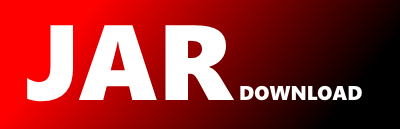
net.sf.nakeduml.userinteractionmetamodel.AbstractUserInteractionFolder Maven / Gradle / Ivy
package net.sf.nakeduml.userinteractionmetamodel;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import net.sf.nakeduml.util.CompositionNode;
abstract public class AbstractUserInteractionFolder extends UserInteractionElement implements CompositionNode {
private Set operationUserInteraction = new HashSet();
private Set entityUserInteraction = new HashSet();
private Set childFolder = new HashSet();
/** Default constructor for
*/
public AbstractUserInteractionFolder() {
}
public void addAllToChildFolder(Set childFolder) {
for ( UserInteractionFolder o : childFolder ) {
addToChildFolder(o);
}
}
public void addAllToEntityUserInteraction(Set entityUserInteraction) {
for ( ClassifierUserInteraction o : entityUserInteraction ) {
addToEntityUserInteraction(o);
}
}
public void addAllToOperationUserInteraction(Set operationUserInteraction) {
for ( OperationUserInteraction o : operationUserInteraction ) {
addToOperationUserInteraction(o);
}
}
public void addToChildFolder(UserInteractionFolder childFolder) {
childFolder.setParentFolder(this);
}
public void addToEntityUserInteraction(ClassifierUserInteraction entityUserInteraction) {
entityUserInteraction.setFolder(this);
}
public void addToOperationUserInteraction(OperationUserInteraction operationUserInteraction) {
operationUserInteraction.setFolder(this);
}
/** Call this method when you want to attach this object to the containment tree. Useful with transitive persistence
*/
public void addToOwningObject() {
}
public void clearChildFolder() {
removeAllFromChildFolder(getChildFolder());
}
public void clearEntityUserInteraction() {
removeAllFromEntityUserInteraction(getEntityUserInteraction());
}
public void clearOperationUserInteraction() {
removeAllFromOperationUserInteraction(getOperationUserInteraction());
}
public void copyState(AbstractUserInteractionFolder from, AbstractUserInteractionFolder to) {
to.setName(from.getName());
to.setAdditionalHumanName(from.getAdditionalHumanName());
to.setRepresentedElement(getRepresentedElement());
for ( OperationUserInteraction child : getOperationUserInteraction() ) {
to.addToOperationUserInteraction(child.makeCopy());
}
for ( ClassifierUserInteraction child : getEntityUserInteraction() ) {
to.addToEntityUserInteraction(child.makeCopy());
}
for ( UserInteractionFolder child : getChildFolder() ) {
to.addToChildFolder(child.makeCopy());
}
}
public UserInteractionFolder createChildFolder() {
UserInteractionFolder newInstance= new UserInteractionFolder();
newInstance.init(this);
return newInstance;
}
public void createComponents() {
super.createComponents();
}
public ClassifierUserInteraction createEntityUserInteraction() {
ClassifierUserInteraction newInstance= new ClassifierUserInteraction();
newInstance.init(this);
return newInstance;
}
public OperationUserInteraction createOperationUserInteraction() {
OperationUserInteraction newInstance= new OperationUserInteraction();
newInstance.init(this);
return newInstance;
}
public Set getChildFolder() {
return childFolder;
}
public Set getEntityUserInteraction() {
return entityUserInteraction;
}
public Set getOperationUserInteraction() {
return operationUserInteraction;
}
public Set getOwnedElement() {
Set ownedElementSubsetting = new HashSet();
ownedElementSubsetting.addAll(super.getOwnedElement());
ownedElementSubsetting.addAll(getOperationUserInteraction());
ownedElementSubsetting.addAll(getEntityUserInteraction());
ownedElementSubsetting.addAll(getChildFolder());
return ownedElementSubsetting;
}
public CompositionNode getOwningObject() {
return null;
}
public List getPathFromRoot() {
List pathFromRoot = (List)collectionLiteral1();
return pathFromRoot;
}
public void init(CompositionNode owner) {
super.init(owner);
createComponents();
}
abstract public AbstractUserInteractionFolder makeCopy();
public void markDeleted() {
super.markDeleted();
for ( OperationUserInteraction child : new ArrayList(getOperationUserInteraction()) ) {
child.markDeleted();
}
for ( ClassifierUserInteraction child : new ArrayList(getEntityUserInteraction()) ) {
child.markDeleted();
}
for ( UserInteractionFolder child : new ArrayList(getChildFolder()) ) {
child.markDeleted();
}
}
public void removeAllFromChildFolder(Set childFolder) {
for ( UserInteractionFolder o : childFolder ) {
removeFromChildFolder(o);
}
}
public void removeAllFromEntityUserInteraction(Set entityUserInteraction) {
for ( ClassifierUserInteraction o : entityUserInteraction ) {
removeFromEntityUserInteraction(o);
}
}
public void removeAllFromOperationUserInteraction(Set operationUserInteraction) {
for ( OperationUserInteraction o : operationUserInteraction ) {
removeFromOperationUserInteraction(o);
}
}
public void removeFromChildFolder(UserInteractionFolder childFolder) {
childFolder.setParentFolder(null);
}
public void removeFromEntityUserInteraction(ClassifierUserInteraction entityUserInteraction) {
entityUserInteraction.setFolder(null);
}
public void removeFromOperationUserInteraction(OperationUserInteraction operationUserInteraction) {
operationUserInteraction.setFolder(null);
}
public void removeFromOwningObject() {
this.markDeleted();
}
public void setChildFolder(Set childFolder) {
for ( UserInteractionFolder o : new HashSet(this.childFolder) ) {
o.setParentFolder(null);
}
for ( UserInteractionFolder o : childFolder ) {
o.setParentFolder((AbstractUserInteractionFolder)this);
}
}
public void setEntityUserInteraction(Set entityUserInteraction) {
for ( ClassifierUserInteraction o : new HashSet(this.entityUserInteraction) ) {
o.setFolder(null);
}
for ( ClassifierUserInteraction o : entityUserInteraction ) {
o.setFolder((AbstractUserInteractionFolder)this);
}
}
public void setOperationUserInteraction(Set operationUserInteraction) {
for ( OperationUserInteraction o : new HashSet(this.operationUserInteraction) ) {
o.setFolder(null);
}
for ( OperationUserInteraction o : operationUserInteraction ) {
o.setFolder((AbstractUserInteractionFolder)this);
}
}
public void shallowCopyState(AbstractUserInteractionFolder from, AbstractUserInteractionFolder to) {
to.setName(from.getName());
to.setAdditionalHumanName(from.getAdditionalHumanName());
to.setRepresentedElement(getRepresentedElement());
}
public String toString() {
StringBuilder sb = new StringBuilder();
if ( getOwner()==null ) {
sb.append("owner=null;");
} else {
sb.append("owner="+getOwner().getClass().getSimpleName()+"[");
sb.append(getOwner().getName());
sb.append("];");
}
sb.append("name=");
sb.append(getName());
sb.append(";");
sb.append("additionalHumanName=");
sb.append(getAdditionalHumanName());
sb.append(";");
sb.append("humanName=");
sb.append(getHumanName());
sb.append(";");
sb.append("qualifiedName=");
sb.append(getQualifiedName());
sb.append(";");
if ( getRepresentedElement()==null ) {
sb.append("representedElement=null;");
} else {
sb.append("representedElement="+getRepresentedElement().getClass().getSimpleName()+"[");
sb.append(getRepresentedElement().getName());
sb.append("];");
}
return sb.toString();
}
public String toXmlString() {
StringBuilder sb = new StringBuilder();
if ( getName()==null ) {
sb.append(" ");
} else {
sb.append("");
sb.append(getName());
sb.append(" ");
sb.append("\n");
}
if ( getAdditionalHumanName()==null ) {
sb.append(" ");
} else {
sb.append("");
sb.append(getAdditionalHumanName());
sb.append(" ");
sb.append("\n");
}
if ( getRepresentedElement()==null ) {
sb.append(" ");
} else {
sb.append("");
sb.append(getRepresentedElement().getClass().getSimpleName());
sb.append("[");
sb.append(getRepresentedElement().getName());
sb.append("]");
sb.append(" ");
sb.append("\n");
}
for ( OperationUserInteraction operationUserInteraction : getOperationUserInteraction() ) {
sb.append("");
sb.append(operationUserInteraction.toXmlString());
sb.append(" ");
sb.append("\n");
}
for ( ClassifierUserInteraction entityUserInteraction : getEntityUserInteraction() ) {
sb.append("");
sb.append(entityUserInteraction.toXmlString());
sb.append(" ");
sb.append("\n");
}
for ( UserInteractionFolder childFolder : getChildFolder() ) {
sb.append("");
sb.append(childFolder.toXmlString());
sb.append(" ");
sb.append("\n");
}
return sb.toString();
}
/** Implements Sequence{}
*/
private List collectionLiteral1() {
List myList = new ArrayList();
return myList;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy