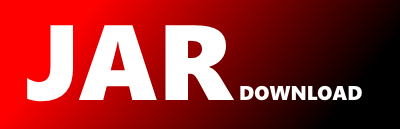
org.nakedobjects.nos.client.dnd.content.OneToManyFieldImpl Maven / Gradle / Ivy
The newest version!
package org.nakedobjects.nos.client.dnd.content;
import org.nakedobjects.noa.NakedObjectRuntimeException;
import org.nakedobjects.noa.adapter.Naked;
import org.nakedobjects.noa.adapter.NakedCollection;
import org.nakedobjects.noa.adapter.NakedObject;
import org.nakedobjects.noa.reflect.Consent;
import org.nakedobjects.noa.reflect.NakedObjectField;
import org.nakedobjects.noa.reflect.OneToManyAssociation;
import org.nakedobjects.noa.spec.Features;
import org.nakedobjects.noa.spec.NakedObjectSpecification;
import org.nakedobjects.nof.core.reflect.Veto;
import org.nakedobjects.nof.core.util.DebugString;
import org.nakedobjects.nos.client.dnd.Content;
import org.nakedobjects.nos.client.dnd.OneToManyField;
import org.nakedobjects.nos.client.dnd.UserActionSet;
import org.nakedobjects.nos.client.dnd.drawing.Image;
import org.nakedobjects.nos.client.dnd.image.ImageFactory;
public class OneToManyFieldImpl extends AbstractCollectionContent implements OneToManyField {
private final NakedCollection collection;
private final ObjectField field;
public OneToManyFieldImpl(final NakedObject parent, final NakedCollection object, final OneToManyAssociation association) {
field = new ObjectField(parent, association);
this.collection = (NakedCollection) object;
}
public Consent canClear() {
return Veto.DEFAULT;
}
public Consent canDrop(final Content sourceContent) {
if (sourceContent.getNaked() instanceof NakedObject) {
NakedObject object = (NakedObject) sourceContent.getNaked();
NakedObject parent = field.getParent();
NakedCollection collection = (NakedCollection) getNaked();
if (collection == null) {
return new Veto("Collection not set up; can't add elements to a non-existant collection");
}
Consent usableByUser = getOneToManyAssociation().isUsable();
if (usableByUser.isVetoed()) {
return usableByUser;
}
Consent usableInState = getOneToManyAssociation().isUsable(parent);
if (usableInState.isVetoed()) {
return usableInState;
}
NakedObjectSpecification specification = object.getSpecification();
if (!specification.isOfType(collection.getElementSpecification())) {
return new Veto("Only objects of type " + collection.getElementSpecification().getSingularName()
+ " are allowed in this collection");
}
if (parent.getResolveState().isPersistent() && object.getResolveState().isTransient()) {
return new Veto("Can't set field in persistent object with reference to non-persistent object");
}
if (Features.isAggregated(specification) && specification.getAggregate(object) != getParent()) {
return new Veto("Object is already associated with another object: " + specification.getAggregate(object));
}
return getOneToManyAssociation().isValidToAdd(parent, object);
} else {
return Veto.DEFAULT;
}
}
public Consent canSet(final NakedObject dragSource) {
return Veto.DEFAULT;
}
public void clear() {
throw new NakedObjectRuntimeException("Invalid call");
}
public void contentMenuOptions(final UserActionSet options) {
super.contentMenuOptions(options);
OptionFactory.addCreateOptions(getOneToManyAssociation().getSpecification(), options);
}
public void debugDetails(final DebugString debug) {
field.debugDetails(debug);
debug.appendln("collection", collection);
super.debugDetails(debug);
}
public Naked drop(final Content sourceContent) {
NakedObject object = (NakedObject) sourceContent.getNaked();
NakedObject parent = field.getParent();
Consent perm = canDrop(sourceContent);
if (perm.isAllowed()) {
getOneToManyAssociation().addElement(parent, object);
}
return null;
}
public NakedCollection getCollection() {
return collection;
}
public String getDescription() {
String name = getFieldName();
String type = getField().getSpecification().getSingularName();
type = name.indexOf(type) == -1 ? " (" + type + ")" : "";
String description = getOneToManyAssociation().getDescription();
return name + type + " " + description;
}
public NakedObjectField getField() {
return field.getFieldReflector();
}
public String getFieldName() {
return field.getName();
}
public String getHelp() {
return getOneToManyAssociation().getHelp();
}
public String getIconName() {
return null;
// return "internal-collection";
}
public Image getIconPicture(final int iconHeight) {
NakedObjectSpecification specification = getOneToManyAssociation().getSpecification();
Image icon = ImageFactory.getInstance().loadIcon(specification, iconHeight, null);
if (icon == null) {
icon = ImageFactory.getInstance().loadDefaultIcon(iconHeight, null);
}
return icon;
}
public String getId() {
return getOneToManyAssociation().getId();
}
public Naked getNaked() {
return collection;
}
public OneToManyAssociation getOneToManyAssociation() {
return (OneToManyAssociation) field.getFieldReflector();
}
public NakedObject getParent() {
return field.getParent();
}
public NakedObjectSpecification getSpecification() {
return field.getSpecification();
}
public boolean isCollection() {
return true;
}
public boolean isMandatory() {
return getOneToManyAssociation().isMandatory();
}
public boolean isTransient() {
return false;
}
public void setObject(final NakedObject object) {
throw new NakedObjectRuntimeException("Invalid call");
}
public final String title() {
return field.getName();
}
public String toString() {
return collection + "/" + field.getFieldReflector();
}
public String windowTitle() {
return title() + " for " + field.getParent().titleString();
}
}
// Copyright (c) Naked Objects Group Ltd.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy