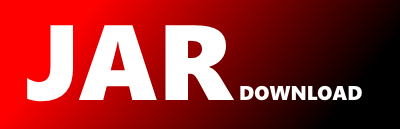
org.nakedobjects.plugins.remoting.client.facets.PropertySetterFacetWrapProxy Maven / Gradle / Ivy
package org.nakedobjects.plugins.remoting.client.facets;
import org.nakedobjects.metamodel.adapter.NakedObject;
import org.nakedobjects.metamodel.facets.DecoratingFacet;
import org.nakedobjects.metamodel.facets.properties.modify.PropertySetterFacet;
import org.nakedobjects.metamodel.facets.properties.modify.PropertySetterFacetAbstract;
import org.nakedobjects.plugins.remoting.shared.ObjectEncoder;
import org.nakedobjects.plugins.remoting.shared.ServerFacade;
import org.nakedobjects.plugins.remoting.shared.data.EncodeableObjectData;
import org.nakedobjects.plugins.remoting.shared.data.IdentityData;
import org.nakedobjects.plugins.remoting.shared.data.ObjectData;
import org.nakedobjects.runtime.context.NakedObjectsContext;
import org.nakedobjects.runtime.persistence.ConcurrencyException;
/**
* A reflection peer for changing one-to-many fields remotely, instead of on the local machine. Any requests
* to add or remove elements from the field will be passed over the network to the server for completion. Only
* requests on persistent objects are passed to the server; on a transient object the request will always be
* dealt with locally.
*
*
* If any of the objects involved have been changed on the server by another process then a
* ConcurrencyException will be passed back to the client and re-thrown.
*
*/
public final class PropertySetterFacetWrapProxy extends PropertySetterFacetAbstract implements
DecoratingFacet {
private final ServerFacade distribution;
private final ObjectEncoder encoder;
private final PropertySetterFacet underlyingFacet;
private final String name;
public PropertySetterFacetWrapProxy(
final PropertySetterFacet underlyingFacet,
final ServerFacade distribution,
final ObjectEncoder encoder,
final String name) {
super(underlyingFacet.getFacetHolder());
this.underlyingFacet = underlyingFacet;
this.distribution = distribution;
this.encoder = encoder;
this.name = name;
}
public PropertySetterFacet getDecoratedFacet() {
return underlyingFacet;
}
public void setProperty(final NakedObject inObject, final NakedObject value) {
if (ProxyUtil.executeRemotely(inObject)) {
final IdentityData targetReference = encoder.createIdentityData(inObject);
ObjectData[] updates;
try {
if (value.getSpecification().isObject()) {
final IdentityData associateReference = encoder.createIdentityData(value);
updates = distribution.setAssociation(NakedObjectsContext.getAuthenticationSession(), name, targetReference,
associateReference);
} else {
final EncodeableObjectData val = value == null ? null : encoder.createValue(value);
updates = distribution.setValue(NakedObjectsContext.getAuthenticationSession(), name, targetReference, val);
}
} catch (final ConcurrencyException e) {
throw ProxyUtil.concurrencyException(e);
}
ProxyUtil.updateChangedObjects(updates, encoder);
} else {
underlyingFacet.setProperty(inObject, value);
}
}
}
// Copyright (c) Naked Objects Group Ltd.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy