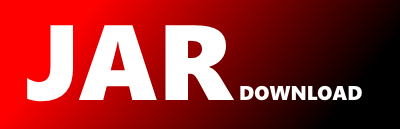
org.nakedobjects.plugins.remoting.shared.data.CollectionDataImpl Maven / Gradle / Ivy
package org.nakedobjects.plugins.remoting.shared.data;
import java.io.Serializable;
import org.nakedobjects.metamodel.adapter.oid.Oid;
import org.nakedobjects.metamodel.adapter.version.Version;
import org.nakedobjects.metamodel.commons.encoding.ByteDecoder;
import org.nakedobjects.metamodel.commons.encoding.ByteEncoder;
import org.nakedobjects.metamodel.commons.encoding.Encodable;
import org.nakedobjects.metamodel.commons.lang.ToString;
public class CollectionDataImpl implements CollectionData, Encodable, Serializable {
private static final long serialVersionUID = 1L;
private final ReferenceData elements[];
private final Oid oid;
private final String collectionType;
private final Version version;
private final boolean hasAllElements;
private final String elementType;
public CollectionDataImpl(
final Oid oid,
final String collectionType,
final String elementType,
final ReferenceData[] elements,
final boolean hasAllElements,
final Version version) {
this.oid = oid;
this.collectionType = collectionType;
this.elementType = elementType;
this.elements = elements;
this.hasAllElements = hasAllElements;
this.version = version;
}
public CollectionDataImpl(final ByteDecoder decoder) {
collectionType = decoder.getString();
elementType = decoder.getString();
oid = (Oid) decoder.getObject();
version = (Version) decoder.getObject();
hasAllElements = decoder.getBoolean();
elements = (ReferenceData[]) decoder.getObjects();
}
public void encode(final ByteEncoder encoder) {
encoder.add(collectionType);
encoder.add(elementType);
encoder.add(oid);
encoder.add(version);
encoder.add(hasAllElements);
encoder.add(elements);
}
public ReferenceData[] getElements() {
return elements;
}
public String getElementype() {
return elementType;
}
public Oid getOid() {
return oid;
}
public String getType() {
return collectionType;
}
public Version getVersion() {
return version;
}
public boolean hasAllElements() {
return hasAllElements;
}
@Override
public String toString() {
final ToString str = new ToString(this);
str.append("collection type", collectionType);
str.append("element type", elementType);
str.append("oid", oid);
str.append("version", version);
str.append(",elements=");
for (int i = 0; elements != null && i < elements.length; i++) {
if (i > 0) {
str.append(";");
}
if (elements[i] == null) {
str.append("null");
} else {
final String name = elements[i].getClass().getName();
str.append(name.substring(name.lastIndexOf('.') + 1));
}
}
return str.toString();
}
}
// Copyright (c) Naked Objects Group Ltd.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy