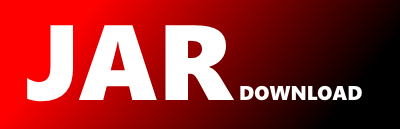
org.myire.collection.Sequences Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of thrice Show documentation
Show all versions of thrice Show documentation
Java classes that have come in handy three times or more
/*
* Copyright 2013, 2017 Peter Franzen. All rights reserved.
*
* Licensed under the Apache License v2.0: http://www.apache.org/licenses/LICENSE-2.0
*/
package org.myire.collection;
import java.util.List;
import java.util.function.Consumer;
import static java.util.Objects.requireNonNull;
import javax.annotation.Nonnegative;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import org.myire.annotation.Unreachable;
/**
* Factory methods for {@code Sequence} implementations.
*
* @author Peter Franzen
*/
public final class Sequences
{
/**
* Private constructor to disallow instantiations of utility method class.
*/
@Unreachable
private Sequences()
{
// Empty default ctor, defined to override access scope.
}
/**
* Get the empty sequence. This is an immutable sequence of size 0.
*
* @param The sequence's element type.
*
* @return The empty sequence.
*/
@SuppressWarnings("unchecked")
@Nonnull
static public Sequence emptySequence()
{
return (Sequence) EmptySequence.INSTANCE;
}
/**
* Create a sequence that holds a single element.
*
* @param pElement The sequence's sole element.
*
* @param The sequence's element type.
*
* @return A singleton sequence, never null.
*/
@Nonnull
static public Sequence singleton(@Nullable T pElement)
{
return new SingletonSequence<>(pElement);
}
/**
* Create a {@code Sequence} that is a wrapper around the elements in a {@code List}. The
* returned {@code Sequence} will reflect changes made to the specified list. Added elements
* will be accessible through the sequence, removed elements will disappear from the sequence.
*
* @param pList The list to wrap.
*
* @param The sequence's element type.
*
* @return A new {@code Sequence}, never null.
*
* @throws NullPointerException if {@code pList} is null.
*/
@Nonnull
static public Sequence wrap(@Nonnull List extends T> pList)
{
return new ListSequence<>(pList);
}
/**
* Create a {@code Sequence} that is a wrapper around the elements in an array. The returned
* {@code Sequence} will reflect changes made to the elements in the array. Replacing an element
* in the array will also replace the element in the sequence.
*
* @param pElements The array to wrap.
*
* @param The sequence's element type.
*
* @return A new {@code Sequence}, never null.
*
* @throws NullPointerException if {@code pElements} is null.
*/
@Nonnull
static public Sequence wrap(@Nonnull T[] pElements)
{
return pElements.length == 0 ? emptySequence() : new ArraySequence<>(pElements);
}
/**
* Immutable implementation of an empty {@code Sequence}.
*
* @param The sequence's element type.
*/
static private class EmptySequence implements Sequence
{
static final Sequence
© 2015 - 2025 Weber Informatics LLC | Privacy Policy