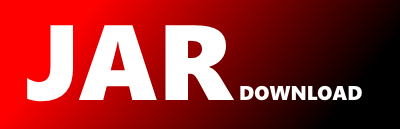
org.nakedobjects.nos.client.dnd.viewer.ViewerFrame Maven / Gradle / Ivy
package org.nakedobjects.nos.client.dnd.viewer;
import java.awt.FileDialog;
import java.awt.Frame;
import java.awt.Graphics;
import java.awt.Image;
import java.awt.event.ComponentAdapter;
import java.awt.event.ComponentEvent;
import java.awt.event.WindowAdapter;
import java.awt.event.WindowEvent;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.net.URL;
import org.nakedobjects.nof.core.util.AboutNakedObjects;
import org.nakedobjects.nos.client.dnd.Toolkit;
public class ViewerFrame extends Frame implements RenderingArea {
private static final String DEFAULT_TITLE = "Naked Objects";
private static final long serialVersionUID = 1L;
private XViewer viewer;
public ViewerFrame() {}
{
setBackground(((AwtColor) Toolkit.getColor("background.application")).getAwtColor());
AWTUtilities.addWindowIcon(this, "application-logo.png");
setTitle(null);
/*
* compensate for change in tab handling in Java 1.4
*/
try {
Class c = getClass();
Method m = c.getMethod("setFocusTraversalKeysEnabled", new Class[] { Boolean.TYPE });
m.invoke(this, new Object[] { Boolean.FALSE });
} catch (SecurityException e1) {
e1.printStackTrace();
} catch (NoSuchMethodException ignore) {
/*
* Ignore no such method exception as this method is only available, but needed, in version 1.4
* and later.
*/
} catch (IllegalArgumentException e) {
e.printStackTrace();
} catch (IllegalAccessException e) {
e.printStackTrace();
} catch (InvocationTargetException e) {
e.printStackTrace();
}
}
/**
* Calls update()
to do double-buffered drawing of all views.
*
* @see #update(Graphics)
* @see java.awt.Component#paint(Graphics)
*/
public final void paint(Graphics g) {
update(g);
}
public void quit() {
viewer.quit();
}
/**
* Paints the double-buffered image. Calls the draw()
method on each top-level view.
*
* @see java.awt.Component#update(Graphics)
*/
public void update(final Graphics g) {
viewer.paint(g);
}
public void setViewer(final XViewer viewer) {
this.viewer = viewer;
}
public void init() {
addWindowListener(new WindowAdapter() {
public void windowClosing(final WindowEvent e) {
new ShutdownDialog(ViewerFrame.this);
}
});
addComponentListener(new ComponentAdapter() {
public void componentResized(final ComponentEvent e) {
ViewerFrame.this.viewer.sizeChange();
}
});
}
/**
* Expose as a .NET property
*
* @property
*/
public void set_Viewer(final XViewer viewer) {
setViewer(viewer);
}
/**
* Expose as a .NET property
*
* @property
*/
public void set_Title(final String title) {
setTitle(title);
}
public void setTitle(final String title) {
String application = AboutNakedObjects.getApplicationName();
String str = title == null ? (application == null ? DEFAULT_TITLE : application) : title;
super.setTitle(str);
}
public String selectFilePath(final String title, final String directory) {
FileDialog dlg = new FileDialog(this, title);
dlg.show();
String path = dlg.getDirectory() + dlg.getFile();
return path;
}
}
// Copyright (c) Naked Objects Group Ltd.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy