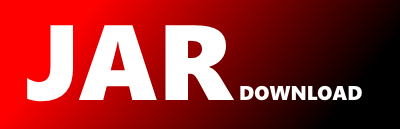
org.nakedobjects.plugins.htmlviewer.request.TableBuilder Maven / Gradle / Ivy
The newest version!
package org.nakedobjects.plugins.htmlviewer.request;
import java.util.Enumeration;
import org.nakedobjects.metamodel.adapter.NakedObject;
import org.nakedobjects.metamodel.facets.collections.modify.CollectionFacet;
import org.nakedobjects.metamodel.facets.propparam.multiline.MultiLineFacet;
import org.nakedobjects.metamodel.spec.NakedObjectSpecification;
import org.nakedobjects.metamodel.spec.feature.NakedObjectAssociation;
import org.nakedobjects.metamodel.spec.feature.NakedObjectAssociationFilters;
import org.nakedobjects.metamodel.spec.feature.OneToManyAssociation;
import org.nakedobjects.metamodel.util.CollectionFacetUtils;
import org.nakedobjects.plugins.htmlviewer.component.Table;
import org.nakedobjects.runtime.context.NakedObjectsContext;
public class TableBuilder {
public static Table createTable(
final Context context,
final String id,
final NakedObject object,
final OneToManyAssociation collectionField) {
final NakedObject collection = collectionField.get(object);
final String name = collectionField.getName();
final NakedObjectSpecification type = collectionField.getSpecification();
final String summary = "Table showing elements of " + name + " field";
return createTable(context, collectionField != null, collection, summary, type);
}
public static Table createTable(
final Context context,
final boolean addSelector,
final NakedObject collection,
final String summary,
final NakedObjectSpecification elementType) {
final CollectionFacet facet = CollectionFacetUtils.getCollectionFacetFromSpec(collection);
final Enumeration elements = facet.elements(collection);
final NakedObjectAssociation[] visibleFields = elementType
.getAssociations(NakedObjectAssociationFilters.STATICALLY_VISIBLE_ASSOCIATIONS);
final NakedObjectAssociation[] fields = new NakedObjectAssociation[visibleFields.length];
int len = 0;
for (int i = 0; i < visibleFields.length; i++) {
if (!visibleFields[i].isOneToManyAssociation()) {
fields[len++] = visibleFields[i];
}
}
final Table table = context.getComponentFactory().createTable(len, addSelector);
table.setSummary(summary);
for (int i = 0; i < len; i++) {
table.addColumnHeader(fields[i].getName());
}
while (elements.hasMoreElements()) {
final NakedObject element = (NakedObject) elements.nextElement();
NakedObjectsContext.getPersistenceSession().resolveImmediately(element);
final String elementId = context.mapObject(element);
table.addRowHeader(context.getComponentFactory().createObjectIcon(element, elementId, "icon"));
for (int i = 0; i < len; i++) {
final NakedObject fld = fields[i].get(element);
if (!fields[i].isVisible(NakedObjectsContext.getAuthenticationSession(), element).isAllowed()) {
table.addEmptyCell();
} else if (fld.getSpecification().isParseable()) {
final String titleString = fld != null ? fld.titleString() : null;
final MultiLineFacet multiline = fld.getSpecification().getFacet(MultiLineFacet.class);
final boolean shouldTruncate = multiline != null && multiline.numberOfLines() > 1;
table.addCell(titleString, shouldTruncate);
} else if (fields[i].isOneToOneAssociation() && fld == null) {
table.addEmptyCell();
} else if (fields[i].isOneToOneAssociation()) {
NakedObjectsContext.getPersistenceSession().resolveImmediately(fld);
final String objectId = context.mapObject(fld);
table.addCell(context.getComponentFactory().createObjectIcon(fields[i], fld, objectId, "icon"));
}
}
/*
* if (addSelector) { table.addCell(context.getFactory().createRemoveOption(id, elementId,
* collectionField.getId())); }
*/
// TODO add selection box
// table.addCell();
/*
* if (collectionField != null) { if (collectionField.isValidToRemove(object,
* element).isAllowed()) { table.addCell(context.getFactory().createRemoveOption(id, elementId,
* collectionField.getId())); } else { table.addEmptyCell(); } }
*/
}
return table;
}
}
// Copyright (c) Naked Objects Group Ltd.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy