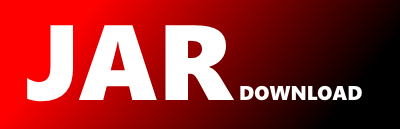
net.opengis.swe.x101.impl.AllowedTokensDocumentImpl Maven / Gradle / Ivy
/*
* An XML document type.
* Localname: AllowedTokens
* Namespace: http://www.opengis.net/swe/1.0.1
* Java type: net.opengis.swe.x101.AllowedTokensDocument
*
* Automatically generated - do not modify.
*/
package net.opengis.swe.x101.impl;
/**
* A document containing one AllowedTokens(@http://www.opengis.net/swe/1.0.1) element.
*
* This is a complex type.
*/
public class AllowedTokensDocumentImpl extends org.apache.xmlbeans.impl.values.XmlComplexContentImpl implements net.opengis.swe.x101.AllowedTokensDocument
{
private static final long serialVersionUID = 1L;
public AllowedTokensDocumentImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
private static final javax.xml.namespace.QName ALLOWEDTOKENS$0 =
new javax.xml.namespace.QName("http://www.opengis.net/swe/1.0.1", "AllowedTokens");
/**
* Gets the "AllowedTokens" element
*/
public net.opengis.swe.x101.AllowedTokensDocument.AllowedTokens getAllowedTokens()
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.AllowedTokensDocument.AllowedTokens target = null;
target = (net.opengis.swe.x101.AllowedTokensDocument.AllowedTokens)get_store().find_element_user(ALLOWEDTOKENS$0, 0);
if (target == null)
{
return null;
}
return target;
}
}
/**
* Sets the "AllowedTokens" element
*/
public void setAllowedTokens(net.opengis.swe.x101.AllowedTokensDocument.AllowedTokens allowedTokens)
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.AllowedTokensDocument.AllowedTokens target = null;
target = (net.opengis.swe.x101.AllowedTokensDocument.AllowedTokens)get_store().find_element_user(ALLOWEDTOKENS$0, 0);
if (target == null)
{
target = (net.opengis.swe.x101.AllowedTokensDocument.AllowedTokens)get_store().add_element_user(ALLOWEDTOKENS$0);
}
target.set(allowedTokens);
}
}
/**
* Appends and returns a new empty "AllowedTokens" element
*/
public net.opengis.swe.x101.AllowedTokensDocument.AllowedTokens addNewAllowedTokens()
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.AllowedTokensDocument.AllowedTokens target = null;
target = (net.opengis.swe.x101.AllowedTokensDocument.AllowedTokens)get_store().add_element_user(ALLOWEDTOKENS$0);
return target;
}
}
/**
* An XML AllowedTokens(@http://www.opengis.net/swe/1.0.1).
*
* This is a complex type.
*/
public static class AllowedTokensImpl extends org.apache.xmlbeans.impl.values.XmlComplexContentImpl implements net.opengis.swe.x101.AllowedTokensDocument.AllowedTokens
{
private static final long serialVersionUID = 1L;
public AllowedTokensImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
private static final javax.xml.namespace.QName VALUELIST$0 =
new javax.xml.namespace.QName("http://www.opengis.net/swe/1.0.1", "valueList");
private static final javax.xml.namespace.QName ID$2 =
new javax.xml.namespace.QName("", "id");
/**
* Gets array of all "valueList" elements
*/
public java.util.List[] getValueListArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(VALUELIST$0, targetList);
java.util.List[] result = new java.util.List[targetList.size()];
for (int i = 0, len = targetList.size() ; i < len ; i++)
result[i] = ((org.apache.xmlbeans.SimpleValue)targetList.get(i)).getListValue();
return result;
}
}
/**
* Gets ith "valueList" element
*/
public java.util.List getValueListArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_element_user(VALUELIST$0, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target.getListValue();
}
}
/**
* Gets (as xml) array of all "valueList" elements
*/
public net.opengis.swe.x101.TokenList[] xgetValueListArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(VALUELIST$0, targetList);
net.opengis.swe.x101.TokenList[] result = new net.opengis.swe.x101.TokenList[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets (as xml) ith "valueList" element
*/
public net.opengis.swe.x101.TokenList xgetValueListArray(int i)
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.TokenList target = null;
target = (net.opengis.swe.x101.TokenList)get_store().find_element_user(VALUELIST$0, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return (net.opengis.swe.x101.TokenList)target;
}
}
/**
* Returns number of "valueList" element
*/
public int sizeOfValueListArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(VALUELIST$0);
}
}
/**
* Sets array of all "valueList" element
*/
public void setValueListArray(java.util.List[] valueListArray)
{
synchronized (monitor())
{
check_orphaned();
arraySetterHelper(valueListArray, VALUELIST$0);
}
}
/**
* Sets ith "valueList" element
*/
public void setValueListArray(int i, java.util.List valueList)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_element_user(VALUELIST$0, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
target.setListValue(valueList);
}
}
/**
* Sets (as xml) array of all "valueList" element
*/
public void xsetValueListArray(net.opengis.swe.x101.TokenList[]valueListArray)
{
synchronized (monitor())
{
check_orphaned();
arraySetterHelper(valueListArray, VALUELIST$0);
}
}
/**
* Sets (as xml) ith "valueList" element
*/
public void xsetValueListArray(int i, net.opengis.swe.x101.TokenList valueList)
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.TokenList target = null;
target = (net.opengis.swe.x101.TokenList)get_store().find_element_user(VALUELIST$0, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
target.set(valueList);
}
}
/**
* Inserts the value as the ith "valueList" element
*/
public void insertValueList(int i, java.util.List valueList)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target =
(org.apache.xmlbeans.SimpleValue)get_store().insert_element_user(VALUELIST$0, i);
target.setListValue(valueList);
}
}
/**
* Appends the value as the last "valueList" element
*/
public void addValueList(java.util.List valueList)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().add_element_user(VALUELIST$0);
target.setListValue(valueList);
}
}
/**
* Inserts and returns a new empty value (as xml) as the ith "valueList" element
*/
public net.opengis.swe.x101.TokenList insertNewValueList(int i)
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.TokenList target = null;
target = (net.opengis.swe.x101.TokenList)get_store().insert_element_user(VALUELIST$0, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "valueList" element
*/
public net.opengis.swe.x101.TokenList addNewValueList()
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.TokenList target = null;
target = (net.opengis.swe.x101.TokenList)get_store().add_element_user(VALUELIST$0);
return target;
}
}
/**
* Removes the ith "valueList" element
*/
public void removeValueList(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(VALUELIST$0, i);
}
}
/**
* Gets the "id" attribute
*/
public java.lang.String getId()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(ID$2);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "id" attribute
*/
public org.apache.xmlbeans.XmlID xgetId()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlID target = null;
target = (org.apache.xmlbeans.XmlID)get_store().find_attribute_user(ID$2);
return target;
}
}
/**
* True if has "id" attribute
*/
public boolean isSetId()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(ID$2) != null;
}
}
/**
* Sets the "id" attribute
*/
public void setId(java.lang.String id)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(ID$2);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(ID$2);
}
target.setStringValue(id);
}
}
/**
* Sets (as xml) the "id" attribute
*/
public void xsetId(org.apache.xmlbeans.XmlID id)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlID target = null;
target = (org.apache.xmlbeans.XmlID)get_store().find_attribute_user(ID$2);
if (target == null)
{
target = (org.apache.xmlbeans.XmlID)get_store().add_attribute_user(ID$2);
}
target.set(id);
}
}
/**
* Unsets the "id" attribute
*/
public void unsetId()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(ID$2);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy