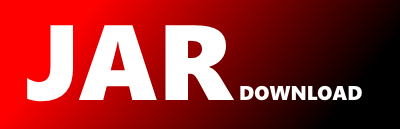
package.lib.BaseSelect.d.ts Maven / Gradle / Ivy
The newest version!
import type { AlignType, BuildInPlacements } from '@rc-component/trigger/lib/interface';
import type { ScrollConfig, ScrollTo } from 'rc-virtual-list/lib/List';
import * as React from 'react';
import type { DisplayInfoType, DisplayValueType, Mode, Placement, RawValueType, RenderDOMFunc, RenderNode } from './interface';
export type { DisplayInfoType, DisplayValueType, Mode, Placement, RenderDOMFunc, RenderNode, RawValueType, };
export interface RefOptionListProps {
onKeyDown: React.KeyboardEventHandler;
onKeyUp: React.KeyboardEventHandler;
scrollTo?: (args: number | ScrollConfig) => void;
}
export type CustomTagProps = {
label: React.ReactNode;
value: any;
disabled: boolean;
onClose: (event?: React.MouseEvent) => void;
closable: boolean;
isMaxTag: boolean;
};
export interface BaseSelectRef {
focus: (options?: FocusOptions) => void;
blur: () => void;
scrollTo: ScrollTo;
}
export interface BaseSelectPrivateProps {
id: string;
prefixCls: string;
omitDomProps?: string[];
displayValues: DisplayValueType[];
onDisplayValuesChange: (values: DisplayValueType[], info: {
type: DisplayInfoType;
values: DisplayValueType[];
}) => void;
/** Current dropdown list active item string value */
activeValue?: string;
/** Link search input with target element */
activeDescendantId?: string;
onActiveValueChange?: (value: string | null) => void;
searchValue: string;
autoClearSearchValue?: boolean;
/** Trigger onSearch, return false to prevent trigger open event */
onSearch: (searchValue: string, info: {
source: 'typing' | 'effect' | 'submit' | 'blur';
}) => void;
/** Trigger when search text match the `tokenSeparators`. Will provide split content */
onSearchSplit?: (words: string[]) => void;
OptionList: React.ForwardRefExoticComponent & React.RefAttributes>;
/** Tell if provided `options` is empty */
emptyOptions: boolean;
}
export type BaseSelectPropsWithoutPrivate = Omit;
export interface BaseSelectProps extends BaseSelectPrivateProps, React.AriaAttributes {
className?: string;
style?: React.CSSProperties;
title?: string;
showSearch?: boolean;
tagRender?: (props: CustomTagProps) => React.ReactElement;
direction?: 'ltr' | 'rtl';
maxLength?: number;
tabIndex?: number;
autoFocus?: boolean;
notFoundContent?: React.ReactNode;
placeholder?: React.ReactNode;
onClear?: () => void;
choiceTransitionName?: string;
mode?: Mode;
disabled?: boolean;
loading?: boolean;
open?: boolean;
defaultOpen?: boolean;
onDropdownVisibleChange?: (open: boolean) => void;
/** @private Internal usage. Do not use in your production. */
getInputElement?: () => JSX.Element;
/** @private Internal usage. Do not use in your production. */
getRawInputElement?: () => JSX.Element;
maxTagTextLength?: number;
maxTagCount?: number | 'responsive';
maxTagPlaceholder?: React.ReactNode | ((omittedValues: DisplayValueType[]) => React.ReactNode);
tokenSeparators?: string[];
allowClear?: boolean | {
clearIcon?: RenderNode;
};
suffixIcon?: RenderNode;
/**
* Clear all icon
* @deprecated Please use `allowClear` instead
**/
clearIcon?: RenderNode;
/** Selector remove icon */
removeIcon?: RenderNode;
animation?: string;
transitionName?: string;
dropdownStyle?: React.CSSProperties;
dropdownClassName?: string;
dropdownMatchSelectWidth?: boolean | number;
dropdownRender?: (menu: React.ReactElement) => React.ReactElement;
dropdownAlign?: AlignType;
placement?: Placement;
builtinPlacements?: BuildInPlacements;
getPopupContainer?: RenderDOMFunc;
showAction?: ('focus' | 'click')[];
onBlur?: React.FocusEventHandler;
onFocus?: React.FocusEventHandler;
onKeyUp?: React.KeyboardEventHandler;
onKeyDown?: React.KeyboardEventHandler;
onMouseDown?: React.MouseEventHandler;
onPopupScroll?: React.UIEventHandler;
onInputKeyDown?: React.KeyboardEventHandler;
onMouseEnter?: React.MouseEventHandler;
onMouseLeave?: React.MouseEventHandler;
onClick?: React.MouseEventHandler;
}
export declare const isMultiple: (mode: Mode) => boolean;
declare const BaseSelect: React.ForwardRefExoticComponent>;
export default BaseSelect;
© 2015 - 2024 Weber Informatics LLC | Privacy Policy