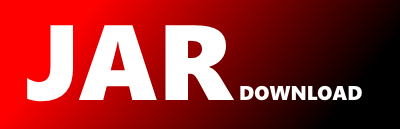
org.n52.sensorthings.Observation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of proxy-dao Show documentation
Show all versions of proxy-dao Show documentation
REST SPI Implementation for SOS Proxy.
package org.n52.sensorthings;
import java.math.BigDecimal;
import java.util.Date;
import com.google.gson.annotations.SerializedName;
import edu.umd.cs.findbugs.annotations.SuppressFBWarnings;
/**
* @author Jan Schulte
*/
public class Observation extends SensorThingsElement {
private Date phenomenonTime;
private BigDecimal result;
private String resultTime;
@SerializedName("[email protected]")
private String datastreamLink;
@SerializedName("[email protected]")
private String featureOfInterestLink;
/**
* @return the phenomenonTime
*/
@SuppressFBWarnings("EI_EXPOSE_REP")
public Date getPhenomenonTime() {
return phenomenonTime;
}
/**
* @param phenomenonTime the phenomenonTime to set
*/
@SuppressFBWarnings("EI_EXPOSE_REP2")
public void setPhenomenonTime(Date phenomenonTime) {
this.phenomenonTime = phenomenonTime;
}
/**
* @return the result
*/
public BigDecimal getResult() {
return result;
}
/**
* @param result the result to set
*/
public void setResult(BigDecimal result) {
this.result = result;
}
/**
* @return the resultTime
*/
public String getResultTime() {
return resultTime;
}
/**
* @param resultTime the resultTime to set
*/
public void setResultTime(String resultTime) {
this.resultTime = resultTime;
}
/**
* @return the datastreamLink
*/
public String getDatastreamLink() {
return datastreamLink;
}
/**
* @param datastreamLink the datastreamLink to set
*/
public void setDatastreamLink(String datastreamLink) {
this.datastreamLink = datastreamLink;
}
/**
* @return the featureOfInterestLink
*/
public String getFeatureOfInterestLink() {
return featureOfInterestLink;
}
/**
* @param featureOfInterestLink the featureOfInterestLink to set
*/
public void setFeatureOfInterestLink(String featureOfInterestLink) {
this.featureOfInterestLink = featureOfInterestLink;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy