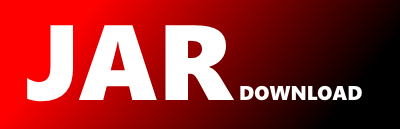
net.opengis.swe.x101.impl.BinaryBlockDocumentImpl Maven / Gradle / Ivy
/*
* An XML document type.
* Localname: BinaryBlock
* Namespace: http://www.opengis.net/swe/1.0.1
* Java type: net.opengis.swe.x101.BinaryBlockDocument
*
* Automatically generated - do not modify.
*/
package net.opengis.swe.x101.impl;
/**
* A document containing one BinaryBlock(@http://www.opengis.net/swe/1.0.1) element.
*
* This is a complex type.
*/
public class BinaryBlockDocumentImpl extends org.apache.xmlbeans.impl.values.XmlComplexContentImpl implements net.opengis.swe.x101.BinaryBlockDocument
{
private static final long serialVersionUID = 1L;
public BinaryBlockDocumentImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
private static final javax.xml.namespace.QName BINARYBLOCK$0 =
new javax.xml.namespace.QName("http://www.opengis.net/swe/1.0.1", "BinaryBlock");
/**
* Gets the "BinaryBlock" element
*/
public net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock getBinaryBlock()
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock target = null;
target = (net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock)get_store().find_element_user(BINARYBLOCK$0, 0);
if (target == null)
{
return null;
}
return target;
}
}
/**
* Sets the "BinaryBlock" element
*/
public void setBinaryBlock(net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock binaryBlock)
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock target = null;
target = (net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock)get_store().find_element_user(BINARYBLOCK$0, 0);
if (target == null)
{
target = (net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock)get_store().add_element_user(BINARYBLOCK$0);
}
target.set(binaryBlock);
}
}
/**
* Appends and returns a new empty "BinaryBlock" element
*/
public net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock addNewBinaryBlock()
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock target = null;
target = (net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock)get_store().add_element_user(BINARYBLOCK$0);
return target;
}
}
/**
* An XML BinaryBlock(@http://www.opengis.net/swe/1.0.1).
*
* This is a complex type.
*/
public static class BinaryBlockImpl extends net.opengis.swe.x101.impl.AbstractEncodingTypeImpl implements net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock
{
private static final long serialVersionUID = 1L;
public BinaryBlockImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
private static final javax.xml.namespace.QName MEMBER$0 =
new javax.xml.namespace.QName("http://www.opengis.net/swe/1.0.1", "member");
private static final javax.xml.namespace.QName BYTELENGTH$2 =
new javax.xml.namespace.QName("", "byteLength");
private static final javax.xml.namespace.QName BYTEENCODING$4 =
new javax.xml.namespace.QName("", "byteEncoding");
private static final javax.xml.namespace.QName BYTEORDER$6 =
new javax.xml.namespace.QName("", "byteOrder");
/**
* Gets array of all "member" elements
*/
public net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock.Member[] getMemberArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(MEMBER$0, targetList);
net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock.Member[] result = new net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock.Member[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "member" element
*/
public net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock.Member getMemberArray(int i)
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock.Member target = null;
target = (net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock.Member)get_store().find_element_user(MEMBER$0, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "member" element
*/
public int sizeOfMemberArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(MEMBER$0);
}
}
/**
* Sets array of all "member" element
*/
public void setMemberArray(net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock.Member[] memberArray)
{
synchronized (monitor())
{
check_orphaned();
arraySetterHelper(memberArray, MEMBER$0);
}
}
/**
* Sets ith "member" element
*/
public void setMemberArray(int i, net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock.Member member)
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock.Member target = null;
target = (net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock.Member)get_store().find_element_user(MEMBER$0, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
target.set(member);
}
}
/**
* Inserts and returns a new empty value (as xml) as the ith "member" element
*/
public net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock.Member insertNewMember(int i)
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock.Member target = null;
target = (net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock.Member)get_store().insert_element_user(MEMBER$0, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "member" element
*/
public net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock.Member addNewMember()
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock.Member target = null;
target = (net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock.Member)get_store().add_element_user(MEMBER$0);
return target;
}
}
/**
* Removes the ith "member" element
*/
public void removeMember(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(MEMBER$0, i);
}
}
/**
* Gets the "byteLength" attribute
*/
public java.math.BigInteger getByteLength()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(BYTELENGTH$2);
if (target == null)
{
return null;
}
return target.getBigIntegerValue();
}
}
/**
* Gets (as xml) the "byteLength" attribute
*/
public org.apache.xmlbeans.XmlPositiveInteger xgetByteLength()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlPositiveInteger target = null;
target = (org.apache.xmlbeans.XmlPositiveInteger)get_store().find_attribute_user(BYTELENGTH$2);
return target;
}
}
/**
* True if has "byteLength" attribute
*/
public boolean isSetByteLength()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(BYTELENGTH$2) != null;
}
}
/**
* Sets the "byteLength" attribute
*/
public void setByteLength(java.math.BigInteger byteLength)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(BYTELENGTH$2);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(BYTELENGTH$2);
}
target.setBigIntegerValue(byteLength);
}
}
/**
* Sets (as xml) the "byteLength" attribute
*/
public void xsetByteLength(org.apache.xmlbeans.XmlPositiveInteger byteLength)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlPositiveInteger target = null;
target = (org.apache.xmlbeans.XmlPositiveInteger)get_store().find_attribute_user(BYTELENGTH$2);
if (target == null)
{
target = (org.apache.xmlbeans.XmlPositiveInteger)get_store().add_attribute_user(BYTELENGTH$2);
}
target.set(byteLength);
}
}
/**
* Unsets the "byteLength" attribute
*/
public void unsetByteLength()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(BYTELENGTH$2);
}
}
/**
* Gets the "byteEncoding" attribute
*/
public net.opengis.swe.x101.ByteEncoding.Enum getByteEncoding()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(BYTEENCODING$4);
if (target == null)
{
return null;
}
return (net.opengis.swe.x101.ByteEncoding.Enum)target.getEnumValue();
}
}
/**
* Gets (as xml) the "byteEncoding" attribute
*/
public net.opengis.swe.x101.ByteEncoding xgetByteEncoding()
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.ByteEncoding target = null;
target = (net.opengis.swe.x101.ByteEncoding)get_store().find_attribute_user(BYTEENCODING$4);
return target;
}
}
/**
* Sets the "byteEncoding" attribute
*/
public void setByteEncoding(net.opengis.swe.x101.ByteEncoding.Enum byteEncoding)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(BYTEENCODING$4);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(BYTEENCODING$4);
}
target.setEnumValue(byteEncoding);
}
}
/**
* Sets (as xml) the "byteEncoding" attribute
*/
public void xsetByteEncoding(net.opengis.swe.x101.ByteEncoding byteEncoding)
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.ByteEncoding target = null;
target = (net.opengis.swe.x101.ByteEncoding)get_store().find_attribute_user(BYTEENCODING$4);
if (target == null)
{
target = (net.opengis.swe.x101.ByteEncoding)get_store().add_attribute_user(BYTEENCODING$4);
}
target.set(byteEncoding);
}
}
/**
* Gets the "byteOrder" attribute
*/
public net.opengis.swe.x101.ByteOrder.Enum getByteOrder()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(BYTEORDER$6);
if (target == null)
{
return null;
}
return (net.opengis.swe.x101.ByteOrder.Enum)target.getEnumValue();
}
}
/**
* Gets (as xml) the "byteOrder" attribute
*/
public net.opengis.swe.x101.ByteOrder xgetByteOrder()
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.ByteOrder target = null;
target = (net.opengis.swe.x101.ByteOrder)get_store().find_attribute_user(BYTEORDER$6);
return target;
}
}
/**
* Sets the "byteOrder" attribute
*/
public void setByteOrder(net.opengis.swe.x101.ByteOrder.Enum byteOrder)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(BYTEORDER$6);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(BYTEORDER$6);
}
target.setEnumValue(byteOrder);
}
}
/**
* Sets (as xml) the "byteOrder" attribute
*/
public void xsetByteOrder(net.opengis.swe.x101.ByteOrder byteOrder)
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.ByteOrder target = null;
target = (net.opengis.swe.x101.ByteOrder)get_store().find_attribute_user(BYTEORDER$6);
if (target == null)
{
target = (net.opengis.swe.x101.ByteOrder)get_store().add_attribute_user(BYTEORDER$6);
}
target.set(byteOrder);
}
}
/**
* An XML member(@http://www.opengis.net/swe/1.0.1).
*
* This is a complex type.
*/
public static class MemberImpl extends org.apache.xmlbeans.impl.values.XmlComplexContentImpl implements net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock.Member
{
private static final long serialVersionUID = 1L;
public MemberImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
private static final javax.xml.namespace.QName COMPONENT$0 =
new javax.xml.namespace.QName("http://www.opengis.net/swe/1.0.1", "Component");
private static final javax.xml.namespace.QName BLOCK$2 =
new javax.xml.namespace.QName("http://www.opengis.net/swe/1.0.1", "Block");
/**
* Gets the "Component" element
*/
public net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock.Member.Component getComponent()
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock.Member.Component target = null;
target = (net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock.Member.Component)get_store().find_element_user(COMPONENT$0, 0);
if (target == null)
{
return null;
}
return target;
}
}
/**
* True if has "Component" element
*/
public boolean isSetComponent()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(COMPONENT$0) != 0;
}
}
/**
* Sets the "Component" element
*/
public void setComponent(net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock.Member.Component component)
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock.Member.Component target = null;
target = (net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock.Member.Component)get_store().find_element_user(COMPONENT$0, 0);
if (target == null)
{
target = (net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock.Member.Component)get_store().add_element_user(COMPONENT$0);
}
target.set(component);
}
}
/**
* Appends and returns a new empty "Component" element
*/
public net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock.Member.Component addNewComponent()
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock.Member.Component target = null;
target = (net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock.Member.Component)get_store().add_element_user(COMPONENT$0);
return target;
}
}
/**
* Unsets the "Component" element
*/
public void unsetComponent()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(COMPONENT$0, 0);
}
}
/**
* Gets the "Block" element
*/
public net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock.Member.Block getBlock()
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock.Member.Block target = null;
target = (net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock.Member.Block)get_store().find_element_user(BLOCK$2, 0);
if (target == null)
{
return null;
}
return target;
}
}
/**
* True if has "Block" element
*/
public boolean isSetBlock()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(BLOCK$2) != 0;
}
}
/**
* Sets the "Block" element
*/
public void setBlock(net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock.Member.Block block)
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock.Member.Block target = null;
target = (net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock.Member.Block)get_store().find_element_user(BLOCK$2, 0);
if (target == null)
{
target = (net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock.Member.Block)get_store().add_element_user(BLOCK$2);
}
target.set(block);
}
}
/**
* Appends and returns a new empty "Block" element
*/
public net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock.Member.Block addNewBlock()
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock.Member.Block target = null;
target = (net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock.Member.Block)get_store().add_element_user(BLOCK$2);
return target;
}
}
/**
* Unsets the "Block" element
*/
public void unsetBlock()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(BLOCK$2, 0);
}
}
/**
* An XML Component(@http://www.opengis.net/swe/1.0.1).
*
* This is a complex type.
*/
public static class ComponentImpl extends org.apache.xmlbeans.impl.values.XmlComplexContentImpl implements net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock.Member.Component
{
private static final long serialVersionUID = 1L;
public ComponentImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
private static final javax.xml.namespace.QName REF$0 =
new javax.xml.namespace.QName("", "ref");
private static final javax.xml.namespace.QName DATATYPE$2 =
new javax.xml.namespace.QName("", "dataType");
private static final javax.xml.namespace.QName SIGNIFICANTBITS$4 =
new javax.xml.namespace.QName("", "significantBits");
private static final javax.xml.namespace.QName BITLENGTH$6 =
new javax.xml.namespace.QName("", "bitLength");
private static final javax.xml.namespace.QName PADDINGBITSBEFORE$8 =
new javax.xml.namespace.QName("", "paddingBits-before");
private static final javax.xml.namespace.QName PADDINGBITSAFTER$10 =
new javax.xml.namespace.QName("", "paddingBits-after");
private static final javax.xml.namespace.QName ENCRYPTION$12 =
new javax.xml.namespace.QName("", "encryption");
/**
* Gets the "ref" attribute
*/
public java.lang.String getRef()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(REF$0);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "ref" attribute
*/
public org.apache.xmlbeans.XmlToken xgetRef()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlToken target = null;
target = (org.apache.xmlbeans.XmlToken)get_store().find_attribute_user(REF$0);
return target;
}
}
/**
* Sets the "ref" attribute
*/
public void setRef(java.lang.String ref)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(REF$0);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(REF$0);
}
target.setStringValue(ref);
}
}
/**
* Sets (as xml) the "ref" attribute
*/
public void xsetRef(org.apache.xmlbeans.XmlToken ref)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlToken target = null;
target = (org.apache.xmlbeans.XmlToken)get_store().find_attribute_user(REF$0);
if (target == null)
{
target = (org.apache.xmlbeans.XmlToken)get_store().add_attribute_user(REF$0);
}
target.set(ref);
}
}
/**
* Gets the "dataType" attribute
*/
public java.lang.String getDataType()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(DATATYPE$2);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "dataType" attribute
*/
public org.apache.xmlbeans.XmlAnyURI xgetDataType()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnyURI target = null;
target = (org.apache.xmlbeans.XmlAnyURI)get_store().find_attribute_user(DATATYPE$2);
return target;
}
}
/**
* True if has "dataType" attribute
*/
public boolean isSetDataType()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(DATATYPE$2) != null;
}
}
/**
* Sets the "dataType" attribute
*/
public void setDataType(java.lang.String dataType)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(DATATYPE$2);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(DATATYPE$2);
}
target.setStringValue(dataType);
}
}
/**
* Sets (as xml) the "dataType" attribute
*/
public void xsetDataType(org.apache.xmlbeans.XmlAnyURI dataType)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnyURI target = null;
target = (org.apache.xmlbeans.XmlAnyURI)get_store().find_attribute_user(DATATYPE$2);
if (target == null)
{
target = (org.apache.xmlbeans.XmlAnyURI)get_store().add_attribute_user(DATATYPE$2);
}
target.set(dataType);
}
}
/**
* Unsets the "dataType" attribute
*/
public void unsetDataType()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(DATATYPE$2);
}
}
/**
* Gets the "significantBits" attribute
*/
public java.math.BigInteger getSignificantBits()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(SIGNIFICANTBITS$4);
if (target == null)
{
return null;
}
return target.getBigIntegerValue();
}
}
/**
* Gets (as xml) the "significantBits" attribute
*/
public org.apache.xmlbeans.XmlPositiveInteger xgetSignificantBits()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlPositiveInteger target = null;
target = (org.apache.xmlbeans.XmlPositiveInteger)get_store().find_attribute_user(SIGNIFICANTBITS$4);
return target;
}
}
/**
* True if has "significantBits" attribute
*/
public boolean isSetSignificantBits()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(SIGNIFICANTBITS$4) != null;
}
}
/**
* Sets the "significantBits" attribute
*/
public void setSignificantBits(java.math.BigInteger significantBits)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(SIGNIFICANTBITS$4);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(SIGNIFICANTBITS$4);
}
target.setBigIntegerValue(significantBits);
}
}
/**
* Sets (as xml) the "significantBits" attribute
*/
public void xsetSignificantBits(org.apache.xmlbeans.XmlPositiveInteger significantBits)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlPositiveInteger target = null;
target = (org.apache.xmlbeans.XmlPositiveInteger)get_store().find_attribute_user(SIGNIFICANTBITS$4);
if (target == null)
{
target = (org.apache.xmlbeans.XmlPositiveInteger)get_store().add_attribute_user(SIGNIFICANTBITS$4);
}
target.set(significantBits);
}
}
/**
* Unsets the "significantBits" attribute
*/
public void unsetSignificantBits()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(SIGNIFICANTBITS$4);
}
}
/**
* Gets the "bitLength" attribute
*/
public java.math.BigInteger getBitLength()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(BITLENGTH$6);
if (target == null)
{
return null;
}
return target.getBigIntegerValue();
}
}
/**
* Gets (as xml) the "bitLength" attribute
*/
public org.apache.xmlbeans.XmlPositiveInteger xgetBitLength()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlPositiveInteger target = null;
target = (org.apache.xmlbeans.XmlPositiveInteger)get_store().find_attribute_user(BITLENGTH$6);
return target;
}
}
/**
* True if has "bitLength" attribute
*/
public boolean isSetBitLength()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(BITLENGTH$6) != null;
}
}
/**
* Sets the "bitLength" attribute
*/
public void setBitLength(java.math.BigInteger bitLength)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(BITLENGTH$6);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(BITLENGTH$6);
}
target.setBigIntegerValue(bitLength);
}
}
/**
* Sets (as xml) the "bitLength" attribute
*/
public void xsetBitLength(org.apache.xmlbeans.XmlPositiveInteger bitLength)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlPositiveInteger target = null;
target = (org.apache.xmlbeans.XmlPositiveInteger)get_store().find_attribute_user(BITLENGTH$6);
if (target == null)
{
target = (org.apache.xmlbeans.XmlPositiveInteger)get_store().add_attribute_user(BITLENGTH$6);
}
target.set(bitLength);
}
}
/**
* Unsets the "bitLength" attribute
*/
public void unsetBitLength()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(BITLENGTH$6);
}
}
/**
* Gets the "paddingBits-before" attribute
*/
public java.math.BigInteger getPaddingBitsBefore()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(PADDINGBITSBEFORE$8);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_default_attribute_value(PADDINGBITSBEFORE$8);
}
if (target == null)
{
return null;
}
return target.getBigIntegerValue();
}
}
/**
* Gets (as xml) the "paddingBits-before" attribute
*/
public org.apache.xmlbeans.XmlNonNegativeInteger xgetPaddingBitsBefore()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlNonNegativeInteger target = null;
target = (org.apache.xmlbeans.XmlNonNegativeInteger)get_store().find_attribute_user(PADDINGBITSBEFORE$8);
if (target == null)
{
target = (org.apache.xmlbeans.XmlNonNegativeInteger)get_default_attribute_value(PADDINGBITSBEFORE$8);
}
return target;
}
}
/**
* True if has "paddingBits-before" attribute
*/
public boolean isSetPaddingBitsBefore()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(PADDINGBITSBEFORE$8) != null;
}
}
/**
* Sets the "paddingBits-before" attribute
*/
public void setPaddingBitsBefore(java.math.BigInteger paddingBitsBefore)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(PADDINGBITSBEFORE$8);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(PADDINGBITSBEFORE$8);
}
target.setBigIntegerValue(paddingBitsBefore);
}
}
/**
* Sets (as xml) the "paddingBits-before" attribute
*/
public void xsetPaddingBitsBefore(org.apache.xmlbeans.XmlNonNegativeInteger paddingBitsBefore)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlNonNegativeInteger target = null;
target = (org.apache.xmlbeans.XmlNonNegativeInteger)get_store().find_attribute_user(PADDINGBITSBEFORE$8);
if (target == null)
{
target = (org.apache.xmlbeans.XmlNonNegativeInteger)get_store().add_attribute_user(PADDINGBITSBEFORE$8);
}
target.set(paddingBitsBefore);
}
}
/**
* Unsets the "paddingBits-before" attribute
*/
public void unsetPaddingBitsBefore()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(PADDINGBITSBEFORE$8);
}
}
/**
* Gets the "paddingBits-after" attribute
*/
public java.math.BigInteger getPaddingBitsAfter()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(PADDINGBITSAFTER$10);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_default_attribute_value(PADDINGBITSAFTER$10);
}
if (target == null)
{
return null;
}
return target.getBigIntegerValue();
}
}
/**
* Gets (as xml) the "paddingBits-after" attribute
*/
public org.apache.xmlbeans.XmlNonNegativeInteger xgetPaddingBitsAfter()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlNonNegativeInteger target = null;
target = (org.apache.xmlbeans.XmlNonNegativeInteger)get_store().find_attribute_user(PADDINGBITSAFTER$10);
if (target == null)
{
target = (org.apache.xmlbeans.XmlNonNegativeInteger)get_default_attribute_value(PADDINGBITSAFTER$10);
}
return target;
}
}
/**
* True if has "paddingBits-after" attribute
*/
public boolean isSetPaddingBitsAfter()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(PADDINGBITSAFTER$10) != null;
}
}
/**
* Sets the "paddingBits-after" attribute
*/
public void setPaddingBitsAfter(java.math.BigInteger paddingBitsAfter)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(PADDINGBITSAFTER$10);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(PADDINGBITSAFTER$10);
}
target.setBigIntegerValue(paddingBitsAfter);
}
}
/**
* Sets (as xml) the "paddingBits-after" attribute
*/
public void xsetPaddingBitsAfter(org.apache.xmlbeans.XmlNonNegativeInteger paddingBitsAfter)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlNonNegativeInteger target = null;
target = (org.apache.xmlbeans.XmlNonNegativeInteger)get_store().find_attribute_user(PADDINGBITSAFTER$10);
if (target == null)
{
target = (org.apache.xmlbeans.XmlNonNegativeInteger)get_store().add_attribute_user(PADDINGBITSAFTER$10);
}
target.set(paddingBitsAfter);
}
}
/**
* Unsets the "paddingBits-after" attribute
*/
public void unsetPaddingBitsAfter()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(PADDINGBITSAFTER$10);
}
}
/**
* Gets the "encryption" attribute
*/
public java.lang.String getEncryption()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(ENCRYPTION$12);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "encryption" attribute
*/
public org.apache.xmlbeans.XmlAnyURI xgetEncryption()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnyURI target = null;
target = (org.apache.xmlbeans.XmlAnyURI)get_store().find_attribute_user(ENCRYPTION$12);
return target;
}
}
/**
* True if has "encryption" attribute
*/
public boolean isSetEncryption()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(ENCRYPTION$12) != null;
}
}
/**
* Sets the "encryption" attribute
*/
public void setEncryption(java.lang.String encryption)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(ENCRYPTION$12);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(ENCRYPTION$12);
}
target.setStringValue(encryption);
}
}
/**
* Sets (as xml) the "encryption" attribute
*/
public void xsetEncryption(org.apache.xmlbeans.XmlAnyURI encryption)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnyURI target = null;
target = (org.apache.xmlbeans.XmlAnyURI)get_store().find_attribute_user(ENCRYPTION$12);
if (target == null)
{
target = (org.apache.xmlbeans.XmlAnyURI)get_store().add_attribute_user(ENCRYPTION$12);
}
target.set(encryption);
}
}
/**
* Unsets the "encryption" attribute
*/
public void unsetEncryption()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(ENCRYPTION$12);
}
}
}
/**
* An XML Block(@http://www.opengis.net/swe/1.0.1).
*
* This is a complex type.
*/
public static class BlockImpl extends org.apache.xmlbeans.impl.values.XmlComplexContentImpl implements net.opengis.swe.x101.BinaryBlockDocument.BinaryBlock.Member.Block
{
private static final long serialVersionUID = 1L;
public BlockImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
private static final javax.xml.namespace.QName REF$0 =
new javax.xml.namespace.QName("", "ref");
private static final javax.xml.namespace.QName BYTELENGTH$2 =
new javax.xml.namespace.QName("", "byteLength");
private static final javax.xml.namespace.QName PADDINGBYTESBEFORE$4 =
new javax.xml.namespace.QName("", "paddingBytes-before");
private static final javax.xml.namespace.QName PADDINGBYTESAFTER$6 =
new javax.xml.namespace.QName("", "paddingBytes-after");
private static final javax.xml.namespace.QName ENCRYPTION$8 =
new javax.xml.namespace.QName("", "encryption");
private static final javax.xml.namespace.QName COMPRESSION$10 =
new javax.xml.namespace.QName("", "compression");
/**
* Gets the "ref" attribute
*/
public java.lang.String getRef()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(REF$0);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "ref" attribute
*/
public org.apache.xmlbeans.XmlToken xgetRef()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlToken target = null;
target = (org.apache.xmlbeans.XmlToken)get_store().find_attribute_user(REF$0);
return target;
}
}
/**
* Sets the "ref" attribute
*/
public void setRef(java.lang.String ref)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(REF$0);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(REF$0);
}
target.setStringValue(ref);
}
}
/**
* Sets (as xml) the "ref" attribute
*/
public void xsetRef(org.apache.xmlbeans.XmlToken ref)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlToken target = null;
target = (org.apache.xmlbeans.XmlToken)get_store().find_attribute_user(REF$0);
if (target == null)
{
target = (org.apache.xmlbeans.XmlToken)get_store().add_attribute_user(REF$0);
}
target.set(ref);
}
}
/**
* Gets the "byteLength" attribute
*/
public java.math.BigInteger getByteLength()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(BYTELENGTH$2);
if (target == null)
{
return null;
}
return target.getBigIntegerValue();
}
}
/**
* Gets (as xml) the "byteLength" attribute
*/
public org.apache.xmlbeans.XmlPositiveInteger xgetByteLength()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlPositiveInteger target = null;
target = (org.apache.xmlbeans.XmlPositiveInteger)get_store().find_attribute_user(BYTELENGTH$2);
return target;
}
}
/**
* True if has "byteLength" attribute
*/
public boolean isSetByteLength()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(BYTELENGTH$2) != null;
}
}
/**
* Sets the "byteLength" attribute
*/
public void setByteLength(java.math.BigInteger byteLength)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(BYTELENGTH$2);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(BYTELENGTH$2);
}
target.setBigIntegerValue(byteLength);
}
}
/**
* Sets (as xml) the "byteLength" attribute
*/
public void xsetByteLength(org.apache.xmlbeans.XmlPositiveInteger byteLength)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlPositiveInteger target = null;
target = (org.apache.xmlbeans.XmlPositiveInteger)get_store().find_attribute_user(BYTELENGTH$2);
if (target == null)
{
target = (org.apache.xmlbeans.XmlPositiveInteger)get_store().add_attribute_user(BYTELENGTH$2);
}
target.set(byteLength);
}
}
/**
* Unsets the "byteLength" attribute
*/
public void unsetByteLength()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(BYTELENGTH$2);
}
}
/**
* Gets the "paddingBytes-before" attribute
*/
public java.math.BigInteger getPaddingBytesBefore()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(PADDINGBYTESBEFORE$4);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_default_attribute_value(PADDINGBYTESBEFORE$4);
}
if (target == null)
{
return null;
}
return target.getBigIntegerValue();
}
}
/**
* Gets (as xml) the "paddingBytes-before" attribute
*/
public org.apache.xmlbeans.XmlNonNegativeInteger xgetPaddingBytesBefore()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlNonNegativeInteger target = null;
target = (org.apache.xmlbeans.XmlNonNegativeInteger)get_store().find_attribute_user(PADDINGBYTESBEFORE$4);
if (target == null)
{
target = (org.apache.xmlbeans.XmlNonNegativeInteger)get_default_attribute_value(PADDINGBYTESBEFORE$4);
}
return target;
}
}
/**
* True if has "paddingBytes-before" attribute
*/
public boolean isSetPaddingBytesBefore()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(PADDINGBYTESBEFORE$4) != null;
}
}
/**
* Sets the "paddingBytes-before" attribute
*/
public void setPaddingBytesBefore(java.math.BigInteger paddingBytesBefore)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(PADDINGBYTESBEFORE$4);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(PADDINGBYTESBEFORE$4);
}
target.setBigIntegerValue(paddingBytesBefore);
}
}
/**
* Sets (as xml) the "paddingBytes-before" attribute
*/
public void xsetPaddingBytesBefore(org.apache.xmlbeans.XmlNonNegativeInteger paddingBytesBefore)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlNonNegativeInteger target = null;
target = (org.apache.xmlbeans.XmlNonNegativeInteger)get_store().find_attribute_user(PADDINGBYTESBEFORE$4);
if (target == null)
{
target = (org.apache.xmlbeans.XmlNonNegativeInteger)get_store().add_attribute_user(PADDINGBYTESBEFORE$4);
}
target.set(paddingBytesBefore);
}
}
/**
* Unsets the "paddingBytes-before" attribute
*/
public void unsetPaddingBytesBefore()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(PADDINGBYTESBEFORE$4);
}
}
/**
* Gets the "paddingBytes-after" attribute
*/
public java.math.BigInteger getPaddingBytesAfter()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(PADDINGBYTESAFTER$6);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_default_attribute_value(PADDINGBYTESAFTER$6);
}
if (target == null)
{
return null;
}
return target.getBigIntegerValue();
}
}
/**
* Gets (as xml) the "paddingBytes-after" attribute
*/
public org.apache.xmlbeans.XmlNonNegativeInteger xgetPaddingBytesAfter()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlNonNegativeInteger target = null;
target = (org.apache.xmlbeans.XmlNonNegativeInteger)get_store().find_attribute_user(PADDINGBYTESAFTER$6);
if (target == null)
{
target = (org.apache.xmlbeans.XmlNonNegativeInteger)get_default_attribute_value(PADDINGBYTESAFTER$6);
}
return target;
}
}
/**
* True if has "paddingBytes-after" attribute
*/
public boolean isSetPaddingBytesAfter()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(PADDINGBYTESAFTER$6) != null;
}
}
/**
* Sets the "paddingBytes-after" attribute
*/
public void setPaddingBytesAfter(java.math.BigInteger paddingBytesAfter)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(PADDINGBYTESAFTER$6);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(PADDINGBYTESAFTER$6);
}
target.setBigIntegerValue(paddingBytesAfter);
}
}
/**
* Sets (as xml) the "paddingBytes-after" attribute
*/
public void xsetPaddingBytesAfter(org.apache.xmlbeans.XmlNonNegativeInteger paddingBytesAfter)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlNonNegativeInteger target = null;
target = (org.apache.xmlbeans.XmlNonNegativeInteger)get_store().find_attribute_user(PADDINGBYTESAFTER$6);
if (target == null)
{
target = (org.apache.xmlbeans.XmlNonNegativeInteger)get_store().add_attribute_user(PADDINGBYTESAFTER$6);
}
target.set(paddingBytesAfter);
}
}
/**
* Unsets the "paddingBytes-after" attribute
*/
public void unsetPaddingBytesAfter()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(PADDINGBYTESAFTER$6);
}
}
/**
* Gets the "encryption" attribute
*/
public java.lang.String getEncryption()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(ENCRYPTION$8);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "encryption" attribute
*/
public org.apache.xmlbeans.XmlAnyURI xgetEncryption()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnyURI target = null;
target = (org.apache.xmlbeans.XmlAnyURI)get_store().find_attribute_user(ENCRYPTION$8);
return target;
}
}
/**
* True if has "encryption" attribute
*/
public boolean isSetEncryption()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(ENCRYPTION$8) != null;
}
}
/**
* Sets the "encryption" attribute
*/
public void setEncryption(java.lang.String encryption)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(ENCRYPTION$8);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(ENCRYPTION$8);
}
target.setStringValue(encryption);
}
}
/**
* Sets (as xml) the "encryption" attribute
*/
public void xsetEncryption(org.apache.xmlbeans.XmlAnyURI encryption)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnyURI target = null;
target = (org.apache.xmlbeans.XmlAnyURI)get_store().find_attribute_user(ENCRYPTION$8);
if (target == null)
{
target = (org.apache.xmlbeans.XmlAnyURI)get_store().add_attribute_user(ENCRYPTION$8);
}
target.set(encryption);
}
}
/**
* Unsets the "encryption" attribute
*/
public void unsetEncryption()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(ENCRYPTION$8);
}
}
/**
* Gets the "compression" attribute
*/
public java.lang.String getCompression()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(COMPRESSION$10);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "compression" attribute
*/
public org.apache.xmlbeans.XmlAnyURI xgetCompression()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnyURI target = null;
target = (org.apache.xmlbeans.XmlAnyURI)get_store().find_attribute_user(COMPRESSION$10);
return target;
}
}
/**
* True if has "compression" attribute
*/
public boolean isSetCompression()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(COMPRESSION$10) != null;
}
}
/**
* Sets the "compression" attribute
*/
public void setCompression(java.lang.String compression)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(COMPRESSION$10);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(COMPRESSION$10);
}
target.setStringValue(compression);
}
}
/**
* Sets (as xml) the "compression" attribute
*/
public void xsetCompression(org.apache.xmlbeans.XmlAnyURI compression)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnyURI target = null;
target = (org.apache.xmlbeans.XmlAnyURI)get_store().find_attribute_user(COMPRESSION$10);
if (target == null)
{
target = (org.apache.xmlbeans.XmlAnyURI)get_store().add_attribute_user(COMPRESSION$10);
}
target.set(compression);
}
}
/**
* Unsets the "compression" attribute
*/
public void unsetCompression()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(COMPRESSION$10);
}
}
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy