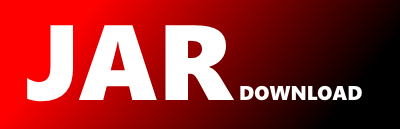
org.nasdanika.html.model.bootstrap.Appearance Maven / Gradle / Ivy
/**
*/
package org.nasdanika.html.model.bootstrap;
import org.eclipse.emf.common.util.EList;
import org.eclipse.emf.common.util.EMap;
import org.eclipse.emf.ecore.EObject;
import org.nasdanika.html.bootstrap.Color;
/**
*
* A representation of the model object 'Appearance'.
*
*
*
* This class is used for configuring common aspects of HTML and Bootstrap elements such as background, spacing, text, etc.
*
*
*
* The following features are supported:
*
*
* - {@link org.nasdanika.html.model.bootstrap.Appearance#getBackground Background}
* - {@link org.nasdanika.html.model.bootstrap.Appearance#getAttributes Attributes}
* - {@link org.nasdanika.html.model.bootstrap.Appearance#getBorder Border}
* - {@link org.nasdanika.html.model.bootstrap.Appearance#getMargin Margin}
* - {@link org.nasdanika.html.model.bootstrap.Appearance#getPadding Padding}
* - {@link org.nasdanika.html.model.bootstrap.Appearance#getText Text}
* - {@link org.nasdanika.html.model.bootstrap.Appearance#getFloat Float}
* - {@link org.nasdanika.html.model.bootstrap.Appearance#getChildren Children}
*
*
* @see org.nasdanika.html.model.bootstrap.BootstrapPackage#getAppearance()
* @model annotation="http://www.eclipse.org/emf/2002/Ecore constraints='border_overlap background attributes'"
* @generated
*/
public interface Appearance extends EObject {
/**
* Returns the value of the 'Background' attribute.
*
*
*
* Bootstrap color for background.
*
* @return the value of the 'Background' attribute.
* @see #setBackground(Color)
* @see org.nasdanika.html.model.bootstrap.BootstrapPackage#getAppearance_Background()
* @model dataType="org.nasdanika.html.model.bootstrap.Color"
* @generated
*/
Color getBackground();
/**
* Sets the value of the '{@link org.nasdanika.html.model.bootstrap.Appearance#getBackground Background}' attribute.
*
*
* @param value the new value of the 'Background' attribute.
* @see #getBackground()
* @generated
*/
void setBackground(Color value);
/**
* Returns the value of the 'Attributes' map.
* The key is of type {@link java.lang.String},
* and the value is of type {@link org.eclipse.emf.ecore.EObject},
*
*
*
* HTML element (tag) attributes. Attributes defined at the appearance level overwrite attributes defined at the HTML element level.
* It is recommended to use one of the other.
*
* ## Interpolation
*
* Attribute values are interpolated, i.e. tokens in the form of ``${token name[|default value]}`` are replaced with the contextual values or default values, if any. Examples:
*
* * ``${my-style}`` - Token without a default value.
* * ``${font-weight|bold}`` - Token with a default value.
*
* ## Regular attributes
*
* For all top-level entries except ``class``, ``style``, and ``data`` attribute value is produced by converting the value to string for scalars and to JSON string for lists and maps.
* For attributes which do not start with ``data-`` a warning is issued if the value is not a scalar, i.e. a list or a map.
*
* ## Class
*
* For class attribute its value is formed by concantenating elements using space as a separator. If elements are hierarchical then class name is formed by concatenation with a dash (``-``) as a separator.
*
* ## Data
*
* If value of ``data`` attbibute is a map then keys of that map get concatenated with ``data`` using dash (``-``) as a separator, them same applies to nested maps. Non-map values become attribute values - scalars are converted to string, lists are converted to JSON string.
*
* ## Style
*
* Style can be defined as a string, list or map. If style is defined as a list, all list values are concatenated with a space as a separator - it is a convent way for long unstructured definitions.
*
* If style value is a map then the value and its contained map values are processed in the following fashion:
*
* * Keys are concatenated with dash as a separator.
* * List values are contcatenated wtih space as a separator.
*
*
* @return the value of the 'Attributes' map.
* @see org.nasdanika.html.model.bootstrap.BootstrapPackage#getAppearance_Attributes()
* @model mapType="org.nasdanika.exec.Property<org.eclipse.emf.ecore.EString, org.eclipse.emf.ecore.EObject>"
* @generated
*/
EMap getAttributes();
/**
* Returns the value of the 'Border' containment reference list.
* The list contents are of type {@link org.nasdanika.html.model.bootstrap.Border}.
*
*
*
* Border configuration.
*
* @return the value of the 'Border' containment reference list.
* @see org.nasdanika.html.model.bootstrap.BootstrapPackage#getAppearance_Border()
* @model containment="true" upper="4"
* annotation="urn:org.nasdanika homogenous='true' strict-containment='true'"
* @generated
*/
EList getBorder();
/**
* Returns the value of the 'Margin' containment reference list.
* The list contents are of type {@link org.nasdanika.html.model.bootstrap.Spacing}.
*
*
*
* Margin configuration.
*
* @return the value of the 'Margin' containment reference list.
* @see org.nasdanika.html.model.bootstrap.BootstrapPackage#getAppearance_Margin()
* @model containment="true"
* annotation="urn:org.nasdanika homogenous='true' strict-containment='true'"
* @generated
*/
EList getMargin();
/**
* Returns the value of the 'Padding' containment reference list.
* The list contents are of type {@link org.nasdanika.html.model.bootstrap.Spacing}.
*
*
*
* Padding configuration.
*
* @return the value of the 'Padding' containment reference list.
* @see org.nasdanika.html.model.bootstrap.BootstrapPackage#getAppearance_Padding()
* @model containment="true"
* annotation="urn:org.nasdanika homogenous='true' strict-containment='true'"
* @generated
*/
EList getPadding();
/**
* Returns the value of the 'Text' containment reference.
*
*
*
* Text style
*
* @return the value of the 'Text' containment reference.
* @see #setText(Text)
* @see org.nasdanika.html.model.bootstrap.BootstrapPackage#getAppearance_Text()
* @model containment="true"
* annotation="urn:org.nasdanika homogenous='true' strict-containment='true'"
* @generated
*/
Text getText();
/**
* Sets the value of the '{@link org.nasdanika.html.model.bootstrap.Appearance#getText Text}' containment reference.
*
*
* @param value the new value of the 'Text' containment reference.
* @see #getText()
* @generated
*/
void setText(Text value);
/**
* Returns the value of the 'Float' containment reference list.
* The list contents are of type {@link org.nasdanika.html.model.bootstrap.Float}.
*
*
*
* Float configuration.
*
* @return the value of the 'Float' containment reference list.
* @see org.nasdanika.html.model.bootstrap.BootstrapPackage#getAppearance_Float()
* @model containment="true"
* annotation="urn:org.nasdanika homogenous='true' strict-containment='true' feature-key='float'"
* @generated
*/
EList getFloat();
/**
* Returns the value of the 'Children' map.
* The key is of type {@link java.lang.String},
* and the value is of type {@link org.nasdanika.html.model.bootstrap.Appearance},
*
*
* @return the value of the 'Children' map.
* @see org.nasdanika.html.model.bootstrap.BootstrapPackage#getAppearance_Children()
* @model mapType="org.nasdanika.html.model.bootstrap.AppearanceEntry<org.eclipse.emf.ecore.EString, org.nasdanika.html.model.bootstrap.Appearance>"
* @generated
*/
EMap getChildren();
/**
*
*
* @model
* @generated
*/
Appearance effectiveAppearance(String path);
} // Appearance
© 2015 - 2024 Weber Informatics LLC | Privacy Policy