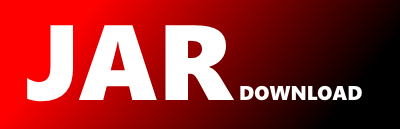
org.nakedobjects.nos.client.dnd.UserActionSet Maven / Gradle / Ivy
The newest version!
package org.nakedobjects.nos.client.dnd;
import java.util.Vector;
import org.nakedobjects.noa.reflect.Consent;
import org.nakedobjects.noa.reflect.NakedObjectAction.Type;
import org.nakedobjects.nof.core.reflect.Allow;
import org.nakedobjects.nof.core.util.ToString;
import org.nakedobjects.nos.client.dnd.drawing.Color;
import org.nakedobjects.nos.client.dnd.drawing.Location;
public class UserActionSet implements UserAction {
private Color backgroundColor = Toolkit.getColor("debug.baseline");
private final String groupName;
private final boolean includeDebug;
private final boolean includeExploration;
private Vector options = new Vector();
private final Type type;
public UserActionSet(final boolean includeExploration, final boolean includeDebug, final Type type) {
this.type = type;
this.groupName = "";
this.includeExploration = includeExploration;
this.includeDebug = includeDebug;
}
public UserActionSet(final String groupName, final UserActionSet parent) {
this.groupName = groupName;
this.includeExploration = parent.includeExploration;
this.includeDebug = parent.includeDebug;
this.type = parent.type;
this.backgroundColor = parent.getColor();
}
public UserActionSet(final String groupName, final UserActionSet parent, final Type type) {
this.groupName = groupName;
this.includeExploration = parent.includeExploration;
this.includeDebug = parent.includeDebug;
this.type = type;
this.backgroundColor = parent.getColor();
}
/**
* Add the specified option if it is of the right type for this menu.
*/
public void add(final UserAction option) {
Type section = option.getType();
if (section == USER || (includeExploration && section == EXPLORATION) || (includeDebug && section == DEBUG)) {
options.addElement(option);
}
}
public Consent disabled(final View view) {
return Allow.DEFAULT;
}
public void execute(final Workspace workspace, final View view, final Location at) {}
/**
* Returns the background colour for the menu
*/
public Color getColor() {
return backgroundColor;
}
public String getDescription(final View view) {
return "";
}
public String getHelp(final View view) {
return "";
}
public UserAction[] getMenuOptions() {
UserAction[] v = new UserAction[options.size()];
for (int i = 0; i < v.length; i++) {
v[i] = (UserAction) options.elementAt(i);
}
return v;
}
public String getName(final View view) {
return groupName;
}
public Type getType() {
return type;
}
/**
* Specifies the background colour for the menu
*/
public void setColor(final Color color) {
backgroundColor = color;
}
public String toString() {
ToString str = new ToString(this);
str.append("type", type);
for (int i = 0, size = options.size(); i < size; i++) {
str.append(((UserAction) options.elementAt(i)).getClass() + " ,");
}
return str.toString();
}
}
// Copyright (c) Naked Objects Group Ltd.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy