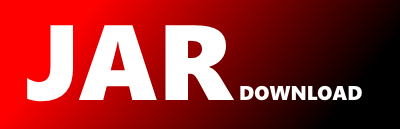
org.nakedobjects.nos.client.dnd.basic.EmptyField Maven / Gradle / Ivy
package org.nakedobjects.nos.client.dnd.basic;
import org.nakedobjects.noa.adapter.Naked;
import org.nakedobjects.noa.adapter.NakedObject;
import org.nakedobjects.noa.reflect.Consent;
import org.nakedobjects.nos.client.dnd.Canvas;
import org.nakedobjects.nos.client.dnd.Content;
import org.nakedobjects.nos.client.dnd.ContentDrag;
import org.nakedobjects.nos.client.dnd.ObjectContent;
import org.nakedobjects.nos.client.dnd.ObjectParameter;
import org.nakedobjects.nos.client.dnd.OneToOneField;
import org.nakedobjects.nos.client.dnd.Toolkit;
import org.nakedobjects.nos.client.dnd.View;
import org.nakedobjects.nos.client.dnd.ViewAxis;
import org.nakedobjects.nos.client.dnd.ViewSpecification;
import org.nakedobjects.nos.client.dnd.border.ObjectBorder;
import org.nakedobjects.nos.client.dnd.drawing.Location;
import org.nakedobjects.nos.client.dnd.drawing.Size;
import org.nakedobjects.nos.client.dnd.drawing.Text;
import org.nakedobjects.nos.client.dnd.lookup.OpenObjectDropDownBorder;
import org.nakedobjects.nos.client.dnd.view.graphic.IconGraphic;
import org.nakedobjects.nos.client.dnd.view.simple.AbstractView;
import org.nakedobjects.nos.client.dnd.view.text.TitleText;
public class EmptyField extends AbstractView {
public static class Specification implements ViewSpecification {
public boolean canDisplay(final Content content) {
return content == null || content.getNaked() == null;
}
public View createView(final Content content, final ViewAxis axis) {
EmptyField emptyField = new EmptyField(content, this, axis, Toolkit.getText("normal"));
if ((content instanceof OneToOneField && ((OneToOneField) content).isEditable().isAllowed()) || content instanceof ObjectParameter) {
if (content.isOptionEnabled()) {
return new ObjectBorder(new OpenObjectDropDownBorder(emptyField));
} else {
return new ObjectBorder(emptyField);
}
} else {
return emptyField;
}
}
public String getName() {
return "empty field";
}
public boolean isAligned() {
return false;
}
public boolean isOpen() {
return false;
}
public boolean isReplaceable() {
return true;
}
public boolean isSubView() {
return true;
}
}
private IconGraphic icon;
private TitleText text;
public EmptyField(final Content content, final ViewSpecification specification, final ViewAxis axis, final Text style) {
super(content, specification, axis);
if (((ObjectContent) content).getObject() != null) {
throw new IllegalArgumentException("Content for EmptyField must be null: " + content);
}
NakedObject object = ((ObjectContent) getContent()).getObject();
if (object != null) {
throw new IllegalArgumentException("Content for EmptyField must be null: " + object);
}
icon = new IconGraphic(this, style);
text = new EmptyFieldTitleText(this, style);
}
public void draw(final Canvas canvas) {
super.draw(canvas);
int x = 0;
int y = icon.getBaseline();
icon.draw(canvas, x, y);
x += icon.getSize().getWidth();
x += View.HPADDING;
text.draw(canvas, x, y);
}
public int getBaseline() {
return icon.getBaseline();
}
public Size getMaximumSize() {
Size size = icon.getSize();
size.extendWidth(View.HPADDING);
size.extendWidth(text.getSize().getWidth());
return size;
}
private Consent canDrop(final NakedObject dragSource) {
ObjectContent content = (ObjectContent) getContent();
return content.canSet(dragSource);
}
public void dragIn(final ContentDrag drag) {
Content sourceContent = drag.getSourceContent();
if (sourceContent instanceof ObjectContent) {
NakedObject source = ((ObjectContent) sourceContent).getObject();
Consent canDrop = canDrop(source);
if (canDrop.isAllowed()) {
getState().setCanDrop();
} else {
getState().setCantDrop();
}
if (!canDrop.getReason().equals("")) {
getFeedbackManager().setAction(canDrop.getReason());
} else {
getFeedbackManager().setAction("Set to " + sourceContent.title());
}
} else {
getState().setCantDrop();
}
markDamaged();
}
public void dragOut(final ContentDrag drag) {
getState().clearObjectIdentified();
markDamaged();
}
public void drop(final ContentDrag drag) {
getState().clearViewIdentified();
markDamaged();
NakedObject target = ((ObjectContent) getParent().getContent()).getObject();
Content sourceContent = drag.getSourceContent();
if (sourceContent instanceof ObjectContent) {
NakedObject source = ((ObjectContent) sourceContent).getObject();
setField(target, source);
}
}
/**
* Objects returned by menus are used to set this field before passing the call on to the parent.
*/
public void objectActionResult(final Naked result, final Location at) {
NakedObject target = ((ObjectContent) getParent().getContent()).getObject();
if (result instanceof NakedObject) {
setField(target, (NakedObject) result);
}
super.objectActionResult(result, at);
}
private void setField(final NakedObject parent, final NakedObject object) {
if (canDrop(object).isAllowed()) {
((ObjectContent) getContent()).setObject(object);
getParent().invalidateContent();
}
}
public String toString() {
return "EmptyField" + getId();
}
}
// Copyright (c) Naked Objects Group Ltd.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy