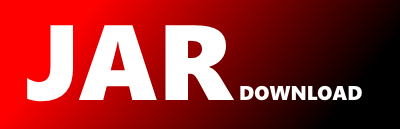
org.nakedobjects.plugins.htmlviewer.html.HtmlForm Maven / Gradle / Ivy
The newest version!
package org.nakedobjects.plugins.htmlviewer.html;
import java.io.PrintWriter;
import org.nakedobjects.metamodel.spec.NakedObjectSpecification;
import org.nakedobjects.plugins.htmlviewer.component.Form;
import org.nakedobjects.runtime.context.NakedObjectsContext;
public class HtmlForm extends CompositeComponent implements Form {
private final boolean confirm;
private final boolean hasNext;
private final boolean hasPrevious;
private final boolean isEditing;
private final String id;
public HtmlForm(final String id, final String action, final int page, final int noOfPages, final boolean isEditing) {
this(id, action, page, noOfPages, false, isEditing);
}
private HtmlForm(
final String id,
final String action,
final int page,
final int noOfPages,
final boolean confirm,
final boolean isEditing) {
this.id = id;
this.confirm = confirm;
this.isEditing = isEditing;
hasPrevious = page >= 1;
hasNext = page < noOfPages - 1;
}
private void addField(
final String label,
final String field,
final String description,
final boolean readOnly,
final boolean required,
final String errorMessage) {
String error = "";
if (errorMessage != null) {
error = " " + errorMessage + "";
}
String optional = "";
if (!readOnly) {
if (!required) {
optional = " (optional)";
} else {
optional = " *";
}
}
add(new Html("" + label
+ ": " + field + optional + error + ""));
}
public void addField(
final NakedObjectSpecification spec,
final String label,
final String title,
final String field,
final String value,
final int noLines,
final boolean wrap,
final int maxLength,
final int typicalLength,
final boolean required,
final String error) {
String inputField;
/*
* REVIEW the following qualification are a bit limited - it's the simplest thing than will work - we
* need to determine from the specification whether something is boolean type or a password.
*
* Also see the note in the Form I/F.
*/
if (spec.isOfType(NakedObjectsContext.getSpecificationLoader().loadSpecification(boolean.class))
|| spec.isOfType(NakedObjectsContext.getSpecificationLoader().loadSpecification(Boolean.class))) {
final String selected = (value != null && value.toLowerCase().equals("true")) ? "checked " : "";
inputField = "";
} else if (spec.getFullName().endsWith(".Password")) {
final String typicalLengthStr = typicalLength == 0 ? "" : (" size=\"" + typicalLength + "\"");
final String maxLengthStr = maxLength == 0 ? "" : (" maxlength=\"" + maxLength + "\"");
inputField = "";
} else if (noLines > 1) {
final int w = typicalLength > 0 ? typicalLength / noLines : 50;
inputField = "";
} else {
final String typicalLengthStr = typicalLength == 0 ? "" : (" size=\"" + typicalLength + "\"");
final String maxLengthStr = maxLength == 0 ? "" : (" maxlength=\"" + maxLength + "\"");
inputField = "";
}
addField(label, inputField, title, false, required, error);
}
public void addFieldName(final String fieldLabel) {
add(new Heading(fieldLabel, 4));
}
public void addLookup(
final String fieldLabel,
final String description,
final String fieldId,
final int selectedIndex,
final String[] instances,
final String[] ids,
final boolean required,
final String errorMessage) {
final StringBuffer testInputField = new StringBuffer();
testInputField.append("");
addField(fieldLabel, testInputField.toString(), description, false, required, errorMessage);
}
public void addReadOnlyField(final String fieldLabel, final String title, final String description) {
addField(fieldLabel, "" + title + "", description, true, false, null);
}
@Override
public void write(final PrintWriter writer) {
super.write(writer);
}
@Override
protected void writeAfter(final PrintWriter writer) {
writer.println("");
if (hasNext) {
writer.println("");
if (isEditing) {
writer.println("");
} else {
writer.println("");
}
} else {
if (isEditing) {
writer.println("");
} else {
writer.println("");
}
}
if (hasPrevious) {
writer.println("");
}
writer.println("");
writer.println("");
writer.println("");
}
@Override
protected void writeBefore(final PrintWriter writer) {
writer.print("
© 2015 - 2025 Weber Informatics LLC | Privacy Policy