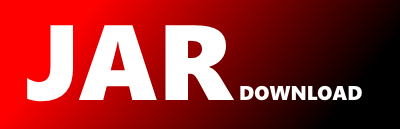
org.nbnResolving.database.impl.EpicurDaoImpl Maven / Gradle / Ivy
/* *********************************************************************
* Class EpicurDaoImpl
*
* Copyright (c) 2010-2013, German National Library/Deutsche Nationalbibliothek
* Adickesallee 1, D-60322 Frankfurt am Main, Federal Republic of Germany
*
* This program is free software. Licensed under the Apache License, Version 2.0
* (the "License"); you may not use this file except in compliance with the
* License. You may obtain a copy of the License at
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
* WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
* License for the specific language governing permissions and limitations under
* the License.
*
* Kadir Karaca Kocer -- German National Library
*
**********************************************************************/
/* ********************************************************************
* CHANGELOG:
*
* 2013-09-23 Refactoring due to JDBC-Pooling problems, Karaca
* 2013-02-26 More comments and Checkstyle improvements, Karaca
* 2012-03-15 Commented and ported to Apache Maven, Karaca
* 2012-10-01 getNamespaceByName() and getNamespaceIdsForInstitution() added, Karaca
* 2012-08-30 New function getUrlsFaultyByInstId() - M. Klein
* 2012-08-29 New functions getNumberOf...ByInstId() - M. Klein
* 2012-08-28 Functions getNumberOf...ByPersId() deleted - M. Klein
* 2012-07-16 Refactoring - M. Klein
* 2012-07-10 New functions getNumberOf...ByPersId() - M. Klein
* 2012-07-09 Adjusting the interface - M. Klein
* Created on 2011-08-25 by Kadir Karaca Kocer, German National Library
* ******************************************************************
*/
package org.nbnResolving.database.impl;
import java.sql.Connection;
import java.sql.SQLException;
import java.util.List;
import javax.sql.DataSource;
import org.apache.commons.logging.Log;
import org.apache.commons.logging.LogFactory;
import org.nbnResolving.database.DataAccessException;
import org.nbnResolving.database.EpicurDAO;
import org.nbnResolving.database.model.JoinNSPERMISSION;
import org.nbnResolving.database.model.TableINSTITUTION;
import org.nbnResolving.database.model.TableMIMETYPES;
import org.nbnResolving.database.model.TableNAMESPACE;
import org.nbnResolving.database.model.TableNS2PERMISSION;
import org.nbnResolving.database.model.TablePERMISSION;
import org.nbnResolving.database.model.TablePERSON;
import org.nbnResolving.database.model.TableURL;
import org.nbnResolving.database.model.TableURN;
/**
* Class that implements the Epicur Data Access Object Interface.
* This is original Sybase relational database model of
* the first generation URN:NBN system of German National Library.
*
* Because of this legacy production database, the new generation
* URN system must be backwards compatible.
* Please see the original relational data model
* to understand how this functions and why.
*
* @author Kadir Karaca Kocer, German National Library
*/
public class EpicurDaoImpl implements EpicurDAO {
private static final Log LOGGER = LogFactory.getLog(EpicurDaoImpl.class);
private static final boolean READ_ONLY = true;
private static final boolean WRITE_ACCESS = false;
/**
* Source where the data comes from. For this implementation a RDBMS.
* Works with Sybase, MySQL & HyperSQL. All other SQL-Standard Database system should also work.
*/
protected DataSource dataSource;
// getters & setters
/**
* @return DataSource
*/
public DataSource getDataSource() {
return this.dataSource;
}
/**
* @param ds The Data Source to set.
*/
public void setDataSource(final DataSource ds) {
this.dataSource = ds;
}
/**
* @param readOnly
* @return A connection to RDBMS.
* @throws DataAccessException
*/
public Connection getConnection(boolean readOnly) throws DataAccessException {
if (this.dataSource == null) {
throw new DataAccessException("ERROR: exception in getConnection(). DataSource is not correctly initialized!");
}
Connection conn = null;
try {
conn = this.dataSource.getConnection();
conn.setReadOnly(readOnly);
} catch (SQLException e) {
throw new DataAccessException("ERROR: exception in getConnection() " + e.getMessage() + "\nSQL State is: " + e.getSQLState());
}
return conn;
}
/**
* @param conn The Connection to close.
* @throws DataAccessException
*/
public static void closeConnection(final Connection conn) throws DataAccessException {
if (conn != null) {
try {
if (!conn.isClosed()) {
conn.close();
LOGGER.debug("Database connection successfully closed.");
} else {
LOGGER.warn("Can not close Connection. It is already closed. Skipping ...");
}
} catch (SQLException e) {
String message = "ERROR: exception in closeConnection() " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
}
} else {
LOGGER.warn("Can not close Connection. It is already NULL. Skipping ...");
}
}
/**
* {@inheritDoc}
*/
@Override
public TableINSTITUTION getInstitutionById(final int instId) throws DataAccessException {
TableINSTITUTION inst = null;
Connection conn = null;
try {
conn = getConnection(READ_ONLY);
inst = DatabaseUtils.getInstitution(conn, instId, true);
} catch (SQLException e) {
String message = "ERROR: exception in getInstitutionById(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.getInstitutionById() ended successfully.");}
return inst;
}
/**
* {@inheritDoc}
*/
@Override
public TableMIMETYPES getMimetypeById(final int mimetypeId) throws DataAccessException {
final TableMIMETYPES mime = new TableMIMETYPES();
Connection conn = null;
try {
conn = getConnection(READ_ONLY);
mime.setMimetype_id(mimetypeId);
mime.setMimetype(DatabaseUtils.getMimeType(conn, mimetypeId));
} catch (SQLException e) {
String message = "ERROR: exception in getMimetypeById(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.getMimetypeById() ended successfully.");}
return mime;
}
/**
* {@inheritDoc}
*/
@Override
public List getMimetypes() throws DataAccessException {
List mimetypes = null;
Connection conn = null;
try {
conn = getConnection(READ_ONLY);
mimetypes = DatabaseUtils.getMimeTypeList(conn);
} catch (SQLException e) {
String message = "ERROR: exception in getMimetypes(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.getMimetypes() ended successfully.");}
return mimetypes;
}
/**
* {@inheritDoc}
*/
@Override
public List getNs2PermissionsByPersId(final int personId) throws DataAccessException {
List n2p = null;
Connection conn = null;
try {
conn = getConnection(READ_ONLY);
n2p = DatabaseUtils.getNs2PermissionsByPersId(conn, personId);
} catch (SQLException e) {
String message = "ERROR: exception in getNs2PermissionsByPersId(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.getNs2PermissionsByPersId() ended successfully.");}
return n2p;
}
/**
* {@inheritDoc}
*/
@Override
public TableNAMESPACE getNamespaceById(final int nsId) throws DataAccessException {
TableNAMESPACE ns = null;
Connection conn = null;
try {
conn = getConnection(READ_ONLY);
ns = DatabaseUtils.getNamespace(conn, nsId);
} catch (SQLException e) {
String message = "ERROR: exception in getNamespaceById(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.getNamespaceById() ended successfully.");}
return ns;
}
/**
* {@inheritDoc}
*/
@Override
public TableNAMESPACE getNamespaceByName(final String name) throws DataAccessException {
TableNAMESPACE ns = null;
Connection conn = null;
try {
conn = getConnection(READ_ONLY);
ns = DatabaseUtils.getNamespace(conn, name);
} catch (SQLException e) {
String message = "ERROR: exception in getNamespaceByName(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.getNamespaceByName() ended successfully.");}
return ns;
}
/**
* {@inheritDoc}
*/
@Override
public List getNamespaceIdsForInstitution(final int instId) throws DataAccessException {
List list = null;
Connection conn = null;
try {
conn = getConnection(READ_ONLY);
list = DatabaseUtils.getNamespaceIdsForInstitution(conn, instId);
} catch (SQLException e) {
String message = "ERROR: exception in getNamespaceIdsForInstitution(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.getNamespaceIdsForInstitution() ended successfully.");}
return list;
}
/**
* {@inheritDoc}
*/
@Override
public TablePERMISSION getPermissionById(final int permissionId) throws DataAccessException {
TablePERMISSION permission = null;
Connection conn = null;
try {
conn = getConnection(READ_ONLY);
permission = DatabaseUtils.getPermissionById(conn, permissionId);
} catch (SQLException e) {
String message = "ERROR: exception in getPermissionById(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.getPermissionById() ended successfully.");}
return permission;
}
// **** PERSON ****************************************
/**
* {@inheritDoc}
*/
@Override
public TablePERSON getPersonByLogin(final String login) throws DataAccessException {
TablePERSON person = null;
Connection conn = null;
try {
conn = getConnection(READ_ONLY);
person = DatabaseUtils.getPersonByLogin(conn, login);
} catch (SQLException e) {
String message = "ERROR: exception in getPersonByLogin(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.getPersonByLogin() ended successfully.");}
return person;
}
/**
* {@inheritDoc}
*/
@Override
public TablePERSON getPersonById(final int persId) throws DataAccessException {
TablePERSON person = null;
Connection conn = null;
try {
conn = getConnection(READ_ONLY);
person = DatabaseUtils.getPersonById(conn, persId);
} catch (SQLException e) {
String message = "ERROR: exception in getPersonById(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.getPersonById() ended successfully.");}
return person;
}
// **** URL ******************************************
/**
* {@inheritDoc}
*/
@Override
public List getUrlsByUrnId(final long urnId) throws DataAccessException {
List urls = null;
Connection conn = null;
try {
conn = getConnection(READ_ONLY);
urls = DatabaseUtils.getURLsByURNid(conn, urnId, false);
} catch (SQLException e) {
String message = "ERROR: exception in getUrlsByUrnId(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.getUrlsByUrnId() ended successfully.");}
return urls;
}
/**
* {@inheritDoc}
*/
@Override
public List getUrlsFaultyByPersId(final int persId) throws DataAccessException {
List urls = null;
Connection conn = null;
try {
conn = getConnection(READ_ONLY);
urls = DatabaseUtils.getURLsByPersId(conn, persId, true);
} catch (SQLException e) {
String message = "ERROR: exception in getUrlsFaultyByPersId(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.getUrlsFaultyByPersId() ended successfully.");}
return urls;
}
/**
* {@inheritDoc}
*/
@Override
public List getUrlsFaultyByInstId(final int instId) throws DataAccessException {
List urls = null;
Connection conn = null;
try {
conn = getConnection(READ_ONLY);
urls = DatabaseUtils.getURLsByInstId(conn, instId, true);
} catch (SQLException e) {
String message = "ERROR: exception in getUrlsFaultyByInstId(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.getUrlsFaultyByInstId() ended successfully.");}
return urls;
}
/**
* {@inheritDoc}
*/
@Override
public List getNsPermissionsByPersId(final int persId) throws DataAccessException {
List nsPermissions = null;
Connection conn = null;
try {
conn = getConnection(READ_ONLY);
nsPermissions = DatabaseUtils.getNsPermissionsByPersId(conn, persId);
} catch (SQLException e) {
String message = "ERROR: exception in getNsPermissionsByPersId(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.getNsPermissionsByPersId() ended successfully.");}
return nsPermissions;
}
/**
* {@inheritDoc}
*/
@Override
public List getUrlsFaultyByUrnId(final long urnId) throws DataAccessException {
List urls = null;
Connection conn = null;
try {
conn = getConnection(READ_ONLY);
urls = DatabaseUtils.getURLsByURNid(conn, urnId, true);
} catch (SQLException e) {
String message = "ERROR: exception in getUrlsFaultyByUrnId(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.getUrlsFaultyByUrnId() ended successfully.");}
return urls;
}
/**
* {@inheritDoc}
*/
@Override
public int getNumberOfUrlsByUrnId(final long urnId) throws DataAccessException {
int count = -1;
Connection conn = null;
try {
conn = getConnection(READ_ONLY);
count = DatabaseUtils.getNumberOfURLsByUrnId(conn, urnId, false);
} catch (SQLException e) {
String message = "ERROR: exception in getNumberOfUrlsByUrnId(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.getNumberOfUrlsByUrnId() ended successfully.");}
return count;
}
/**
* {@inheritDoc}
*/
@Override
public int getNumberOfUrlsByUrl(final String urlSubStr) throws DataAccessException {
int count = -1;
Connection conn = null;
try {
conn = getConnection(READ_ONLY);
count = DatabaseUtils.getNumberOfURLsByUrl(conn, urlSubStr);
} catch (SQLException e) {
String message = "ERROR: exception in getNumberOfUrlsByUrl(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.getNumberOfUrlsByUrl() ended successfully.");}
return count;
}
/**
* {@inheritDoc}
*/
@Override
public int getNumberOfUrlsFaultyByUrnId(final long urnId) throws DataAccessException {
int count = -1;
Connection conn = null;
try {
conn = getConnection(READ_ONLY);
count = DatabaseUtils.getNumberOfURLsByUrnId(conn, urnId, true);
} catch (SQLException e) {
String message = "ERROR: exception in getNumberOfUrlsFaultyByUrnId(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.getNumberOfUrlsFaultyByUrnId() ended successfully.");}
return count;
}
/**
* {@inheritDoc}
*/
@Override
public List getUrlsByUrl(final String urlSubStr) throws DataAccessException {
List urls = null;
Connection conn = null;
try {
conn = getConnection(READ_ONLY);
urls = DatabaseUtils.getURLsStartingWith(conn, urlSubStr);
} catch (SQLException e) {
String message = "ERROR: exception in getUrlsByUrl(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.getUrlsByUrl() ended successfully.");}
return urls;
}
/**
* {@inheritDoc}
*/
@Override
public TableURL getUrlByUrl(final String urlStr) throws DataAccessException {
TableURL url = null;
Connection conn = null;
try {
conn = getConnection(READ_ONLY);
url = DatabaseUtils.getURL(conn, urlStr);
} catch (SQLException e) {
String message = "ERROR: exception in getUrlByUrl(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.getUrlByUrl() ended successfully.");}
return url;
}
/**
* {@inheritDoc}
*/
@Override
public void updateUrl(final TableURL url) throws DataAccessException {
Connection conn = null;
try {
conn = getConnection(WRITE_ACCESS);
AdminUtils.updateURL(url, conn);
} catch (SQLException e) {
String message = "ERROR: exception in updateUrl(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.updateUrl() ended successfully.");}
}
/**
* {@inheritDoc}
*/
@Override
public void updateUrl(final TableURL url, final String urlStr) throws DataAccessException {
Connection conn = null;
try {
conn = getConnection(WRITE_ACCESS);
AdminUtils.updateURL(url, urlStr, conn);
} catch (SQLException e) {
String message = "ERROR: exception in updateUrl(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.updateUrl() ended successfully.");}
}
/**
* {@inheritDoc}
*/
@Override
public void insertUrl(final TableURL url) throws DataAccessException {
Connection conn = null;
try {
conn = getConnection(WRITE_ACCESS);
AdminUtils.writeURLIntoDB(url, conn);
} catch (SQLException e) {
String message = "ERROR: exception in insertUrl(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.insertUrl() ended successfully.");}
}
/**
* {@inheritDoc}
*/
@Override
public void deleteUrl(final String urlStr) throws DataAccessException {
//Authorisation & Institution id check should happen at a level higher!
Connection conn = null;
try {
conn = getConnection(WRITE_ACCESS);
AdminUtils.deleteURL(urlStr, conn);
} catch (SQLException e) {
String message = "ERROR: exception in deleteUrl(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.deleteUrl() ended successfully.");}
}
// **** URN ******************************************
/**
* {@inheritDoc}
*/
@Override
public TableURN getUrnById(final long urnId) throws DataAccessException {
TableURN urn = null;
Connection conn = null;
try {
conn = getConnection(READ_ONLY);
urn = DatabaseUtils.getURNInfo(conn, urnId);
} catch (SQLException e) {
String message = "ERROR: exception in getUrnById(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.getUrnById() ended successfully.");}
return urn;
}
/**
* {@inheritDoc}
*/
@Override
public String getUrnAsStringById(final long urnId) throws DataAccessException {
String urn = null;
Connection conn = null;
try {
conn = getConnection(READ_ONLY);
urn = DatabaseUtils.getURNasString(conn, urnId);
} catch (SQLException e) {
String message = "ERROR: exception in getUrnAsStringById(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.getUrnAsStringById() ended successfully.");}
return urn;
}
/**
* {@inheritDoc}
*/
@Override
public List getUrnsByNsId(final int nsId) throws DataAccessException {
List urns = null;
Connection conn = null;
try {
conn = getConnection(READ_ONLY);
urns = DatabaseUtils.getURNsForNamespace(conn, nsId, DatabaseUtils.URN_ALL);
} catch (SQLException e) {
String message = "ERROR: exception in getUrnsByNsId(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.getUrnsByNsId() ended successfully.");}
return urns;
}
/**
* {@inheritDoc}
*/
@Override
public List getUrnsReservedByNsId(final int nsId) throws DataAccessException {
List urns = null;
Connection conn = null;
try {
conn = getConnection(READ_ONLY);
urns = DatabaseUtils.getURNsForNamespace(conn, nsId, DatabaseUtils.URN_RESERVED);
} catch (SQLException e) {
String message = "ERROR: exception in getUrnsReservedByNsId(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.getUrnsReservedByNsId() ended successfully.");}
return urns;
}
/**
* {@inheritDoc}
*/
@Override
public List getUrnsRegisteredByNsId(final int nsId) throws DataAccessException {
List urns = null;
Connection conn = null;
try {
conn = getConnection(READ_ONLY);
urns = DatabaseUtils.getURNsForNamespace(conn, nsId, DatabaseUtils.URN_ACTIVE);
} catch (SQLException e) {
String message = "ERROR: exception in getUrnsRegisteredByNsId(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.getUrnsRegisteredByNsId() ended successfully.");}
return urns;
}
/**
* {@inheritDoc}
*/
@Override
public List getUrnsByUrn(final String urnSubstr) throws DataAccessException {
List urns = null;
Connection conn = null;
try {
conn = getConnection(READ_ONLY);
urns = DatabaseUtils.getURNsStartingWith(conn, urnSubstr, DatabaseUtils.URN_ALL);
} catch (SQLException e) {
String message = "ERROR: exception in getUrnsByUrn(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.getUrnsByUrn() ended successfully.");}
return urns;
}
/**
* {@inheritDoc}
*/
@Override
public List getUrnsReservedByUrn(final String urnSubstr) throws DataAccessException {
List urns = null;
Connection conn = null;
try {
conn = getConnection(READ_ONLY);
urns = DatabaseUtils.getURNsStartingWith(conn, urnSubstr, DatabaseUtils.URN_RESERVED);
} catch (SQLException e) {
String message = "ERROR: exception in getUrnsReservedByUrn(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.getUrnsReservedByUrn() ended successfully.");}
return urns;
}
/**
* {@inheritDoc}
*/
@Override
public List getUrnsRegisteredByUrn(final String urnSubStr) throws DataAccessException {
List urns = null;
Connection conn = null;
try {
conn = getConnection(READ_ONLY);
urns = DatabaseUtils.getURNsStartingWith(conn, urnSubStr, DatabaseUtils.URN_ACTIVE);
} catch (SQLException e) {
String message = "ERROR: exception in getUrnsRegisteredByUrn(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.getUrnsRegisteredByUrn() ended successfully.");}
return urns;
}
/**
* {@inheritDoc}
*/
@Override
public TableURN getUrnByUrn(final String urnStr) throws DataAccessException {
TableURN urn = null;
Connection conn = null;
try {
conn = getConnection(READ_ONLY);
urn = DatabaseUtils.getURNInfo(conn, urnStr, false);
} catch (SQLException e) {
String message = "ERROR: exception in getUrnByUrn(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.getUrnByUrn() ended successfully.");}
return urn;
}
/**
* {@inheritDoc}
*/
@Override
public int getNumberOfUrnsByUrn(final String urnSubStr) throws DataAccessException {
int count = -1; // -1: not defined
Connection conn = null;
try {
conn = getConnection(READ_ONLY);
count = DatabaseUtils.getNumberOfURNs(conn, urnSubStr, DatabaseUtils.URN_ALL);
} catch (SQLException e) {
String message = "ERROR: exception in getNumberOfUrnsByUrn(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.getNumberOfUrnsByUrn() ended successfully.");}
return count;
}
/**
* {@inheritDoc}
*/
@Override
public int getNumberOfUrnsRegisteredByUrn(final String urnSubStr) throws DataAccessException {
int count = -1; // -1: not defined
Connection conn = null;
try {
conn = getConnection(READ_ONLY);
count = DatabaseUtils.getNumberOfURNs(conn, urnSubStr, DatabaseUtils.URN_ACTIVE);
} catch (SQLException e) {
String message = "ERROR: exception in getNumberOfUrnsRegisteredByUrn(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.getNumberOfUrnsRegisteredByUrn() ended successfully.");}
return count;
}
/**
* {@inheritDoc}
*/
@Override
public int getNumberOfUrnsReservedByUrn(final String urnSubStr) throws DataAccessException {
int count = -1; // -1: not defined
Connection conn = null;
try {
conn = getConnection(READ_ONLY);
count = DatabaseUtils.getNumberOfURNs(conn, urnSubStr, DatabaseUtils.URN_RESERVED);
} catch (SQLException e) {
String message = "ERROR: exception in getNumberOfUrnsReservedByUrn(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.getNumberOfUrnsReservedByUrn() ended successfully.");}
return count;
}
/**
* {@inheritDoc}
*/
@Override
public int getNumberOfUrnsReservedByNsId(final int nsId) throws DataAccessException {
int count = -1; // -1: not defined
Connection conn = null;
try {
conn = getConnection(READ_ONLY);
count = DatabaseUtils.getNumberOfURNs(conn, nsId, DatabaseUtils.URN_RESERVED);
} catch (SQLException e) {
String message = "ERROR: exception in getNumberOfUrnsReservedByNsId(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.getNumberOfUrnsReservedByNsId() ended successfully.");}
return count;
}
/**
* {@inheritDoc}
*/
@Override
public int getNumberOfUrnsRegisteredByInstId(final int instId) throws DataAccessException {
int count = -1; // -1: not defined
Connection conn = null;
try {
conn = getConnection(READ_ONLY);
count = DatabaseUtils.getNumberOfURNsByInstId(conn, instId, DatabaseUtils.URN_ACTIVE);
} catch (SQLException e) {
String message = "ERROR: exception in getNumberOfUrnsRegisteredByInstId(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.getNumberOfUrnsRegisteredByInstId() ended successfully.");}
return count;
}
/**
* {@inheritDoc}
*/
@Override
public int getNumberOfNamespacesByInstId(final int instId) throws DataAccessException {
int count = -1; // -1: not defined
Connection conn = null;
try {
conn = getConnection(READ_ONLY);
count = DatabaseUtils.getNumberOfNamespacesByInstId(conn, instId);
} catch (SQLException e) {
String message = "ERROR: exception in getNumberOfNamespacesByInstId(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.getNumberOfNamespacesByInstId() ended successfully.");}
return count;
}
/**
* {@inheritDoc}
*/
@Override
public int getNumberOfUrnsReservedByInstId(final int instId) throws DataAccessException {
int count = -1; // -1: not defined
Connection conn = null;
try {
conn = getConnection(READ_ONLY);
count = DatabaseUtils.getNumberOfURNsByInstId(conn, instId, DatabaseUtils.URN_RESERVED);
} catch (SQLException e) {
String message = "ERROR: exception in getNumberOfUrnsReservedByInstId(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.getNumberOfUrnsReservedByInstId() ended successfully.");}
return count;
}
/**
* {@inheritDoc}
*/
@Override
public int getNumberOfUrnsByInstId(final int instId) throws DataAccessException {
int count = -1; // -1: not defined
Connection conn = null;
try {
conn = getConnection(READ_ONLY);
count = DatabaseUtils.getNumberOfURNsByInstId(conn, instId);
} catch (SQLException e) {
String message = "ERROR: exception in getNumberOfUrnsByInstId(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.getNumberOfUrnsByInstId() ended successfully.");}
return count;
}
/**
* {@inheritDoc}
*/
@Override
public int getNumberOfUrlsByInstId(final int instId) throws DataAccessException {
int count = -1; // -1: not defined
Connection conn = null;
try {
conn = getConnection(READ_ONLY);
count = DatabaseUtils.getNumberOfURLsByInstId(conn, instId, false);
} catch (SQLException e) {
String message = "ERROR: exception in getNumberOfUrlsByInstId(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.getNumberOfUrlsByInstId() ended successfully.");}
return count;
}
/**
* {@inheritDoc}
*/
@Override
public int getNumberOfUrlsFaultyByInstId(final int instId) throws DataAccessException {
int count = -1; // -1: not defined
Connection conn = null;
try {
conn = getConnection(READ_ONLY);
count = DatabaseUtils.getNumberOfURLsByInstId(conn, instId, true);
} catch (SQLException e) {
String message = "ERROR: exception in getNumberOfUrlsFaultyByInstId(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.getNumberOfUrlsFaultyByInstId() ended successfully.");}
return count;
}
/**
* {@inheritDoc}
*/
@Override
public int getNumberOfUrnsRegisteredByNsId(final int nsId) throws DataAccessException {
int count = -1; // -1: not defined
Connection conn = null;
try {
conn = getConnection(READ_ONLY);
count = DatabaseUtils.getNumberOfURNs(conn, nsId, DatabaseUtils.URN_ACTIVE);
} catch (SQLException e) {
String message = "ERROR: exception in getNumberOfUrnsRegisteredByNsId(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.getNumberOfUrnsRegisteredByNsId() ended successfully.");}
return count;
}
/**
* {@inheritDoc}
*/
@Override
public void updateUrn(final TableURN urn) throws DataAccessException {
Connection conn = null;
try {
conn = getConnection(WRITE_ACCESS);
AdminUtils.updateURN(urn, conn);
} catch (SQLException e) {
String message = "ERROR: exception in updateUrn(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.updateUrn() ended successfully.");}
}
/**
* {@inheritDoc}
*/
@Override
public long insertUrn(final TableURN urn) throws DataAccessException {
long urnid = -1; // -1: not defined
Connection conn = null;
try {
conn = getConnection(WRITE_ACCESS);
urnid = AdminUtils.writeURNIntoDB(urn, conn);
} catch (SQLException e) {
String message = "ERROR: exception in insertUrn(): " + e.getMessage() + "\nSQL State is: " + e.getSQLState();
LOGGER.error(message);
throw new DataAccessException(message);
} finally {
closeConnection(conn); //close the database connection and free it -> see JDBC pool in Tomcat config
conn = null;
}
if (LOGGER.isDebugEnabled()) {LOGGER.debug("EpicurDaoImpl.insertUrn() ended successfully.");}
return urnid;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy