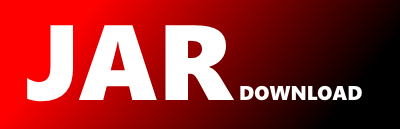
net.opengis.swe.x101.impl.TextBlockDocumentImpl Maven / Gradle / Ivy
/*
* An XML document type.
* Localname: TextBlock
* Namespace: http://www.opengis.net/swe/1.0.1
* Java type: net.opengis.swe.x101.TextBlockDocument
*
* Automatically generated - do not modify.
*/
package net.opengis.swe.x101.impl;
/**
* A document containing one TextBlock(@http://www.opengis.net/swe/1.0.1) element.
*
* This is a complex type.
*/
public class TextBlockDocumentImpl extends org.apache.xmlbeans.impl.values.XmlComplexContentImpl implements net.opengis.swe.x101.TextBlockDocument
{
private static final long serialVersionUID = 1L;
public TextBlockDocumentImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
private static final javax.xml.namespace.QName TEXTBLOCK$0 =
new javax.xml.namespace.QName("http://www.opengis.net/swe/1.0.1", "TextBlock");
/**
* Gets the "TextBlock" element
*/
public net.opengis.swe.x101.TextBlockDocument.TextBlock getTextBlock()
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.TextBlockDocument.TextBlock target = null;
target = (net.opengis.swe.x101.TextBlockDocument.TextBlock)get_store().find_element_user(TEXTBLOCK$0, 0);
if (target == null)
{
return null;
}
return target;
}
}
/**
* Sets the "TextBlock" element
*/
public void setTextBlock(net.opengis.swe.x101.TextBlockDocument.TextBlock textBlock)
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.TextBlockDocument.TextBlock target = null;
target = (net.opengis.swe.x101.TextBlockDocument.TextBlock)get_store().find_element_user(TEXTBLOCK$0, 0);
if (target == null)
{
target = (net.opengis.swe.x101.TextBlockDocument.TextBlock)get_store().add_element_user(TEXTBLOCK$0);
}
target.set(textBlock);
}
}
/**
* Appends and returns a new empty "TextBlock" element
*/
public net.opengis.swe.x101.TextBlockDocument.TextBlock addNewTextBlock()
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.TextBlockDocument.TextBlock target = null;
target = (net.opengis.swe.x101.TextBlockDocument.TextBlock)get_store().add_element_user(TEXTBLOCK$0);
return target;
}
}
/**
* An XML TextBlock(@http://www.opengis.net/swe/1.0.1).
*
* This is a complex type.
*/
public static class TextBlockImpl extends net.opengis.swe.x101.impl.AbstractEncodingTypeImpl implements net.opengis.swe.x101.TextBlockDocument.TextBlock
{
private static final long serialVersionUID = 1L;
public TextBlockImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
private static final javax.xml.namespace.QName TOKENSEPARATOR$0 =
new javax.xml.namespace.QName("", "tokenSeparator");
private static final javax.xml.namespace.QName BLOCKSEPARATOR$2 =
new javax.xml.namespace.QName("", "blockSeparator");
private static final javax.xml.namespace.QName DECIMALSEPARATOR$4 =
new javax.xml.namespace.QName("", "decimalSeparator");
/**
* Gets the "tokenSeparator" attribute
*/
public java.lang.String getTokenSeparator()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(TOKENSEPARATOR$0);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "tokenSeparator" attribute
*/
public net.opengis.swe.x101.TextSeparator xgetTokenSeparator()
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.TextSeparator target = null;
target = (net.opengis.swe.x101.TextSeparator)get_store().find_attribute_user(TOKENSEPARATOR$0);
return target;
}
}
/**
* Sets the "tokenSeparator" attribute
*/
public void setTokenSeparator(java.lang.String tokenSeparator)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(TOKENSEPARATOR$0);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(TOKENSEPARATOR$0);
}
target.setStringValue(tokenSeparator);
}
}
/**
* Sets (as xml) the "tokenSeparator" attribute
*/
public void xsetTokenSeparator(net.opengis.swe.x101.TextSeparator tokenSeparator)
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.TextSeparator target = null;
target = (net.opengis.swe.x101.TextSeparator)get_store().find_attribute_user(TOKENSEPARATOR$0);
if (target == null)
{
target = (net.opengis.swe.x101.TextSeparator)get_store().add_attribute_user(TOKENSEPARATOR$0);
}
target.set(tokenSeparator);
}
}
/**
* Gets the "blockSeparator" attribute
*/
public java.lang.String getBlockSeparator()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(BLOCKSEPARATOR$2);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "blockSeparator" attribute
*/
public net.opengis.swe.x101.TextSeparator xgetBlockSeparator()
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.TextSeparator target = null;
target = (net.opengis.swe.x101.TextSeparator)get_store().find_attribute_user(BLOCKSEPARATOR$2);
return target;
}
}
/**
* Sets the "blockSeparator" attribute
*/
public void setBlockSeparator(java.lang.String blockSeparator)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(BLOCKSEPARATOR$2);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(BLOCKSEPARATOR$2);
}
target.setStringValue(blockSeparator);
}
}
/**
* Sets (as xml) the "blockSeparator" attribute
*/
public void xsetBlockSeparator(net.opengis.swe.x101.TextSeparator blockSeparator)
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.TextSeparator target = null;
target = (net.opengis.swe.x101.TextSeparator)get_store().find_attribute_user(BLOCKSEPARATOR$2);
if (target == null)
{
target = (net.opengis.swe.x101.TextSeparator)get_store().add_attribute_user(BLOCKSEPARATOR$2);
}
target.set(blockSeparator);
}
}
/**
* Gets the "decimalSeparator" attribute
*/
public java.lang.String getDecimalSeparator()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(DECIMALSEPARATOR$4);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "decimalSeparator" attribute
*/
public net.opengis.swe.x101.DecimalSeparator xgetDecimalSeparator()
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.DecimalSeparator target = null;
target = (net.opengis.swe.x101.DecimalSeparator)get_store().find_attribute_user(DECIMALSEPARATOR$4);
return target;
}
}
/**
* Sets the "decimalSeparator" attribute
*/
public void setDecimalSeparator(java.lang.String decimalSeparator)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_attribute_user(DECIMALSEPARATOR$4);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_attribute_user(DECIMALSEPARATOR$4);
}
target.setStringValue(decimalSeparator);
}
}
/**
* Sets (as xml) the "decimalSeparator" attribute
*/
public void xsetDecimalSeparator(net.opengis.swe.x101.DecimalSeparator decimalSeparator)
{
synchronized (monitor())
{
check_orphaned();
net.opengis.swe.x101.DecimalSeparator target = null;
target = (net.opengis.swe.x101.DecimalSeparator)get_store().find_attribute_user(DECIMALSEPARATOR$4);
if (target == null)
{
target = (net.opengis.swe.x101.DecimalSeparator)get_store().add_attribute_user(DECIMALSEPARATOR$4);
}
target.set(decimalSeparator);
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy