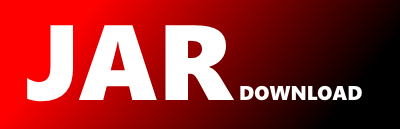
net.opengis.swe.x101.impl.QuantityRangeDocumentImpl Maven / Gradle / Ivy
/*
* An XML document type.
* Localname: QuantityRange
* Namespace: http://www.opengis.net/swe/1.0.1
* Java type: net.opengis.swe.x101.QuantityRangeDocument
*
* Automatically generated - do not modify.
*/
package net.opengis.swe.x101.impl;
/**
* A document containing one QuantityRange(@http://www.opengis.net/swe/1.0.1) element.
*
* This is a complex type.
*/
public class QuantityRangeDocumentImpl extends org.apache.xmlbeans.impl.values.XmlComplexContentImpl implements net.opengis.swe.x101.QuantityRangeDocument
{
private static final long serialVersionUID = 1L;
public QuantityRangeDocumentImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
private static final javax.xml.namespace.QName QUANTITYRANGE$0 =
new javax.xml.namespace.QName("http://www.opengis.net/swe/1.0.1", "QuantityRange");
/**
* Gets the "QuantityRange" element
*/
public net.opengis.swe.x101.QuantityRangeDocument.QuantityRange getQuantityRange()
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.QuantityRangeDocument.QuantityRange target = null;
target = (net.opengis.swe.x101.QuantityRangeDocument.QuantityRange)get_store().find_element_user(QUANTITYRANGE$0, 0);
if (target == null)
{
return null;
}
return target;
}
}
/**
* Sets the "QuantityRange" element
*/
public void setQuantityRange(net.opengis.swe.x101.QuantityRangeDocument.QuantityRange quantityRange)
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.QuantityRangeDocument.QuantityRange target = null;
target = (net.opengis.swe.x101.QuantityRangeDocument.QuantityRange)get_store().find_element_user(QUANTITYRANGE$0, 0);
if (target == null)
{
target = (net.opengis.swe.x101.QuantityRangeDocument.QuantityRange)get_store().add_element_user(QUANTITYRANGE$0);
}
target.set(quantityRange);
}
}
/**
* Appends and returns a new empty "QuantityRange" element
*/
public net.opengis.swe.x101.QuantityRangeDocument.QuantityRange addNewQuantityRange()
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.QuantityRangeDocument.QuantityRange target = null;
target = (net.opengis.swe.x101.QuantityRangeDocument.QuantityRange)get_store().add_element_user(QUANTITYRANGE$0);
return target;
}
}
/**
* An XML QuantityRange(@http://www.opengis.net/swe/1.0.1).
*
* This is a complex type.
*/
public static class QuantityRangeImpl extends net.opengis.swe.x101.impl.AbstractDataComponentTypeImpl implements net.opengis.swe.x101.QuantityRangeDocument.QuantityRange
{
private static final long serialVersionUID = 1L;
public QuantityRangeImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
private static final javax.xml.namespace.QName UOM$0 =
new javax.xml.namespace.QName("http://www.opengis.net/swe/1.0.1", "uom");
private static final javax.xml.namespace.QName CONSTRAINT$2 =
new javax.xml.namespace.QName("http://www.opengis.net/swe/1.0.1", "constraint");
private static final javax.xml.namespace.QName QUALITY$4 =
new javax.xml.namespace.QName("http://www.opengis.net/swe/1.0.1", "quality");
private static final javax.xml.namespace.QName VALUE$6 =
new javax.xml.namespace.QName("http://www.opengis.net/swe/1.0.1", "value");
private static final javax.xml.namespace.QName REFERENCEFRAME$8 =
new javax.xml.namespace.QName("", "referenceFrame");
private static final javax.xml.namespace.QName AXISID$10 =
new javax.xml.namespace.QName("", "axisID");
/**
* Gets the "uom" element
*/
public net.opengis.swe.x101.UomPropertyType getUom()
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.UomPropertyType target = null;
target = (net.opengis.swe.x101.UomPropertyType)get_store().find_element_user(UOM$0, 0);
if (target == null)
{
return null;
}
return target;
}
}
/**
* True if has "uom" element
*/
public boolean isSetUom()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(UOM$0) != 0;
}
}
/**
* Sets the "uom" element
*/
public void setUom(net.opengis.swe.x101.UomPropertyType uom)
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.UomPropertyType target = null;
target = (net.opengis.swe.x101.UomPropertyType)get_store().find_element_user(UOM$0, 0);
if (target == null)
{
target = (net.opengis.swe.x101.UomPropertyType)get_store().add_element_user(UOM$0);
}
target.set(uom);
}
}
/**
* Appends and returns a new empty "uom" element
*/
public net.opengis.swe.x101.UomPropertyType addNewUom()
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.UomPropertyType target = null;
target = (net.opengis.swe.x101.UomPropertyType)get_store().add_element_user(UOM$0);
return target;
}
}
/**
* Unsets the "uom" element
*/
public void unsetUom()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(UOM$0, 0);
}
}
/**
* Gets the "constraint" element
*/
public net.opengis.swe.x101.AllowedValuesPropertyType getConstraint()
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.AllowedValuesPropertyType target = null;
target = (net.opengis.swe.x101.AllowedValuesPropertyType)get_store().find_element_user(CONSTRAINT$2, 0);
if (target == null)
{
return null;
}
return target;
}
}
/**
* True if has "constraint" element
*/
public boolean isSetConstraint()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(CONSTRAINT$2) != 0;
}
}
/**
* Sets the "constraint" element
*/
public void setConstraint(net.opengis.swe.x101.AllowedValuesPropertyType constraint)
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.AllowedValuesPropertyType target = null;
target = (net.opengis.swe.x101.AllowedValuesPropertyType)get_store().find_element_user(CONSTRAINT$2, 0);
if (target == null)
{
target = (net.opengis.swe.x101.AllowedValuesPropertyType)get_store().add_element_user(CONSTRAINT$2);
}
target.set(constraint);
}
}
/**
* Appends and returns a new empty "constraint" element
*/
public net.opengis.swe.x101.AllowedValuesPropertyType addNewConstraint()
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.AllowedValuesPropertyType target = null;
target = (net.opengis.swe.x101.AllowedValuesPropertyType)get_store().add_element_user(CONSTRAINT$2);
return target;
}
}
/**
* Unsets the "constraint" element
*/
public void unsetConstraint()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(CONSTRAINT$2, 0);
}
}
/**
* Gets array of all "quality" elements
*/
public net.opengis.swe.x101.QualityPropertyType[] getQualityArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(QUALITY$4, targetList);
net.opengis.swe.x101.QualityPropertyType[] result = new net.opengis.swe.x101.QualityPropertyType[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "quality" element
*/
public net.opengis.swe.x101.QualityPropertyType getQualityArray(int i)
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.QualityPropertyType target = null;
target = (net.opengis.swe.x101.QualityPropertyType)get_store().find_element_user(QUALITY$4, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "quality" element
*/
public int sizeOfQualityArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(QUALITY$4);
}
}
/**
* Sets array of all "quality" element
*/
public void setQualityArray(net.opengis.swe.x101.QualityPropertyType[] qualityArray)
{
synchronized (monitor())
{
check_orphaned();
arraySetterHelper(qualityArray, QUALITY$4);
}
}
/**
* Sets ith "quality" element
*/
public void setQualityArray(int i, net.opengis.swe.x101.QualityPropertyType quality)
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.QualityPropertyType target = null;
target = (net.opengis.swe.x101.QualityPropertyType)get_store().find_element_user(QUALITY$4, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
target.set(quality);
}
}
/**
* Inserts and returns a new empty value (as xml) as the ith "quality" element
*/
public net.opengis.swe.x101.QualityPropertyType insertNewQuality(int i)
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.QualityPropertyType target = null;
target = (net.opengis.swe.x101.QualityPropertyType)get_store().insert_element_user(QUALITY$4, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "quality" element
*/
public net.opengis.swe.x101.QualityPropertyType addNewQuality()
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.QualityPropertyType target = null;
target = (net.opengis.swe.x101.QualityPropertyType)get_store().add_element_user(QUALITY$4);
return target;
}
}
/**
* Removes the ith "quality" element
*/
public void removeQuality(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(QUALITY$4, i);
}
}
/**
* Gets the "value" element
*/
public java.util.List getValue()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_element_user(VALUE$6, 0);
if (target == null)
{
return null;
}
return target.getListValue();
}
}
/**
* Gets (as xml) the "value" element
*/
public net.opengis.swe.x101.DecimalPair xgetValue()
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.DecimalPair target = null;
target = (net.opengis.swe.x101.DecimalPair)get_store().find_element_user(VALUE$6, 0);
return target;
}
}
/**
* True if has "value" element
*/
public boolean isSetValue()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(VALUE$6) != 0;
}
}
/**
* Sets the "value" element
*/
public void setValue(java.util.List value)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_element_user(VALUE$6, 0);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_element_user(VALUE$6);
}
target.setListValue(value);
}
}
/**
* Sets (as xml) the "value" element
*/
public void xsetValue(net.opengis.swe.x101.DecimalPair value)
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.DecimalPair target = null;
target = (net.opengis.swe.x101.DecimalPair)get_store().find_element_user(VALUE$6, 0);
if (target == null)
{
target = (net.opengis.swe.x101.DecimalPair)get_store().add_element_user(VALUE$6);
}
target.set(value);
}
}
/**
* Unsets the "value" element
*/
public void unsetValue()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(VALUE$6, 0);
}
}
/**
* Gets the "referenceFrame" attribute
*/
public java.lang.String getReferenceFrame()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(REFERENCEFRAME$8);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "referenceFrame" attribute
*/
public org.apache.xmlbeans.XmlAnyURI xgetReferenceFrame()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnyURI target = null;
target = (org.apache.xmlbeans.XmlAnyURI)get_store().find_attribute_user(REFERENCEFRAME$8);
return target;
}
}
/**
* True if has "referenceFrame" attribute
*/
public boolean isSetReferenceFrame()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(REFERENCEFRAME$8) != null;
}
}
/**
* Sets the "referenceFrame" attribute
*/
public void setReferenceFrame(java.lang.String referenceFrame)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(REFERENCEFRAME$8);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(REFERENCEFRAME$8);
}
target.setStringValue(referenceFrame);
}
}
/**
* Sets (as xml) the "referenceFrame" attribute
*/
public void xsetReferenceFrame(org.apache.xmlbeans.XmlAnyURI referenceFrame)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlAnyURI target = null;
target = (org.apache.xmlbeans.XmlAnyURI)get_store().find_attribute_user(REFERENCEFRAME$8);
if (target == null)
{
target = (org.apache.xmlbeans.XmlAnyURI)get_store().add_attribute_user(REFERENCEFRAME$8);
}
target.set(referenceFrame);
}
}
/**
* Unsets the "referenceFrame" attribute
*/
public void unsetReferenceFrame()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(REFERENCEFRAME$8);
}
}
/**
* Gets the "axisID" attribute
*/
public java.lang.String getAxisID()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(AXISID$10);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "axisID" attribute
*/
public org.apache.xmlbeans.XmlToken xgetAxisID()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlToken target = null;
target = (org.apache.xmlbeans.XmlToken)get_store().find_attribute_user(AXISID$10);
return target;
}
}
/**
* True if has "axisID" attribute
*/
public boolean isSetAxisID()
{
synchronized (monitor())
{
check_orphaned();
return get_store().find_attribute_user(AXISID$10) != null;
}
}
/**
* Sets the "axisID" attribute
*/
public void setAxisID(java.lang.String axisID)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(AXISID$10);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(AXISID$10);
}
target.setStringValue(axisID);
}
}
/**
* Sets (as xml) the "axisID" attribute
*/
public void xsetAxisID(org.apache.xmlbeans.XmlToken axisID)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlToken target = null;
target = (org.apache.xmlbeans.XmlToken)get_store().find_attribute_user(AXISID$10);
if (target == null)
{
target = (org.apache.xmlbeans.XmlToken)get_store().add_attribute_user(AXISID$10);
}
target.set(axisID);
}
}
/**
* Unsets the "axisID" attribute
*/
public void unsetAxisID()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_attribute(AXISID$10);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy