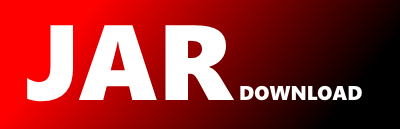
package.nan_new.h Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of nan Show documentation
Show all versions of nan Show documentation
Native Abstractions for Node.js: C++ header for Node 0.8 -> 18 compatibility
/*********************************************************************
* NAN - Native Abstractions for Node.js
*
* Copyright (c) 2018 NAN contributors
*
* MIT License
********************************************************************/
#ifndef NAN_NEW_H_
#define NAN_NEW_H_
namespace imp { // scnr
// TODO(agnat): Generalize
template v8::Local To(v8::Local i);
template <>
inline
v8::Local
To(v8::Local i) {
return Nan::To(i).ToLocalChecked();
}
template <>
inline
v8::Local
To(v8::Local i) {
return Nan::To(i).ToLocalChecked();
}
template <>
inline
v8::Local
To(v8::Local i) {
return Nan::To(i).ToLocalChecked();
}
template struct FactoryBase {
typedef v8::Local return_t;
};
template struct MaybeFactoryBase {
typedef MaybeLocal return_t;
};
template struct Factory;
template <>
struct Factory : FactoryBase {
static inline return_t New();
static inline return_t New(int length);
};
template <>
struct Factory : FactoryBase {
static inline return_t New(bool value);
};
template <>
struct Factory : FactoryBase {
static inline return_t New(bool value);
};
template <>
struct Factory : FactoryBase {
static inline
return_t
New( v8::ExtensionConfiguration* extensions = NULL
, v8::Local tmpl = v8::Local()
, v8::Local obj = v8::Local());
};
template <>
struct Factory : MaybeFactoryBase {
static inline return_t New(double value);
};
template <>
struct Factory : FactoryBase {
static inline return_t New(void *value);
};
template <>
struct Factory : FactoryBase {
static inline
return_t
New( FunctionCallback callback
, v8::Local data = v8::Local());
};
template <>
struct Factory : FactoryBase {
static inline
return_t
New( FunctionCallback callback = NULL
, v8::Local data = v8::Local()
, v8::Local signature = v8::Local());
};
template <>
struct Factory : FactoryBase {
static inline return_t New(double value);
};
template <>
struct Factory : FactoryBase {
static inline return_t New(double value);
};
template
struct IntegerFactory : FactoryBase {
typedef typename FactoryBase::return_t return_t;
static inline return_t New(int32_t value);
static inline return_t New(uint32_t value);
};
template <>
struct Factory : IntegerFactory {};
template <>
struct Factory : IntegerFactory {};
template <>
struct Factory : FactoryBase {
static inline return_t New(int32_t value);
static inline return_t New(uint32_t value);
};
template <>
struct Factory : FactoryBase {
static inline return_t New();
};
template <>
struct Factory : FactoryBase {
static inline return_t New();
};
template <>
struct Factory : MaybeFactoryBase {
static inline return_t New(
v8::Local pattern, v8::RegExp::Flags flags);
};
template <>
struct Factory : MaybeFactoryBase {
static inline return_t New( v8::Local source);
static inline return_t New( v8::Local source
, v8::ScriptOrigin const& origin);
};
template <>
struct Factory : FactoryBase {
typedef v8::Local FTH;
static inline return_t New(FTH receiver = FTH());
};
template <>
struct Factory : MaybeFactoryBase {
static inline return_t New();
static inline return_t New(const char *value, int length = -1);
static inline return_t New(const uint16_t *value, int length = -1);
static inline return_t New(std::string const& value);
static inline return_t New(v8::String::ExternalStringResource * value);
static inline return_t New(ExternalOneByteStringResource * value);
};
template <>
struct Factory : FactoryBase {
static inline return_t New(v8::Local value);
};
} // end of namespace imp
#if (NODE_MODULE_VERSION >= 12)
namespace imp {
template <>
struct Factory : MaybeFactoryBase {
static inline return_t New( v8::Local source);
static inline return_t New( v8::Local source
, v8::ScriptOrigin const& origin);
};
} // end of namespace imp
# include "nan_implementation_12_inl.h"
#else // NODE_MODULE_VERSION >= 12
# include "nan_implementation_pre_12_inl.h"
#endif
//=== API ======================================================================
template
typename imp::Factory::return_t
New() {
return imp::Factory::New();
}
template
typename imp::Factory::return_t
New(A0 arg0) {
return imp::Factory::New(arg0);
}
template
typename imp::Factory::return_t
New(A0 arg0, A1 arg1) {
return imp::Factory::New(arg0, arg1);
}
template
typename imp::Factory::return_t
New(A0 arg0, A1 arg1, A2 arg2) {
return imp::Factory::New(arg0, arg1, arg2);
}
template
typename imp::Factory::return_t
New(A0 arg0, A1 arg1, A2 arg2, A3 arg3) {
return imp::Factory::New(arg0, arg1, arg2, arg3);
}
// Note(agnat): When passing overloaded function pointers to template functions
// as generic arguments the compiler needs help in picking the right overload.
// These two functions handle New and New with
// all argument variations.
// v8::Function and v8::FunctionTemplate with one or two arguments
template
typename imp::Factory::return_t
New( FunctionCallback callback
, v8::Local data = v8::Local()) {
return imp::Factory::New(callback, data);
}
// v8::Function and v8::FunctionTemplate with three arguments
template
typename imp::Factory::return_t
New( FunctionCallback callback
, v8::Local data = v8::Local()
, A2 a2 = A2()) {
return imp::Factory::New(callback, data, a2);
}
// Convenience
#if NODE_MODULE_VERSION < IOJS_3_0_MODULE_VERSION
template inline v8::Local New(v8::Handle h);
#endif
#if NODE_MODULE_VERSION > NODE_0_10_MODULE_VERSION
template
inline v8::Local New(v8::Persistent const& p);
#else
template inline v8::Local New(v8::Persistent const& p);
#endif
template
inline v8::Local New(Persistent const& p);
template
inline v8::Local New(Global const& p);
inline
imp::Factory::return_t
New(bool value) {
return New(value);
}
inline
imp::Factory::return_t
New(int32_t value) {
return New(value);
}
inline
imp::Factory::return_t
New(uint32_t value) {
return New(value);
}
inline
imp::Factory::return_t
New(double value) {
return New(value);
}
inline
imp::Factory::return_t
New(std::string const& value) { // NOLINT(build/include_what_you_use)
return New(value);
}
inline
imp::Factory::return_t
New(const char * value, int length) {
return New(value, length);
}
inline
imp::Factory::return_t
New(const uint16_t * value, int length) {
return New(value, length);
}
inline
imp::Factory::return_t
New(const char * value) {
return New(value);
}
inline
imp::Factory::return_t
New(const uint16_t * value) {
return New(value);
}
inline
imp::Factory::return_t
New(v8::String::ExternalStringResource * value) {
return New(value);
}
inline
imp::Factory::return_t
New(ExternalOneByteStringResource * value) {
return New(value);
}
inline
imp::Factory::return_t
New(v8::Local pattern, v8::RegExp::Flags flags) {
return New(pattern, flags);
}
#endif // NAN_NEW_H_
© 2015 - 2024 Weber Informatics LLC | Privacy Policy