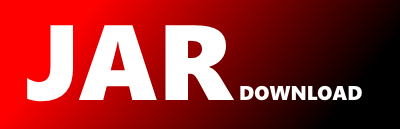
package.nan_implementation_pre_12_inl.h Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nan Show documentation
Show all versions of nan Show documentation
Native Abstractions for Node.js: C++ header for Node 0.8 -> 18 compatibility
/*********************************************************************
* NAN - Native Abstractions for Node.js
*
* Copyright (c) 2018 NAN contributors
*
* MIT License
********************************************************************/
#ifndef NAN_IMPLEMENTATION_PRE_12_INL_H_
#define NAN_IMPLEMENTATION_PRE_12_INL_H_
//==============================================================================
// node v0.10 implementation
//==============================================================================
namespace imp {
//=== Array ====================================================================
Factory::return_t
Factory::New() {
return v8::Array::New();
}
Factory::return_t
Factory::New(int length) {
return v8::Array::New(length);
}
//=== Boolean ==================================================================
Factory::return_t
Factory::New(bool value) {
return v8::Boolean::New(value)->ToBoolean();
}
//=== Boolean Object ===========================================================
Factory::return_t
Factory::New(bool value) {
return v8::BooleanObject::New(value).As();
}
//=== Context ==================================================================
Factory::return_t
Factory::New( v8::ExtensionConfiguration* extensions
, v8::Local tmpl
, v8::Local obj) {
v8::Persistent ctx = v8::Context::New(extensions, tmpl, obj);
v8::Local lctx = v8::Local::New(ctx);
ctx.Dispose();
return lctx;
}
//=== Date =====================================================================
Factory::return_t
Factory::New(double value) {
return v8::Date::New(value).As();
}
//=== External =================================================================
Factory::return_t
Factory::New(void * value) {
return v8::External::New(value);
}
//=== Function =================================================================
Factory::return_t
Factory::New( FunctionCallback callback
, v8::Local data) {
v8::HandleScope scope;
return scope.Close(Factory::New(
callback, data, v8::Local())
->GetFunction());
}
//=== FunctionTemplate =========================================================
Factory::return_t
Factory::New( FunctionCallback callback
, v8::Local data
, v8::Local signature) {
if (callback) {
v8::HandleScope scope;
v8::Local tpl = v8::ObjectTemplate::New();
tpl->SetInternalFieldCount(imp::kFunctionFieldCount);
v8::Local obj = tpl->NewInstance();
obj->SetInternalField(
imp::kFunctionIndex
, v8::External::New(reinterpret_cast(callback)));
v8::Local val = v8::Local::New(data);
if (!val.IsEmpty()) {
obj->SetInternalField(imp::kDataIndex, val);
}
// Note(agnat): Emulate length argument here. Unfortunately, I couldn't find
// a way. Have at it though...
return scope.Close(
v8::FunctionTemplate::New(imp::FunctionCallbackWrapper
, obj
, signature));
} else {
return v8::FunctionTemplate::New(0, data, signature);
}
}
//=== Number ===================================================================
Factory::return_t
Factory::New(double value) {
return v8::Number::New(value);
}
//=== Number Object ============================================================
Factory::return_t
Factory::New(double value) {
return v8::NumberObject::New(value).As();
}
//=== Integer, Int32 and Uint32 ================================================
template
typename IntegerFactory::return_t
IntegerFactory::New(int32_t value) {
return To(T::New(value));
}
template
typename IntegerFactory::return_t
IntegerFactory::New(uint32_t value) {
return To(T::NewFromUnsigned(value));
}
Factory::return_t
Factory::New(int32_t value) {
return To(v8::Uint32::NewFromUnsigned(value));
}
Factory::return_t
Factory::New(uint32_t value) {
return To(v8::Uint32::NewFromUnsigned(value));
}
//=== Object ===================================================================
Factory::return_t
Factory::New() {
return v8::Object::New();
}
//=== Object Template ==========================================================
Factory::return_t
Factory::New() {
return v8::ObjectTemplate::New();
}
//=== RegExp ===================================================================
Factory::return_t
Factory::New(
v8::Local pattern
, v8::RegExp::Flags flags) {
return v8::RegExp::New(pattern, flags);
}
//=== Script ===================================================================
Factory::return_t
Factory::New( v8::Local source) {
return v8::Script::New(source);
}
Factory::return_t
Factory::New( v8::Local source
, v8::ScriptOrigin const& origin) {
return v8::Script::New(source, const_cast(&origin));
}
//=== Signature ================================================================
Factory::return_t
Factory::New(Factory::FTH receiver) {
return v8::Signature::New(receiver);
}
//=== String ===================================================================
Factory::return_t
Factory::New() {
return v8::String::Empty();
}
Factory::return_t
Factory::New(const char * value, int length) {
return v8::String::New(value, length);
}
Factory::return_t
Factory::New(
std::string const& value) /* NOLINT(build/include_what_you_use) */ {
assert(value.size() <= INT_MAX && "string too long");
return v8::String::New(value.data(), static_cast(value.size()));
}
Factory::return_t
Factory::New(const uint16_t * value, int length) {
return v8::String::New(value, length);
}
Factory::return_t
Factory::New(v8::String::ExternalStringResource * value) {
return v8::String::NewExternal(value);
}
Factory::return_t
Factory::New(v8::String::ExternalAsciiStringResource * value) {
return v8::String::NewExternal(value);
}
//=== String Object ============================================================
Factory::return_t
Factory::New(v8::Local value) {
return v8::StringObject::New(value).As();
}
} // end of namespace imp
//=== Presistents and Handles ==================================================
template
inline v8::Local New(v8::Handle h) {
return v8::Local::New(h);
}
template
inline v8::Local New(v8::Persistent const& p) {
return v8::Local::New(p);
}
template
inline v8::Local New(Persistent const& p) {
return v8::Local::New(p.persistent);
}
template
inline v8::Local New(Global const& p) {
return v8::Local::New(p.persistent);
}
#endif // NAN_IMPLEMENTATION_PRE_12_INL_H_
© 2015 - 2024 Weber Informatics LLC | Privacy Policy