK(T, S);
}
// Handle setters
template inline void Set(const v8::Local &handle) {
TYPE_CHECK(T, S);
value_.Set(handle);
}
template inline void Set(const Global &handle) {
TYPE_CHECK(T, S);
#if defined(V8_MAJOR_VERSION) && (V8_MAJOR_VERSION > 4 || \
(V8_MAJOR_VERSION == 4 && defined(V8_MINOR_VERSION) && \
(V8_MINOR_VERSION > 5 || (V8_MINOR_VERSION == 5 && \
defined(V8_BUILD_NUMBER) && V8_BUILD_NUMBER >= 8))))
value_.Set(handle);
#else
value_.Set(*reinterpret_cast*>(&handle));
const_cast &>(handle).Reset();
#endif
}
// Fast primitive setters
inline void Set(bool value) {
TYPE_CHECK(T, v8::Boolean);
value_.Set(value);
}
inline void Set(double i) {
TYPE_CHECK(T, v8::Number);
value_.Set(i);
}
inline void Set(int32_t i) {
TYPE_CHECK(T, v8::Integer);
value_.Set(i);
}
inline void Set(uint32_t i) {
TYPE_CHECK(T, v8::Integer);
value_.Set(i);
}
// Fast JS primitive setters
inline void SetNull() {
TYPE_CHECK(T, v8::Primitive);
value_.SetNull();
}
inline void SetUndefined() {
TYPE_CHECK(T, v8::Primitive);
value_.SetUndefined();
}
inline void SetEmptyString() {
TYPE_CHECK(T, v8::String);
value_.SetEmptyString();
}
// Convenience getter for isolate
inline v8::Isolate *GetIsolate() const {
return value_.GetIsolate();
}
// Pointer setter: Uncompilable to prevent inadvertent misuse.
template
inline void Set(S *whatever) { TYPE_CHECK(S*, v8::Primitive); }
};
template
class FunctionCallbackInfo {
const v8::FunctionCallbackInfo &info_;
const v8::Local data_;
public:
explicit inline FunctionCallbackInfo(
const v8::FunctionCallbackInfo &info
, v8::Local data) :
info_(info)
, data_(data) {}
inline ReturnValue GetReturnValue() const {
return ReturnValue(info_.GetReturnValue());
}
#if NODE_MAJOR_VERSION < 10
inline v8::Local Callee() const { return info_.Callee(); }
#endif
inline v8::Local Data() const { return data_; }
inline v8::Local Holder() const { return info_.Holder(); }
inline bool IsConstructCall() const { return info_.IsConstructCall(); }
inline int Length() const { return info_.Length(); }
inline v8::Local operator[](int i) const { return info_[i]; }
inline v8::Local This() const { return info_.This(); }
inline v8::Isolate *GetIsolate() const { return info_.GetIsolate(); }
protected:
static const int kHolderIndex = 0;
static const int kIsolateIndex = 1;
static const int kReturnValueDefaultValueIndex = 2;
static const int kReturnValueIndex = 3;
static const int kDataIndex = 4;
static const int kCalleeIndex = 5;
static const int kContextSaveIndex = 6;
static const int kArgsLength = 7;
private:
NAN_DISALLOW_ASSIGN_COPY_MOVE(FunctionCallbackInfo)
};
template
class PropertyCallbackInfo {
const v8::PropertyCallbackInfo &info_;
const v8::Local data_;
public:
explicit inline PropertyCallbackInfo(
const v8::PropertyCallbackInfo &info
, const v8::Local data) :
info_(info)
, data_(data) {}
inline v8::Isolate* GetIsolate() const { return info_.GetIsolate(); }
inline v8::Local Data() const { return data_; }
inline v8::Local This() const { return info_.This(); }
inline v8::Local Holder() const { return info_.Holder(); }
inline ReturnValue GetReturnValue() const {
return ReturnValue(info_.GetReturnValue());
}
protected:
static const int kHolderIndex = 0;
static const int kIsolateIndex = 1;
static const int kReturnValueDefaultValueIndex = 2;
static const int kReturnValueIndex = 3;
static const int kDataIndex = 4;
static const int kThisIndex = 5;
static const int kArgsLength = 6;
private:
NAN_DISALLOW_ASSIGN_COPY_MOVE(PropertyCallbackInfo)
};
namespace imp {
static
void FunctionCallbackWrapper(const v8::FunctionCallbackInfo &info) {
v8::Local obj = info.Data().As();
FunctionCallback callback = reinterpret_cast(
reinterpret_cast(
obj->GetInternalField(kFunctionIndex).As()->Value()));
FunctionCallbackInfo
cbinfo(info, obj->GetInternalField(kDataIndex));
callback(cbinfo);
}
typedef void (*NativeFunction)(const v8::FunctionCallbackInfo &);
#if NODE_MODULE_VERSION > NODE_0_12_MODULE_VERSION
static
void GetterCallbackWrapper(
v8::Local property
, const v8::PropertyCallbackInfo &info) {
v8::Local obj = info.Data().As();
PropertyCallbackInfo
cbinfo(info, obj->GetInternalField(kDataIndex));
GetterCallback callback = reinterpret_cast(
reinterpret_cast(
obj->GetInternalField(kGetterIndex).As()->Value()));
callback(property.As(), cbinfo);
}
typedef void (*NativeGetter)
(v8::Local, const v8::PropertyCallbackInfo &);
static
void SetterCallbackWrapper(
v8::Local property
, v8::Local value
, const v8::PropertyCallbackInfo &info) {
v8::Local obj = info.Data().As();
PropertyCallbackInfo
cbinfo(info, obj->GetInternalField(kDataIndex));
SetterCallback callback = reinterpret_cast(
reinterpret_cast(
obj->GetInternalField(kSetterIndex).As()->Value()));
callback(property.As(), value, cbinfo);
}
typedef void (*NativeSetter)(
v8::Local
, v8::Local
, const v8::PropertyCallbackInfo &);
#else
static
void GetterCallbackWrapper(
v8::Local property
, const v8::PropertyCallbackInfo &info) {
v8::Local obj = info.Data().As();
PropertyCallbackInfo
cbinfo(info, obj->GetInternalField(kDataIndex));
GetterCallback callback = reinterpret_cast(
reinterpret_cast(
obj->GetInternalField(kGetterIndex).As()->Value()));
callback(property, cbinfo);
}
typedef void (*NativeGetter)
(v8::Local, const v8::PropertyCallbackInfo &);
static
void SetterCallbackWrapper(
v8::Local property
, v8::Local value
, const v8::PropertyCallbackInfo &info) {
v8::Local obj = info.Data().As();
PropertyCallbackInfo
cbinfo(info, obj->GetInternalField(kDataIndex));
SetterCallback callback = reinterpret_cast(
reinterpret_cast(
obj->GetInternalField(kSetterIndex).As()->Value()));
callback(property, value, cbinfo);
}
typedef void (*NativeSetter)(
v8::Local
, v8::Local
, const v8::PropertyCallbackInfo &);
#endif
#if NODE_MODULE_VERSION > NODE_0_12_MODULE_VERSION
static
void PropertyGetterCallbackWrapper(
v8::Local property
, const v8::PropertyCallbackInfo &info) {
v8::Local obj = info.Data().As();
PropertyCallbackInfo
cbinfo(info, obj->GetInternalField(kDataIndex));
PropertyGetterCallback callback = reinterpret_cast(
reinterpret_cast(
obj->GetInternalField(kPropertyGetterIndex)
.As()->Value()));
callback(property.As(), cbinfo);
}
typedef void (*NativePropertyGetter)
(v8::Local, const v8::PropertyCallbackInfo &);
static
void PropertySetterCallbackWrapper(
v8::Local property
, v8::Local value
, const v8::PropertyCallbackInfo &info) {
v8::Local obj = info.Data().As();
PropertyCallbackInfo
cbinfo(info, obj->GetInternalField(kDataIndex));
PropertySetterCallback callback = reinterpret_cast(
reinterpret_cast(
obj->GetInternalField(kPropertySetterIndex)
.As()->Value()));
callback(property.As(), value, cbinfo);
}
typedef void (*NativePropertySetter)(
v8::Local
, v8::Local
, const v8::PropertyCallbackInfo &);
static
void PropertyEnumeratorCallbackWrapper(
const v8::PropertyCallbackInfo &info) {
v8::Local obj = info.Data().As();
PropertyCallbackInfo
cbinfo(info, obj->GetInternalField(kDataIndex));
PropertyEnumeratorCallback callback =
reinterpret_cast(reinterpret_cast(
obj->GetInternalField(kPropertyEnumeratorIndex)
.As()->Value()));
callback(cbinfo);
}
typedef void (*NativePropertyEnumerator)
(const v8::PropertyCallbackInfo &);
static
void PropertyDeleterCallbackWrapper(
v8::Local property
, const v8::PropertyCallbackInfo &info) {
v8::Local obj = info.Data().As();
PropertyCallbackInfo
cbinfo(info, obj->GetInternalField(kDataIndex));
PropertyDeleterCallback callback = reinterpret_cast(
reinterpret_cast(
obj->GetInternalField(kPropertyDeleterIndex)
.As()->Value()));
callback(property.As(), cbinfo);
}
typedef void (NativePropertyDeleter)
(v8::Local, const v8::PropertyCallbackInfo &);
static
void PropertyQueryCallbackWrapper(
v8::Local property
, const v8::PropertyCallbackInfo &info) {
v8::Local obj = info.Data().As();
PropertyCallbackInfo
cbinfo(info, obj->GetInternalField(kDataIndex));
PropertyQueryCallback callback = reinterpret_cast(
reinterpret_cast(
obj->GetInternalField(kPropertyQueryIndex)
.As()->Value()));
callback(property.As(), cbinfo);
}
typedef void (*NativePropertyQuery)
(v8::Local, const v8::PropertyCallbackInfo &);
#else
static
void PropertyGetterCallbackWrapper(
v8::Local property
, const v8::PropertyCallbackInfo &info) {
v8::Local obj = info.Data().As();
PropertyCallbackInfo
cbinfo(info, obj->GetInternalField(kDataIndex));
PropertyGetterCallback callback = reinterpret_cast(
reinterpret_cast(
obj->GetInternalField(kPropertyGetterIndex)
.As()->Value()));
callback(property, cbinfo);
}
typedef void (*NativePropertyGetter)
(v8::Local, const v8::PropertyCallbackInfo &);
static
void PropertySetterCallbackWrapper(
v8::Local property
, v8::Local value
, const v8::PropertyCallbackInfo &info) {
v8::Local obj = info.Data().As();
PropertyCallbackInfo
cbinfo(info, obj->GetInternalField(kDataIndex));
PropertySetterCallback callback = reinterpret_cast(
reinterpret_cast(
obj->GetInternalField(kPropertySetterIndex)
.As()->Value()));
callback(property, value, cbinfo);
}
typedef void (*NativePropertySetter)(
v8::Local
, v8::Local
, const v8::PropertyCallbackInfo &);
static
void PropertyEnumeratorCallbackWrapper(
const v8::PropertyCallbackInfo &info) {
v8::Local obj = info.Data().As();
PropertyCallbackInfo
cbinfo(info, obj->GetInternalField(kDataIndex));
PropertyEnumeratorCallback callback =
reinterpret_cast(reinterpret_cast(
obj->GetInternalField(kPropertyEnumeratorIndex)
.As()->Value()));
callback(cbinfo);
}
typedef void (*NativePropertyEnumerator)
(const v8::PropertyCallbackInfo &);
static
void PropertyDeleterCallbackWrapper(
v8::Local property
, const v8::PropertyCallbackInfo &info) {
v8::Local obj = info.Data().As();
PropertyCallbackInfo
cbinfo(info, obj->GetInternalField(kDataIndex));
PropertyDeleterCallback callback = reinterpret_cast(
reinterpret_cast(
obj->GetInternalField(kPropertyDeleterIndex)
.As()->Value()));
callback(property, cbinfo);
}
typedef void (NativePropertyDeleter)
(v8::Local, const v8::PropertyCallbackInfo &);
static
void PropertyQueryCallbackWrapper(
v8::Local property
, const v8::PropertyCallbackInfo &info) {
v8::Local obj = info.Data().As();
PropertyCallbackInfo
cbinfo(info, obj->GetInternalField(kDataIndex));
PropertyQueryCallback callback = reinterpret_cast(
reinterpret_cast(
obj->GetInternalField(kPropertyQueryIndex)
.As()->Value()));
callback(property, cbinfo);
}
typedef void (*NativePropertyQuery)
(v8::Local, const v8::PropertyCallbackInfo &);
#endif
static
void IndexGetterCallbackWrapper(
uint32_t index, const v8::PropertyCallbackInfo &info) {
v8::Local obj = info.Data().As();
PropertyCallbackInfo
cbinfo(info, obj->GetInternalField(kDataIndex));
IndexGetterCallback callback = reinterpret_cast(
reinterpret_cast(
obj->GetInternalField(kIndexPropertyGetterIndex)
.As()->Value()));
callback(index, cbinfo);
}
typedef void (*NativeIndexGetter)
(uint32_t, const v8::PropertyCallbackInfo &);
static
void IndexSetterCallbackWrapper(
uint32_t index
, v8::Local value
, const v8::PropertyCallbackInfo &info) {
v8::Local obj = info.Data().As();
PropertyCallbackInfo
cbinfo(info, obj->GetInternalField(kDataIndex));
IndexSetterCallback callback = reinterpret_cast(
reinterpret_cast(
obj->GetInternalField(kIndexPropertySetterIndex)
.As()->Value()));
callback(index, value, cbinfo);
}
typedef void (*NativeIndexSetter)(
uint32_t
, v8::Local
, const v8::PropertyCallbackInfo &);
static
void IndexEnumeratorCallbackWrapper(
const v8::PropertyCallbackInfo &info) {
v8::Local obj = info.Data().As();
PropertyCallbackInfo
cbinfo(info, obj->GetInternalField(kDataIndex));
IndexEnumeratorCallback callback = reinterpret_cast(
reinterpret_cast(
obj->GetInternalField(
kIndexPropertyEnumeratorIndex).As()->Value()));
callback(cbinfo);
}
typedef void (*NativeIndexEnumerator)
(const v8::PropertyCallbackInfo &);
static
void IndexDeleterCallbackWrapper(
uint32_t index, const v8::PropertyCallbackInfo &info) {
v8::Local obj = info.Data().As();
PropertyCallbackInfo
cbinfo(info, obj->GetInternalField(kDataIndex));
IndexDeleterCallback callback = reinterpret_cast(
reinterpret_cast(
obj->GetInternalField(kIndexPropertyDeleterIndex)
.As()->Value()));
callback(index, cbinfo);
}
typedef void (*NativeIndexDeleter)
(uint32_t, const v8::PropertyCallbackInfo &);
static
void IndexQueryCallbackWrapper(
uint32_t index, const v8::PropertyCallbackInfo &info) {
v8::Local obj = info.Data().As();
PropertyCallbackInfo
cbinfo(info, obj->GetInternalField(kDataIndex));
IndexQueryCallback callback = reinterpret_cast(
reinterpret_cast(
obj->GetInternalField(kIndexPropertyQueryIndex)
.As()->Value()));
callback(index, cbinfo);
}
typedef void (*NativeIndexQuery)
(uint32_t, const v8::PropertyCallbackInfo &);
} // end of namespace imp
#endif // NAN_CALLBACKS_12_INL_H_
© 2015 - 2024 Weber Informatics LLC | Privacy Policy