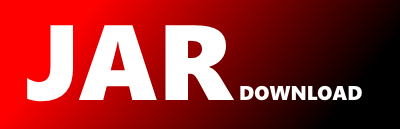
org.mybatis.guice.datasource.dbcp.DriverAdapterCPDSProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of mybatis-guice Show documentation
Show all versions of mybatis-guice Show documentation
The MyBatis Guice module is easy-to-use Google Guice bridge for MyBatis sql mapping framework.
/*
* Copyright 2010 The myBatis Team
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.mybatis.guice.datasource.dbcp;
import javax.sql.ConnectionPoolDataSource;
import org.apache.commons.dbcp.cpdsadapter.DriverAdapterCPDS;
import com.google.inject.Inject;
import com.google.inject.Provider;
import com.google.inject.Singleton;
import com.google.inject.name.Named;
/**
* Provides the Apache commons-dbcp {@code DriverAdapterCPDS}.
*
* @version $Id: DriverAdapterCPDSProvider.java 2229 2010-08-03 19:54:49Z simone.tripodi $
*/
@Singleton
public final class DriverAdapterCPDSProvider implements Provider {
private final DriverAdapterCPDS adapter = new DriverAdapterCPDS();
@Inject
public DriverAdapterCPDSProvider(@Named("JDBC.driver") final String driver,
@Named("JDBC.url") final String url,
@Named("JDBC.username") final String username,
@Named("JDBC.password") final String password) {
try {
this.adapter.setDriver(driver);
} catch (ClassNotFoundException e) {
throw new RuntimeException("Driver '"
+ driver
+ "' not found in the classpath", e);
}
this.adapter.setUrl(url);
this.adapter.setUser(username);
this.adapter.setPassword(password);
}
@Inject(optional = true)
public void setDescription(@Named("DBCP.description") String description) {
this.adapter.setDescription(description);
}
@Inject(optional = true)
public void setLoginTimeout(@Named("JDBC.loginTimeout") int seconds) {
this.adapter.setLoginTimeout(seconds);
}
@Inject(optional = true)
public void setMaxActive(@Named("DBCP.maxActive") int maxActive) {
this.adapter.setMaxActive(maxActive);
}
@Inject(optional = true)
public void setMaxIdle(@Named("DBCP.maxIdle") int maxIdle) {
this.adapter.setMaxIdle(maxIdle);
}
@Inject(optional = true)
public void setMaxPreparedStatements(@Named("DBCP.maxOpenPreparedStatements") int maxPreparedStatements) {
this.adapter.setMaxPreparedStatements(maxPreparedStatements);
}
@Inject(optional = true)
public void setMinEvictableIdleTimeMillis(@Named("DBCP.minEvictableIdleTimeMillis") int minEvictableIdleTimeMillis) {
this.adapter.setMinEvictableIdleTimeMillis(minEvictableIdleTimeMillis);
}
@Inject(optional = true)
public void setNumTestsPerEvictionRun(@Named("DBCP.numTestsPerEvictionRun") int numTestsPerEvictionRun) {
this.adapter.setNumTestsPerEvictionRun(numTestsPerEvictionRun);
}
@Inject(optional = true)
public void setPoolPreparedStatements(@Named("DBCP.poolPreparedStatements") boolean poolPreparedStatements) {
this.adapter.setPoolPreparedStatements(poolPreparedStatements);
}
@Inject(optional = true)
public void setTimeBetweenEvictionRunsMillis(@Named("DBCP.timeBetweenEvictionRunsMillis") int timeBetweenEvictionRunsMillis) {
this.adapter.setTimeBetweenEvictionRunsMillis(timeBetweenEvictionRunsMillis);
}
public ConnectionPoolDataSource get() {
return this.adapter;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy