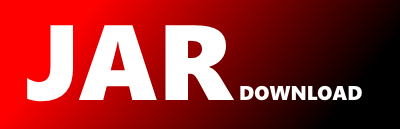
package.dist.development.fog-of-war-BkI3XFdx.d.mts Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of react-router Show documentation
Show all versions of react-router Show documentation
Declarative routing for React
import * as React from 'react';
import { n as RouteObject, F as FutureConfig$1, H as HydrationState, I as InitialEntry, D as DataStrategyFunction, am as PatchRoutesOnNavigationFunction, a as Router$1, T as To, g as RelativeRoutingType, v as NonIndexRouteObject, a0 as LazyRouteFunction, u as IndexRouteObject, h as Location, i as Action, al as Navigator, ao as RouteMatch, r as StaticHandlerContext, d as RouteManifest, R as RouteModules, ak as DataRouteObject, aH as RouteModule, $ as HTMLFormMethod, Z as FormEncType, at as PageLinkDescriptor, aI as History, x as GetScrollRestorationKeyFunction, N as NavigateOptions, y as Fetcher, S as SerializeFrom, B as BlockerFunction } from './route-data-DuV3tXo2.mjs';
/**
* @private
*/
declare function mapRouteProperties(route: RouteObject): Partial & {
hasErrorBoundary: boolean;
};
/**
* @category Routers
*/
declare function createMemoryRouter(routes: RouteObject[], opts?: {
basename?: string;
future?: Partial;
hydrationData?: HydrationState;
initialEntries?: InitialEntry[];
initialIndex?: number;
dataStrategy?: DataStrategyFunction;
patchRoutesOnNavigation?: PatchRoutesOnNavigationFunction;
}): Router$1;
interface RouterProviderProps {
router: Router$1;
flushSync?: (fn: () => unknown) => undefined;
}
/**
* Given a Remix Router instance, render the appropriate UI
*/
declare function RouterProvider({ router, flushSync: reactDomFlushSyncImpl, }: RouterProviderProps): React.ReactElement;
/**
* @category Types
*/
interface MemoryRouterProps {
basename?: string;
children?: React.ReactNode;
initialEntries?: InitialEntry[];
initialIndex?: number;
}
/**
* A `` that stores all entries in memory.
*
* @category Router Components
*/
declare function MemoryRouter({ basename, children, initialEntries, initialIndex, }: MemoryRouterProps): React.ReactElement;
/**
* @category Types
*/
interface NavigateProps {
to: To;
replace?: boolean;
state?: any;
relative?: RelativeRoutingType;
}
/**
* A component-based version of {@link useNavigate} to use in a [`React.Component
* Class`](https://reactjs.org/docs/react-component.html) where hooks are not
* able to be used.
*
* It's recommended to avoid using this component in favor of {@link useNavigate}
*
* @category Components
*/
declare function Navigate({ to, replace, state, relative, }: NavigateProps): null;
/**
* @category Types
*/
interface OutletProps {
/**
Provides a context value to the element tree below the outlet. Use when the parent route needs to provide values to child routes.
```tsx
```
Access the context with {@link useOutletContext}.
*/
context?: unknown;
}
/**
Renders the matching child route of a parent route or nothing if no child route matches.
```tsx
import { Outlet } from "react-router"
export default function SomeParent() {
return (
Parent Content
);
}
```
@category Components
*/
declare function Outlet(props: OutletProps): React.ReactElement | null;
/**
* @category Types
*/
interface PathRouteProps {
caseSensitive?: NonIndexRouteObject["caseSensitive"];
path?: NonIndexRouteObject["path"];
id?: NonIndexRouteObject["id"];
lazy?: LazyRouteFunction;
loader?: NonIndexRouteObject["loader"];
action?: NonIndexRouteObject["action"];
hasErrorBoundary?: NonIndexRouteObject["hasErrorBoundary"];
shouldRevalidate?: NonIndexRouteObject["shouldRevalidate"];
handle?: NonIndexRouteObject["handle"];
index?: false;
children?: React.ReactNode;
element?: React.ReactNode | null;
hydrateFallbackElement?: React.ReactNode | null;
errorElement?: React.ReactNode | null;
Component?: React.ComponentType | null;
HydrateFallback?: React.ComponentType | null;
ErrorBoundary?: React.ComponentType | null;
}
/**
* @category Types
*/
interface LayoutRouteProps extends PathRouteProps {
}
/**
* @category Types
*/
interface IndexRouteProps {
caseSensitive?: IndexRouteObject["caseSensitive"];
path?: IndexRouteObject["path"];
id?: IndexRouteObject["id"];
lazy?: LazyRouteFunction;
loader?: IndexRouteObject["loader"];
action?: IndexRouteObject["action"];
hasErrorBoundary?: IndexRouteObject["hasErrorBoundary"];
shouldRevalidate?: IndexRouteObject["shouldRevalidate"];
handle?: IndexRouteObject["handle"];
index: true;
children?: undefined;
element?: React.ReactNode | null;
hydrateFallbackElement?: React.ReactNode | null;
errorElement?: React.ReactNode | null;
Component?: React.ComponentType | null;
HydrateFallback?: React.ComponentType | null;
ErrorBoundary?: React.ComponentType | null;
}
type RouteProps = PathRouteProps | LayoutRouteProps | IndexRouteProps;
/**
* Configures an element to render when a pattern matches the current location.
* It must be rendered within a {@link Routes} element. Note that these routes
* do not participate in data loading, actions, code splitting, or any other
* route module features.
*
* @category Components
*/
declare function Route$1(_props: RouteProps): React.ReactElement | null;
/**
* @category Types
*/
interface RouterProps {
basename?: string;
children?: React.ReactNode;
location: Partial | string;
navigationType?: Action;
navigator: Navigator;
static?: boolean;
}
/**
* Provides location context for the rest of the app.
*
* Note: You usually won't render a `` directly. Instead, you'll render a
* router that is more specific to your environment such as a ``
* in web browsers or a `` for server rendering.
*
* @category Components
*/
declare function Router({ basename: basenameProp, children, location: locationProp, navigationType, navigator, static: staticProp, }: RouterProps): React.ReactElement | null;
/**
* @category Types
*/
interface RoutesProps {
/**
* Nested {@link Route} elements
*/
children?: React.ReactNode;
/**
* The location to match against. Defaults to the current location.
*/
location?: Partial | string;
}
/**
Renders a branch of {@link Route | ``} that best matches the current
location. Note that these routes do not participate in data loading, actions,
code splitting, or any other route module features.
```tsx
import { Routes, Route } from "react-router"
} />
} />
}>
```
@category Components
*/
declare function Routes({ children, location, }: RoutesProps): React.ReactElement | null;
interface AwaitResolveRenderFunction {
(data: Awaited): React.ReactNode;
}
/**
* @category Types
*/
interface AwaitProps {
/**
When using a function, the resolved value is provided as the parameter.
```tsx [2]
{(resolvedReviews) => }
```
When using React elements, {@link useAsyncValue} will provide the
resolved value:
```tsx [2]
function Reviews() {
const resolvedReviews = useAsyncValue()
return ...
}
```
*/
children: React.ReactNode | AwaitResolveRenderFunction;
/**
The error element renders instead of the children when the promise rejects.
```tsx
Oops
Error loading reviews: {error.message}
}
```
If you do not provide an errorElement, the rejected value will bubble up to
the nearest route-level {@link NonIndexRouteObject#ErrorBoundary | ErrorBoundary} and be accessible
via {@link useRouteError} hook.
*/
errorElement?: React.ReactNode;
/**
Takes a promise returned from a {@link LoaderFunction | loader} value to be resolved and rendered.
```jsx
import { useLoaderData, Await } from "react-router"
export async function loader() {
let reviews = getReviews() // not awaited
let book = await getBook()
return {
book,
reviews, // this is a promise
}
}
export default function Book() {
const {
book,
reviews, // this is the same promise
} = useLoaderData()
return (
{book.title}
{book.description}
{book.title}
{book.description}