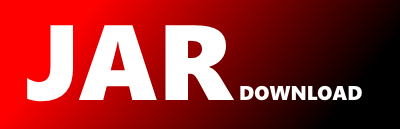
org.nakedobjects.nos.remote.command.socket.ProfilingInputStream Maven / Gradle / Ivy
package org.nakedobjects.nos.remote.command.socket;
import java.io.IOException;
import java.io.InputStream;
/**
* Collection of timing and quantity data for the stream. Note that as the reads block the clock does not start
* until the first read has completed.
*/
public class ProfilingInputStream extends InputStream {
private final InputStream wrapped;
private int bytes = 0;
private long end = 0;
private long start = 0;
public ProfilingInputStream(final InputStream wrapped) {
this.wrapped = wrapped;
}
private void end() {
if (start == 0) {
start = System.currentTimeMillis();
}
end = System.currentTimeMillis();
}
public int getSize() {
return bytes;
}
public float getTime() {
return (end - start) / 1000.0f;
}
public int read() throws IOException {
start();
int read = wrapped.read();
bytes++;
end();
return read;
}
public int read(byte[] b) throws IOException {
start();
int read = wrapped.read(b);
bytes += read;
end();
return read;
}
public int read(byte[] b, int off, int len) throws IOException {
start();
int read = wrapped.read(b, off, len);
bytes += read;
end();
return read;
}
public void resetTimer() {
bytes = 0;
start = end = 0;
}
private void start() {
}
}
// Copyright (c) Naked Objects Group Ltd.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy