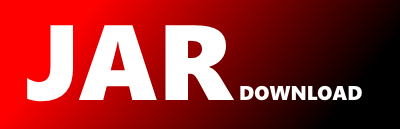
org.n52.matlab.control.extensions.CallbackMatlabProxy Maven / Gradle / Ivy
The newest version!
package org.n52.matlab.control.extensions;
/*
* Copyright (c) 2013, Joshua Kaplan
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without modification, are permitted provided that the
* following conditions are met:
* - Redistributions of source code must retain the above copyright notice, this list of conditions and the following
* disclaimer.
* - Redistributions in binary form must reproduce the above copyright notice, this list of conditions and the
* following disclaimer in the documentation and/or other materials provided with the distribution.
* - Neither the name of matlabcontrol nor the names of its contributors may be used to endorse or promote products
* derived from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES,
* INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
* DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL,
* SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR
* SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY,
* WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE
* OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import java.util.concurrent.ThreadFactory;
import org.n52.matlab.control.MatlabInvocationException;
import org.n52.matlab.control.MatlabProxy.MatlabThreadCallable;
import org.n52.matlab.control.MatlabProxy;
/**
* @deprecated This class was provided as a workaround when {@link MatlabProxy} could not operate on the Event Dispatch
* Thread (EDT) used by AWT and Swing; {@code MatlabProxy} no longer has this limitation.
*
* Wraps around a {@link MatlabProxy} making the method calls which interact with MATLAB operate with callbacks instead
* of return values. For each method in {@code MatlabProxy} that interacts with MATLAB, the same method exists but has
* one additional parameter that is either {@link MatlabCallback} or {@link MatlabDataCallback}. Method invocations do
* not throw {@link MatlabInvocationException}s, but if the proxy throws a {@code MatlabInvocationException} it will be
* provided to the callback.
*
* All interactions with the proxy will be done in a single threaded manner. The underlying proxy methods will be
* completed in the order their corresponding methods in this class were called. Because method invocations on the
* proxy occur on a separate thread from the one calling the methods in this class, it can be used from within MATLAB on
* the Event Dispatch Thread (EDT).
*
* This class is unconditionally thread-safe. There are no guarantees about the relative ordering of method completion
* when methods are invoked both on an instance of this class and on the proxy provided to it.
*
* @since 4.0.0
*
* @author Joshua Kaplan
*/
public class CallbackMatlabProxy
{
/**
* Executor that manages the single daemon thread used to invoke methods on the proxy.
*/
private final ExecutorService _executor = Executors.newFixedThreadPool(1, new DaemonThreadFactory());
private final MatlabProxy _proxy;
/**
* Constructs an instance of this class, delegating all method invocations to the {@code proxy}.
*
* @param proxy
*/
public CallbackMatlabProxy(MatlabProxy proxy)
{
_proxy = proxy;
}
/**
* Returns a brief description. The exact details of this representation are unspecified and are subject to change.
*
* @return
*/
@Override
public String toString()
{
return "[" + this.getClass().getName() + " proxy=" + _proxy + "]";
}
/**
* Delegates to the proxy, calling the {@code callback} when the proxy's corresponding method has completed.
*/
public void isConnected(final MatlabDataCallback callback)
{
_executor.submit(new Runnable()
{
@Override
public void run()
{
boolean connected = _proxy.isConnected();
callback.invocationSucceeded(connected);
}
});
}
/**
* Delegates to the proxy, calling the {@code callback} when the proxy's corresponding method has completed.
*
* @param callback
*/
public void disconnect(final MatlabDataCallback callback)
{
_executor.submit(new Runnable()
{
@Override
public void run()
{
boolean succeeded = _proxy.disconnect();
callback.invocationSucceeded(succeeded);
}
});
}
/**
* Delegates to the proxy, calling the {@code callback} when the proxy's corresponding method has completed.
*
* @param callback
*/
public void exit(final MatlabCallback callback)
{
_executor.submit(new Runnable()
{
@Override
public void run()
{
try
{
_proxy.exit();
callback.invocationSucceeded();
}
catch(MatlabInvocationException e)
{
callback.invocationFailed(e);
}
}
});
}
/**
* Delegates to the proxy, calling the {@code callback} when the proxy's corresponding method has completed.
*
* @param callback
* @param command
*/
public void eval(final MatlabCallback callback, final String command)
{
_executor.submit(new Runnable()
{
@Override
public void run()
{
try
{
_proxy.eval(command);
callback.invocationSucceeded();
}
catch(MatlabInvocationException e)
{
callback.invocationFailed(e);
}
}
});
}
/**
* Delegates to the proxy, calling the {@code callback} when the proxy's corresponding method has completed.
*
* @param callback
* @param command
* @param nargout
*/
public void returningEval(final MatlabDataCallback
© 2015 - 2024 Weber Informatics LLC | Privacy Policy