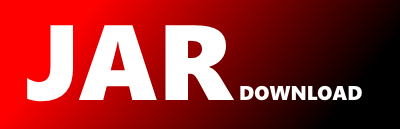
org.nakedobjects.nos.client.dnd.content.ObjectParameterImpl Maven / Gradle / Ivy
The newest version!
package org.nakedobjects.nos.client.dnd.content;
import org.nakedobjects.noa.adapter.Naked;
import org.nakedobjects.noa.adapter.NakedObject;
import org.nakedobjects.noa.reflect.Consent;
import org.nakedobjects.noa.spec.NakedObjectSpecification;
import org.nakedobjects.nof.core.reflect.Allow;
import org.nakedobjects.nof.core.reflect.Veto;
import org.nakedobjects.nof.core.util.DebugString;
import org.nakedobjects.nof.core.util.ToString;
import org.nakedobjects.nos.client.dnd.ObjectParameter;
import org.nakedobjects.nos.client.dnd.UserActionSet;
import org.nakedobjects.nos.client.dnd.View;
import org.nakedobjects.nos.client.dnd.Workspace;
import org.nakedobjects.nos.client.dnd.action.AbstractUserAction;
import org.nakedobjects.nos.client.dnd.drawing.Location;
public class ObjectParameterImpl extends AbstractObjectContent implements ObjectParameter {
private final NakedObject object;
private final String name;
private final NakedObjectSpecification specification;
private final ActionHelper invocation;
private final int i;
private final boolean isRequired;
private final NakedObject[] options;
private final String description;
public ObjectParameterImpl(
final String name,
final String description,
final Naked naked,
final NakedObjectSpecification specification,
final boolean required,
final NakedObject[] options,
final int i,
final ActionHelper invocation) {
this.name = name;
this.description = description;
this.specification = specification;
this.isRequired = required;
this.options = options;
this.i = i;
this.invocation = invocation;
object = (NakedObject) naked;
}
public ObjectParameterImpl(final ObjectParameterImpl content, final NakedObject object) {
name = content.name;
description = content.description;
specification = content.specification;
isRequired = content.isRequired;
options = content.options;
i = content.i;
invocation = content.invocation;
this.object = object;
}
public Consent canClear() {
return Allow.DEFAULT;
}
public Consent canSet(final NakedObject dragSource) {
if (dragSource.getSpecification().isOfType(specification)) {
return Allow.DEFAULT;
} else {
return new Veto("Object must be " + specification.getShortName());
}
}
public void clear() {
setObject(null);
}
public void debugDetails(final DebugString debug) {
debug.appendln("name", name);
debug.appendln("required", isRequired);
debug.appendln("object", object);
}
public Naked getNaked() {
return object;
}
public NakedObject getObject() {
return object;
}
public Naked[] getOptions() {
return options;
}
public boolean isObject() {
return true;
}
public boolean isRequired() {
return isRequired;
}
public boolean isPersistable() {
return false;
}
public boolean isOptionEnabled() {
return options != null;
}
public boolean isTransient() {
return object != null && object.getResolveState().isTransient();
}
public void contentMenuOptions(final UserActionSet options) {
if (object != null) {
options.add(new AbstractUserAction("Clear parameter") {
public void execute(final Workspace workspace, final View view, final Location at) {
clear();
view.getParent().invalidateContent();
}
});
OptionFactory.addObjectMenuOptions(object, options);
} else {
OptionFactory.addCreateOptions(specification, options);
}
}
public void setObject(final NakedObject object) {
invocation.setParameter(i, object);
}
public String title() {
return object == null ? "" : object.titleString();
}
public String toString() {
ToString toString = new ToString(this);
toString.append("label", name);
toString.append("required", isRequired);
toString.append("spec", getSpecification().getFullName());
toString.append("object", object == null ? "null" : object.titleString());
return toString.toString();
}
public String getParameterName() {
return name;
}
public NakedObjectSpecification getSpecification() {
return specification;
}
public String getDescription() {
String title = object == null ? "" : ": " + object.titleString();
String type = name.indexOf(specification.getShortName()) == -1 ? " (" + specification.getShortName() + ")" : "";
return name + type + title + " " + description;
}
public String getHelp() {
return invocation.getHelp();
}
public String getId() {
return null;
}
}
// Copyright (c) Naked Objects Group Ltd.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy