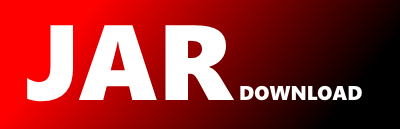
org.nakedobjects.nos.remote.command.socket.ClientConnection Maven / Gradle / Ivy
package org.nakedobjects.nos.remote.command.socket;
import org.nakedobjects.noa.persist.ConcurrencyException;
import org.nakedobjects.nof.core.conf.Configuration;
import org.nakedobjects.nof.core.context.NakedObjectsContext;
import org.nakedobjects.nof.reflect.remote.NakedObjectsRemoteException;
import org.nakedobjects.nos.remote.command.CommandClientConnection;
import org.nakedobjects.nos.remote.command.ConnectionException;
import org.nakedobjects.nos.remote.command.Request;
import org.nakedobjects.nos.remote.command.Response;
import java.io.IOException;
import java.io.InputStream;
import java.io.OutputStream;
import java.net.ConnectException;
import java.net.MalformedURLException;
import java.net.Socket;
import java.net.UnknownHostException;
import org.apache.log4j.Logger;
public abstract class ClientConnection implements CommandClientConnection {
private static final Logger LOG = Logger.getLogger(ClientConnection.class);
private Socket socket;
private int port;
private String host;
private ProfilingOutputStream profilingOutputStream;
private ProfilingInputStream profilingInputStream;
private boolean keepAlive;
private void connect() {
if (socket == null) {
try {
socket = new Socket(host, port);
InputStream input = socket.getInputStream();
OutputStream output = socket.getOutputStream();
if (debugging()) {
output = profilingOutputStream = new ProfilingOutputStream(output);
input = profilingInputStream = new ProfilingInputStream(input);
}
openStreams(input, output);
LOG.debug("connection established " + socket);
} catch (MalformedURLException e) {
throw new ConnectionException("Connection failure", e);
} catch (UnknownHostException e) {
throw new ConnectionException("Connection failure", e);
} catch (ConnectException e) {
throw new ConnectionException("Failed to connect to " + host + "/" + port, e);
} catch (IOException e) {
throw new ConnectionException("Connection failure", e);
}
}
}
private boolean debugging() {
return true;
}
private void disconnect() {
if (socket != null) {
try {
socket.close();
socket = null;
} catch (IOException e) {
LOG.error("failed to close connection", e);
}
}
}
public Response executeRemotely(final Request request) {
connect();
Object response;
try {
response = request(request);
if (profilingOutputStream != null) {
LOG.debug(profilingOutputStream.getSize() + " bytes sent in "+ profilingOutputStream.getTime() + " seconds");
profilingOutputStream.resetTimer();
}
if (profilingInputStream != null) {
LOG.debug(profilingInputStream.getSize() + " bytes received in " + profilingInputStream.getTime() + " seconds");
profilingInputStream.resetTimer();
}
if (!keepAlive) {
disconnect();
}
} catch (IOException e) {
disconnect();
throw new ConnectionException("Failed request", e);
}
if (response instanceof ConcurrencyException) {
throw (ConcurrencyException) response;
} else if (response instanceof Exception) {
throw new NakedObjectsRemoteException("Exception occurred on server", (Throwable) response);
} else {
return (Response) response;
}
}
public void init() {
port = ConnectionProperties.port();
host = ConnectionProperties.host();
keepAlive = NakedObjectsContext.getConfiguration().getBoolean(Configuration.ROOT + "remote.keepalive", false);
LOG.info("connections will be made to " + host + " " + port);
}
protected abstract void openStreams(InputStream input, OutputStream output) throws IOException;
protected void reconnect() {
disconnect();
connect();
}
protected abstract Object request(final Request request) throws IOException;
public void shutdown() {
disconnect();
}
}
// Copyright (c) Naked Objects Group Ltd.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy