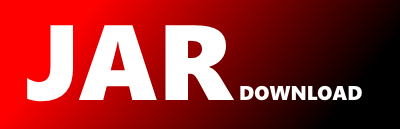
org.nakedobjects.example.expenses.claims.Claims Maven / Gradle / Ivy
The newest version!
package org.nakedobjects.example.expenses.claims;
import java.util.List;
import org.nakedobjects.applib.AbstractService;
import org.nakedobjects.applib.ApplicationException;
import org.nakedobjects.applib.annotation.MemberOrder;
import org.nakedobjects.applib.annotation.Named;
import org.nakedobjects.applib.annotation.Optional;
import org.nakedobjects.example.expenses.employee.Employee;
import org.nakedobjects.example.expenses.services.UserFinder;
/**
* Claims acts as a facade onto the ClaimRepository and ClaimFactory. It wraps the methods that are intended to be
* invoked directly by a user, adding:
* - mark-up (e.g. naming the parameters)
* - helper logic e.g. default.. or choices.. methods
* - user-friendly wrapping for error conditions.
*/
public class Claims extends AbstractService {
// {{ Title & ID
public String getId() {
return getClass().getSimpleName();
}
public String iconName() {
return Claim.class.getSimpleName();
}
// }}
// {{ Injected Services
/*
* This region contains references to the services (Repositories,
* Factories or other Services) used by this domain object. The
* references are injected by the application container.
*/
// {{ Injected: UserFinder
private UserFinder userFinder;
/**
* This field is not persisted, nor displayed to the user.
*/
protected UserFinder getUserFinder() {
return this.userFinder;
}
/**
* Injected by the application container.
*/
public void setUserFinder(UserFinder userFinder) {
this.userFinder = userFinder;
}
// }}
// {{ Injected: ClaimRepository
private ClaimRepository claimRepository;
/**
* This field is not persisted, nor displayed to the user.
*/
protected ClaimRepository getClaimRepository() {
return this.claimRepository;
}
/**
* Injected by the application container.
*/
public void setClaimRepository(ClaimRepository claimRepository) {
this.claimRepository = claimRepository;
}
// }}
// {{ Injected: ClaimFactory
private ClaimFactory claimFactory;
/**
* This field is not persisted, nor displayed to the user.
*/
protected ClaimFactory getClaimFactory() {
return this.claimFactory;
}
/**
* Injected by the application container.
*/
public void setClaimFactory(ClaimFactory claimFactory) {
this.claimFactory = claimFactory;
}
// }}
// }}
@MemberOrder(sequence = "2")
public List findMyClaims(@Named("Status")
@Optional final ClaimStatus status,
@Optional
@Named("Description") final String description) {
return claimRepository.findClaims(meAsEmployee(), status, description);
}
@MemberOrder(sequence = "1")
public List myRecentClaims() {
return claimRepository.findRecentClaims(meAsEmployee());
}
private Employee meAsEmployee() {
Object user = userFinder.currentUserAsObject();
if (user instanceof Employee) {
return (Employee) user;
}
throw new ApplicationException("Current user is not an Employee");
}
@MemberOrder(sequence="3")
public Claim createNewClaim(@Named("Description") String description) {
return claimFactory.createNewClaim(meAsEmployee(), description);
}
public Object[] defaultCreateNewClaim() {
return new Object[] {claimFactory.defaultUniqueClaimDescription(meAsEmployee())};
}
@MemberOrder(sequence="6")
public List claimsAwaitingMyApproval() {
return claimRepository.findClaimsAwaitingApprovalBy(meAsEmployee());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy