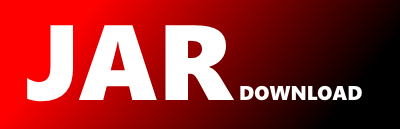
package.dist.esm.exports-mapbox.d.ts Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of react-map-gl Show documentation
Show all versions of react-map-gl Show documentation
React components for Mapbox GL JS-compatible libraries
The newest version!
import * as React from 'react';
import type { Map as MapboxMap, MapboxOptions, Marker as MapboxMarker, MarkerOptions, Popup as MapboxPopup, PopupOptions, AttributionControl as MapboxAttributionControl, FullscreenControl as MapboxFullscreenControl, GeolocateControl as MapboxGeolocateControl, NavigationControl as MapboxNavigationControl, ScaleControl as MapboxScaleControl } from 'mapbox-gl';
import { MapStyle, AnyLayer, AnySource } from './types/style-spec-mapbox';
import { MapProps as _MapProps } from './components/map';
import { MarkerProps as _MarkerProps } from './components/marker';
import { PopupProps as _PopupProps } from './components/popup';
import { AttributionControlProps as _AttributionControlProps } from './components/attribution-control';
import { FullscreenControlProps as _FullscreenControlProps } from './components/fullscreen-control';
import { GeolocateControlProps as _GeolocateControlProps } from './components/geolocate-control';
import { NavigationControlProps as _NavigationControlProps } from './components/navigation-control';
import { ScaleControlProps as _ScaleControlProps } from './components/scale-control';
import { LayerProps as _LayerProps } from './components/layer';
import { SourceProps as _SourceProps } from './components/source';
import type { MapRef as _MapRef } from './mapbox/create-ref';
import type * as events from './types/events';
import type { MapCallbacks } from './types/events-mapbox';
export declare function useMap(): import("./components/use-map").MapCollection;
export declare type MapProps = _MapProps;
export declare type MapRef = _MapRef;
export declare const Map: React.ForwardRefExoticComponent<{
zoom?: number;
bearing?: number;
pitch?: number;
maxZoom?: number;
minZoom?: number;
interactive?: boolean;
minPitch?: number;
maxPitch?: number;
maxBounds?: import("mapbox-gl").LngLatBoundsLike;
projection?: import("mapbox-gl").Projection;
renderWorldCopies?: boolean;
scrollZoom?: boolean | import("mapbox-gl").InteractiveOptions;
boxZoom?: boolean;
dragRotate?: boolean;
dragPan?: boolean | import("mapbox-gl").DragPanOptions;
keyboard?: boolean;
doubleClickZoom?: boolean;
touchZoomRotate?: boolean | import("mapbox-gl").InteractiveOptions;
touchPitch?: boolean | import("mapbox-gl").InteractiveOptions;
accessToken?: string;
localIdeographFontFamily?: string;
antialias?: boolean;
attributionControl?: boolean;
bearingSnap?: number;
clickTolerance?: number;
collectResourceTiming?: boolean;
crossSourceCollisions?: boolean;
cooperativeGestures?: boolean;
customAttribution?: string | string[];
hash?: string | boolean;
fadeDuration?: number;
failIfMajorPerformanceCaveat?: boolean;
locale?: {
[key: string]: string;
};
localFontFamily?: string;
logoPosition?: "top-left" | "top-right" | "bottom-left" | "bottom-right";
optimizeForTerrain?: boolean;
preserveDrawingBuffer?: boolean;
pitchWithRotate?: boolean;
refreshExpiredTiles?: boolean;
trackResize?: boolean;
transformRequest?: import("mapbox-gl").TransformRequestFunction;
maxTileCacheSize?: number;
testMode?: boolean;
worldview?: string;
} & Partial & MapCallbacks & {
mapboxAccessToken?: string;
initialViewState?: Partial & {
bounds?: import("./types").LngLatBoundsLike;
fitBoundsOptions?: {
offset?: import("./types").PointLike;
minZoom?: number;
maxZoom?: number;
padding?: number | import("./types").PaddingOptions;
};
};
gl?: WebGLRenderingContext;
viewState?: import("./types/common").ViewState & {
width: number;
height: number;
};
mapStyle?: string | MapStyle | import("./types").ImmutableLike;
styleDiffing?: boolean;
fog?: import("mapbox-gl").Fog;
light?: import("mapbox-gl").Light;
terrain?: import("mapbox-gl").TerrainSpecification;
interactiveLayerIds?: string[];
cursor?: string;
} & import("./utils/set-globals").GlobalSettings & {
mapLib?: import("./types/lib").MapLib | Promise>;
reuseMaps?: boolean;
id?: string;
style?: React.CSSProperties;
children?: any;
} & React.RefAttributes>;
export declare type MarkerProps = _MarkerProps;
export declare const Marker: (props: MarkerProps & React.RefAttributes) => React.ReactElement | null;
export declare type PopupProps = _PopupProps;
export declare const Popup: (props: PopupProps & React.RefAttributes) => React.ReactElement | null;
declare type AttributionControlOptions = ConstructorParameters[0];
export declare type AttributionControlProps = _AttributionControlProps;
export declare const AttributionControl: (props: AttributionControlProps) => React.ReactElement | null;
declare type FullscreenControlOptions = ConstructorParameters[0];
export declare type FullscreenControlProps = _FullscreenControlProps;
export declare const FullscreenControl: (props: FullscreenControlProps) => React.ReactElement | null;
declare type NavigationControlOptions = ConstructorParameters[0];
export declare type NavigationControlProps = _NavigationControlProps;
export declare const NavigationControl: (props: NavigationControlProps) => React.ReactElement | null;
declare type GeolocateControlOptions = ConstructorParameters[0];
export declare type GeolocateControlProps = _GeolocateControlProps;
export declare const GeolocateControl: (props: GeolocateControlProps & React.RefAttributes) => React.ReactElement | null;
declare type ScaleControlOptions = ConstructorParameters[0];
export declare type ScaleControlProps = _ScaleControlProps;
export declare const ScaleControl: (props: ScaleControlProps) => React.ReactElement | null;
export declare type LayerProps = _LayerProps;
export declare const Layer: (props: LayerProps) => React.ReactElement | null;
export declare type SourceProps = _SourceProps;
export declare const Source: (props: SourceProps) => React.ReactElement | null;
export { default as useControl } from './components/use-control';
export { MapProvider } from './components/use-map';
export default Map;
export * from './types/public';
export type { Point, PointLike, LngLat, LngLatLike, LngLatBounds, LngLatBoundsLike, PaddingOptions, MapboxGeoJSONFeature as MapGeoJSONFeature, GeoJSONSource, VideoSource, ImageSource, CanvasSource, VectorSourceImpl as VectorTileSource } from 'mapbox-gl';
export * from './types/style-spec-mapbox';
export type { MapEvent, MapMouseEvent, MapLayerMouseEvent, MapTouchEvent, MapLayerTouchEvent, MapStyleDataEvent, MapSourceDataEvent, MapWheelEvent, MapBoxZoomEvent, ErrorEvent, ViewStateChangeEvent } from './types/events-mapbox';
export declare type PopupEvent = events.PopupEvent;
export declare type MarkerEvent = events.MarkerEvent;
export declare type MarkerDragEvent = events.MarkerDragEvent;
export declare type GeolocateEvent = events.GeolocateEvent;
export declare type GeolocateResultEvent = events.GeolocateResultEvent;
export declare type GeolocateErrorEvent = events.GeolocateErrorEvent;
/** @deprecated use `MapStyle` */
export declare type MapboxStyle = MapStyle;
export type { Map as MapboxMap, MapboxEvent, MapboxGeoJSONFeature } from 'mapbox-gl';
© 2015 - 2025 Weber Informatics LLC | Privacy Policy