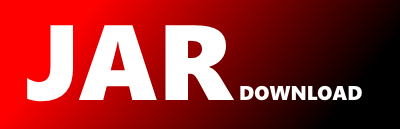
net.opengis.swe.x101.impl.AllowedValuesDocumentImpl Maven / Gradle / Ivy
/*
* An XML document type.
* Localname: AllowedValues
* Namespace: http://www.opengis.net/swe/1.0.1
* Java type: net.opengis.swe.x101.AllowedValuesDocument
*
* Automatically generated - do not modify.
*/
package net.opengis.swe.x101.impl;
/**
* A document containing one AllowedValues(@http://www.opengis.net/swe/1.0.1) element.
*
* This is a complex type.
*/
public class AllowedValuesDocumentImpl extends org.apache.xmlbeans.impl.values.XmlComplexContentImpl implements net.opengis.swe.x101.AllowedValuesDocument
{
private static final long serialVersionUID = 1L;
public AllowedValuesDocumentImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
private static final javax.xml.namespace.QName ALLOWEDVALUES$0 =
new javax.xml.namespace.QName("http://www.opengis.net/swe/1.0.1", "AllowedValues");
/**
* Gets the "AllowedValues" element
*/
public net.opengis.swe.x101.AllowedValuesDocument.AllowedValues getAllowedValues()
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.AllowedValuesDocument.AllowedValues target = null;
target = (net.opengis.swe.x101.AllowedValuesDocument.AllowedValues)get_store().find_element_user(ALLOWEDVALUES$0, 0);
if (target == null)
{
return null;
}
return target;
}
}
/**
* Sets the "AllowedValues" element
*/
public void setAllowedValues(net.opengis.swe.x101.AllowedValuesDocument.AllowedValues allowedValues)
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.AllowedValuesDocument.AllowedValues target = null;
target = (net.opengis.swe.x101.AllowedValuesDocument.AllowedValues)get_store().find_element_user(ALLOWEDVALUES$0, 0);
if (target == null)
{
target = (net.opengis.swe.x101.AllowedValuesDocument.AllowedValues)get_store().add_element_user(ALLOWEDVALUES$0);
}
target.set(allowedValues);
}
}
/**
* Appends and returns a new empty "AllowedValues" element
*/
public net.opengis.swe.x101.AllowedValuesDocument.AllowedValues addNewAllowedValues()
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.AllowedValuesDocument.AllowedValues target = null;
target = (net.opengis.swe.x101.AllowedValuesDocument.AllowedValues)get_store().add_element_user(ALLOWEDVALUES$0);
return target;
}
}
/**
* An XML AllowedValues(@http://www.opengis.net/swe/1.0.1).
*
* This is a complex type.
*/
public static class AllowedValuesImpl extends org.apache.xmlbeans.impl.values.XmlComplexContentImpl implements net.opengis.swe.x101.AllowedValuesDocument.AllowedValues
{
private static final long serialVersionUID = 1L;
public AllowedValuesImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
private static final javax.xml.namespace.QName MIN$0 =
new javax.xml.namespace.QName("http://www.opengis.net/swe/1.0.1", "min");
private static final javax.xml.namespace.QName MAX$2 =
new javax.xml.namespace.QName("http://www.opengis.net/swe/1.0.1", "max");
private static final javax.xml.namespace.QName INTERVAL$4 =
new javax.xml.namespace.QName("http://www.opengis.net/swe/1.0.1", "interval");
private static final javax.xml.namespace.QName VALUELIST$6 =
new javax.xml.namespace.QName("http://www.opengis.net/swe/1.0.1", "valueList");
private static final javax.xml.namespace.QName ID$8 =
new javax.xml.namespace.QName("", "id");
/**
* Gets the "min" element
*/
public double getMin()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_element_user(MIN$0, 0);
if (target == null)
{
return 0.0;
}
return target.getDoubleValue();
}
}
/**
* Gets (as xml) the "min" element
*/
public org.apache.xmlbeans.XmlDouble xgetMin()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlDouble target = null;
target = (org.apache.xmlbeans.XmlDouble)get_store().find_element_user(MIN$0, 0);
return target;
}
}
/**
* True if has "min" element
*/
public boolean isSetMin()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(MIN$0) != 0;
}
}
/**
* Sets the "min" element
*/
public void setMin(double min)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_element_user(MIN$0, 0);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_element_user(MIN$0);
}
target.setDoubleValue(min);
}
}
/**
* Sets (as xml) the "min" element
*/
public void xsetMin(org.apache.xmlbeans.XmlDouble min)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlDouble target = null;
target = (org.apache.xmlbeans.XmlDouble)get_store().find_element_user(MIN$0, 0);
if (target == null)
{
target = (org.apache.xmlbeans.XmlDouble)get_store().add_element_user(MIN$0);
}
target.set(min);
}
}
/**
* Unsets the "min" element
*/
public void unsetMin()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(MIN$0, 0);
}
}
/**
* Gets the "max" element
*/
public double getMax()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_element_user(MAX$2, 0);
if (target == null)
{
return 0.0;
}
return target.getDoubleValue();
}
}
/**
* Gets (as xml) the "max" element
*/
public org.apache.xmlbeans.XmlDouble xgetMax()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlDouble target = null;
target = (org.apache.xmlbeans.XmlDouble)get_store().find_element_user(MAX$2, 0);
return target;
}
}
/**
* True if has "max" element
*/
public boolean isSetMax()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(MAX$2) != 0;
}
}
/**
* Sets the "max" element
*/
public void setMax(double max)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_element_user(MAX$2, 0);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_element_user(MAX$2);
}
target.setDoubleValue(max);
}
}
/**
* Sets (as xml) the "max" element
*/
public void xsetMax(org.apache.xmlbeans.XmlDouble max)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlDouble target = null;
target = (org.apache.xmlbeans.XmlDouble)get_store().find_element_user(MAX$2, 0);
if (target == null)
{
target = (org.apache.xmlbeans.XmlDouble)get_store().add_element_user(MAX$2);
}
target.set(max);
}
}
/**
* Unsets the "max" element
*/
public void unsetMax()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(MAX$2, 0);
}
}
/**
* Gets array of all "interval" elements
*/
public java.util.List[] getIntervalArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(INTERVAL$4, targetList);
java.util.List[] result = new java.util.List[targetList.size()];
for (int i = 0, len = targetList.size() ; i < len ; i++)
result[i] = ((org.apache.xmlbeans.SimpleValue)targetList.get(i)).getListValue();
return result;
}
}
/**
* Gets ith "interval" element
*/
public java.util.List getIntervalArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_element_user(INTERVAL$4, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target.getListValue();
}
}
/**
* Gets (as xml) array of all "interval" elements
*/
public net.opengis.swe.x101.DecimalPair[] xgetIntervalArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(INTERVAL$4, targetList);
net.opengis.swe.x101.DecimalPair[] result = new net.opengis.swe.x101.DecimalPair[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets (as xml) ith "interval" element
*/
public net.opengis.swe.x101.DecimalPair xgetIntervalArray(int i)
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.DecimalPair target = null;
target = (net.opengis.swe.x101.DecimalPair)get_store().find_element_user(INTERVAL$4, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return (net.opengis.swe.x101.DecimalPair)target;
}
}
/**
* Returns number of "interval" element
*/
public int sizeOfIntervalArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(INTERVAL$4);
}
}
/**
* Sets array of all "interval" element
*/
public void setIntervalArray(java.util.List[] intervalArray)
{
synchronized (monitor())
{
check_orphaned();
arraySetterHelper(intervalArray, INTERVAL$4);
}
}
/**
* Sets ith "interval" element
*/
public void setIntervalArray(int i, java.util.List interval)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_element_user(INTERVAL$4, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
target.setListValue(interval);
}
}
/**
* Sets (as xml) array of all "interval" element
*/
public void xsetIntervalArray(net.opengis.swe.x101.DecimalPair[]intervalArray)
{
synchronized (monitor())
{
check_orphaned();
arraySetterHelper(intervalArray, INTERVAL$4);
}
}
/**
* Sets (as xml) ith "interval" element
*/
public void xsetIntervalArray(int i, net.opengis.swe.x101.DecimalPair interval)
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.DecimalPair target = null;
target = (net.opengis.swe.x101.DecimalPair)get_store().find_element_user(INTERVAL$4, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
target.set(interval);
}
}
/**
* Inserts the value as the ith "interval" element
*/
public void insertInterval(int i, java.util.List interval)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target =
(org.apache.xmlbeans.SimpleValue)get_store().insert_element_user(INTERVAL$4, i);
target.setListValue(interval);
}
}
/**
* Appends the value as the last "interval" element
*/
public void addInterval(java.util.List interval)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().add_element_user(INTERVAL$4);
target.setListValue(interval);
}
}
/**
* Inserts and returns a new empty value (as xml) as the ith "interval" element
*/
public net.opengis.swe.x101.DecimalPair insertNewInterval(int i)
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.DecimalPair target = null;
target = (net.opengis.swe.x101.DecimalPair)get_store().insert_element_user(INTERVAL$4, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "interval" element
*/
public net.opengis.swe.x101.DecimalPair addNewInterval()
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.DecimalPair target = null;
target = (net.opengis.swe.x101.DecimalPair)get_store().add_element_user(INTERVAL$4);
return target;
}
}
/**
* Removes the ith "interval" element
*/
public void removeInterval(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(INTERVAL$4, i);
}
}
/**
* Gets array of all "valueList" elements
*/
public java.util.List[] getValueListArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(VALUELIST$6, targetList);
java.util.List[] result = new java.util.List[targetList.size()];
for (int i = 0, len = targetList.size() ; i < len ; i++)
result[i] = ((org.apache.xmlbeans.SimpleValue)targetList.get(i)).getListValue();
return result;
}
}
/**
* Gets ith "valueList" element
*/
public java.util.List getValueListArray(int i)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_element_user(VALUELIST$6, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target.getListValue();
}
}
/**
* Gets (as xml) array of all "valueList" elements
*/
public net.opengis.swe.x101.DecimalList[] xgetValueListArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(VALUELIST$6, targetList);
net.opengis.swe.x101.DecimalList[] result = new net.opengis.swe.x101.DecimalList[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets (as xml) ith "valueList" element
*/
public net.opengis.swe.x101.DecimalList xgetValueListArray(int i)
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.DecimalList target = null;
target = (net.opengis.swe.x101.DecimalList)get_store().find_element_user(VALUELIST$6, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return (net.opengis.swe.x101.DecimalList)target;
}
}
/**
* Returns number of "valueList" element
*/
public int sizeOfValueListArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(VALUELIST$6);
}
}
/**
* Sets array of all "valueList" element
*/
public void setValueListArray(java.util.List[] valueListArray)
{
synchronized (monitor())
{
check_orphaned();
arraySetterHelper(valueListArray, VALUELIST$6);
}
}
/**
* Sets ith "valueList" element
*/
public void setValueListArray(int i, java.util.List valueList)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_element_user(VALUELIST$6, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
target.setListValue(valueList);
}
}
/**
* Sets (as xml) array of all "valueList" element
*/
public void xsetValueListArray(net.opengis.swe.x101.DecimalList[]valueListArray)
{
synchronized (monitor())
{
check_orphaned();
arraySetterHelper(valueListArray, VALUELIST$6);
}
}
/**
* Sets (as xml) ith "valueList" element
*/
public void xsetValueListArray(int i, net.opengis.swe.x101.DecimalList valueList)
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.DecimalList target = null;
target = (net.opengis.swe.x101.DecimalList)get_store().find_element_user(VALUELIST$6, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
target.set(valueList);
}
}
/**
* Inserts the value as the ith "valueList" element
*/
public void insertValueList(int i, java.util.List valueList)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target =
(org.apache.xmlbeans.SimpleValue)get_store().insert_element_user(VALUELIST$6, i);
target.setListValue(valueList);
}
}
/**
* Appends the value as the last "valueList" element
*/
public void addValueList(java.util.List valueList)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().add_element_user(VALUELIST$6);
target.setListValue(valueList);
}
}
/**
* Inserts and returns a new empty value (as xml) as the ith "valueList" element
*/
public net.opengis.swe.x101.DecimalList insertNewValueList(int i)
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.DecimalList target = null;
target = (net.opengis.swe.x101.DecimalList)get_store().insert_element_user(VALUELIST$6, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "valueList" element
*/
public net.opengis.swe.x101.DecimalList addNewValueList()
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.DecimalList target = null;
target = (net.opengis.swe.x101.DecimalList)get_store().add_element_user(VALUELIST$6);
return target;
}
}
/**
* Removes the ith "valueList" element
*/
public void removeValueList(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(VALUELIST$6, i);
}
}
/**
* Gets the "id" attribute
*/
public java.lang.String getId()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(ID$8);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "id" attribute
*/
public org.apache.xmlbeans.XmlID xgetId()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlID target = null;
target = (org.apache.xmlbeans.XmlID)get_store().find_attribute_user(ID$8);
return target;
}
}
/**
* True if has "id" attribute
*/
public boolean isSetId()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(ID$8) != null;
}
}
/**
* Sets the "id" attribute
*/
public void setId(java.lang.String id)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(ID$8);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(ID$8);
}
target.setStringValue(id);
}
}
/**
* Sets (as xml) the "id" attribute
*/
public void xsetId(org.apache.xmlbeans.XmlID id)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlID target = null;
target = (org.apache.xmlbeans.XmlID)get_store().find_attribute_user(ID$8);
if (target == null)
{
target = (org.apache.xmlbeans.XmlID)get_store().add_attribute_user(ID$8);
}
target.set(id);
}
}
/**
* Unsets the "id" attribute
*/
public void unsetId()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(ID$8);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy